04-AOP
Eligible 英 [ˈelɪdʒəbl], 具备条件的
Ancestors 英 [ˈænsɪstəz], n. 祖宗;祖先;(动物的)原种;(机器的)原型
- 切点:哪些具体方法上进行切入,配置时指定通知的地方(怎么筛选这个具体的位置)
- 通知:在特定连接点上执行的动作(额外添加的业务逻辑)
- eg:
before(), after()
- eg:
- 连接点:能够被插入的切面的点(该位置来进行额外逻辑的执行)
- xml解析,处理后BD中包含什么信息
loadBeanDefinitions(default, custom)
- 对AOP相关的BD进行实例化操作
- 第一个对象创建之前,必须把AOP需要的相关对象提前准备好,因为无法预估哪些对象需要动态代理
1. MyAOP
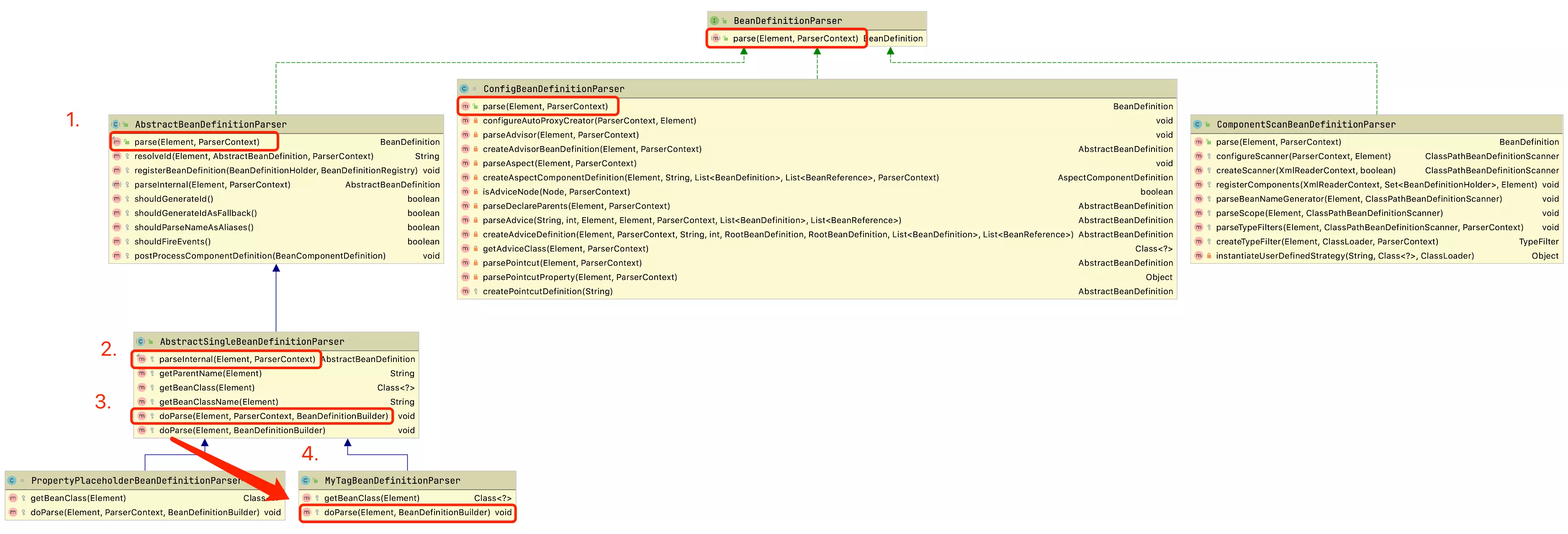
public class T20_1_Aop_xml {
public static void main(String[] args) throws Exception {
saveGeneratedCGlibProxyFiles(System.getProperty("user.dir") + "/proxy_spring");
ClassPathXmlApplicationContext ac = new ClassPathXmlApplicationContext("x20_aop.xml");
System.out.println("------------------- ac.over -------------------");
MyCalc myCalc = ac.getBean("myCalc", MyCalc.class);
myCalc.add(1, 1);
ac.close();
}
public static void saveGeneratedCGlibProxyFiles(String dir) throws Exception {
Field field = System.class.getDeclaredField("props");
field.setAccessible(true);
Properties props = (Properties) field.get(null);
// cglib.debugLocation => /Users/listao/mca_spring/proxy_spring
System.setProperty(DebuggingClassWriter.DEBUG_LOCATION_PROPERTY, dir); // dir为保存文件路径
props.put("net.sf.cglib.core.DebuggingClassWriter.traceEnabled", true);
}
}
<bean id="myCalc" class="com.listao.aop.xml.service.MyCalc"/>
<bean id="logUtil" class="com.listao.aop.xml.util.LogUtil"/>
<aop:config>
<aop:aspect ref="logUtil">
<aop:pointcut id="myPoint" expression="execution(Integer com.listao.aop.xml.service.MyCalc.*(..))"/>
<aop:around method="around" pointcut-ref="myPoint"/>
<aop:before method="before" pointcut-ref="myPoint"/>
<aop:after method="after" pointcut-ref="myPoint"/>
<aop:after-returning method="afterReturning" pointcut-ref="myPoint" returning="result"/>
<aop:after-throwing method="afterThrowing" pointcut-ref="myPoint" throwing="e"/>
</aop:aspect>
</aop:config>
<!--<aop:aspectj-autoproxy/>-->
public class MyCalc /*implements Calculator */ {
public Integer add(Integer i, Integer j) throws NoSuchMethodException {
System.out.println("====>>>> MyCalc.add()");
return i + j;
}
public Integer sub(Integer i, Integer j) throws NoSuchMethodException {
return i - j;
}
public Integer mul(Integer i, Integer j) throws NoSuchMethodException {
return i * j;
}
public Integer div(Integer i, Integer j) throws NoSuchMethodException {
return i / j;
}
}
public class LogUtil {
public int before(JoinPoint joinPoint) {
// 方法签名
Signature signature = joinPoint.getSignature();
// 入参信息
Object[] args = joinPoint.getArgs();
System.out.println("before() -> " + signature.getName() + "(), 参数为: " + Arrays.asList(args));
return 100;
}
public void afterReturning(JoinPoint joinPoint, Object result) {
Signature signature = joinPoint.getSignature();
System.out.println("afterReturning() -> " + signature.getName() + "(), 结果为: " + result);
}
public void afterThrowing(JoinPoint joinPoint, Exception e) {
Signature signature = joinPoint.getSignature();
System.out.println("afterThrowing() -> " + signature.getName() + "(), 异常: " + e.getMessage());
}
public void after(JoinPoint joinPoint) {
Signature signature = joinPoint.getSignature();
System.out.println("after() -> " + signature.getName() + "(), 结束");
}
/**
* ProceedingJoinPoint extends JoinPoint
* MethodInvocationProceedingJoinPoint implements ProceedingJoinPoint
*/
public Object around(ProceedingJoinPoint pjp) throws Throwable {
Signature signature = pjp.getSignature();
Object[] args = pjp.getArgs();
Object result;
try {
System.out.println("around() -> before, " + signature.getName() + "(), 参数为: " + Arrays.asList(args));
// 反射调用目标方法,相当于执行method.invoke(),可以自己修改结果值
result = pjp.proceed();
// args = new Object[]{1, 2};
// result = pjp.proceed(args); // 修改入参
System.out.println("around() -> after, " + signature.getName() + "()结果: " + result);
} catch (Throwable throwable) {
System.out.println("around() -> throwable, " + signature.getName() + "()异常");
throw throwable;
}
return result;
}
}
1. ConfigBeanDefinitionParser
AspectJAwareAdvisorAutoProxyCreator
=> IOC
class ConfigBeanDefinitionParser implements BeanDefinitionParser {
private static final String POINTCUT = "pointcut";
private static final String ADVISOR = "advisor";
private static final String ASPECT = "aspect";
public BeanDefinition parse(Element element, ParserContext parserContext) {
CompositeComponentDefinition compositeDef =
new CompositeComponentDefinition(element.getTagName(), parserContext.extractSource(element));
parserContext.pushContainingComponent(compositeDef);
// 1.. <aop:config> => AspectJAwareAdvisorAutoProxyCreator
configureAutoProxyCreator(parserContext, element);
// 解析<aop:config>节点
List<Element> childElts = DomUtils.getChildElements(element);
for (Element elt : childElts) {
String localName = parserContext.getDelegate().getLocalName(elt);
// <aop:pointcut>
if (POINTCUT.equals(localName)) {
parsePointcut(elt, parserContext);
}
// <aop:advice>
else if (ADVISOR.equals(localName)) {
parseAdvisor(elt, parserContext);
}
// 2.. <aop:aspect>
else if (ASPECT.equals(localName)) {
parseAspect(elt, parserContext);
}
}
parserContext.popAndRegisterContainingComponent();
return null;
}
private void configureAutoProxyCreator(ParserContext parserContext, Element element) {
// 1.
AopNamespaceUtils.registerAspectJAutoProxyCreatorIfNecessary(parserContext, element);
}
// ----------------------------------------------------------------------------------------
private void parseAspect(Element aspectElement, ParserContext parserContext) {
// id
String aspectId = aspectElement.getAttribute(ID);
// 1. ref,必须配置。代表切面`<aop:aspect ref="logUtil">`
String aspectName = aspectElement.getAttribute(REF);
try {
this.parseState.push(new AspectEntry(aspectId, aspectName));
List<BeanDefinition> beanDefinitions = new ArrayList<>();
List<BeanReference> beanReferences = new ArrayList<>();
// 解析<aop:aspect>的declare-parents => DeclareParentsAdvisor. declare-parents
List<Element> declareParents = DomUtils.getChildElementsByTagName(aspectElement, DECLARE_PARENTS);
for (int i = METHOD_INDEX; i < declareParents.size(); i++) {
Element declareParentsElement = declareParents.get(i);
beanDefinitions.add(parseDeclareParents(declareParentsElement, parserContext));
}
// We have to parse "advice" and all the advice kinds in one loop, to get the
// ordering semantics right.
NodeList nodeList = aspectElement.getChildNodes();
boolean adviceFoundAlready = false;
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
// 2.. (around|before|after|after-returning|after-throwing)进入,<aop:pointcut>跳过
if (isAdviceNode(node, parserContext)) {
if (!adviceFoundAlready) {
adviceFoundAlready = true;
if (!StringUtils.hasText(aspectName)) {
parserContext.getReaderContext().error(
"<aspect> tag needs aspect bean reference via 'ref' attribute when declaring advices.",
aspectElement, this.parseState.snapshot());
return;
}
beanReferences.add(new RuntimeBeanReference(aspectName));
}
// 3.. 解析advice并注册到IOC
AbstractBeanDefinition advisorDefinition = parseAdvice(
aspectName, i, aspectElement, (Element) node, parserContext, beanDefinitions, beanReferences);
beanDefinitions.add(advisorDefinition);
}
}
AspectComponentDefinition aspectComponentDefinition = createAspectComponentDefinition(
aspectElement, aspectId, beanDefinitions, beanReferences, parserContext);
parserContext.pushContainingComponent(aspectComponentDefinition);
// pointcut
List<Element> pointcuts = DomUtils.getChildElementsByTagName(aspectElement, POINTCUT);
for (Element pointcutElement : pointcuts) {
// 4.. <aop:pointcut>
parsePointcut(pointcutElement, parserContext);
}
parserContext.popAndRegisterContainingComponent();
} finally {
this.parseState.pop();
}
}
/**
* Return {@code true} if the supplied node describes an advice type. May be one of:
* '{@code before}', '{@code after}', '{@code after-returning}',
* '{@code after-throwing}' or '{@code around}'.
*/
private boolean isAdviceNode(Node aNode, ParserContext parserContext) {
if (!(aNode instanceof Element)) {
return false;
} else {
String name = parserContext.getDelegate().getLocalName(aNode);
// 1.
return (BEFORE.equals(name) || AFTER.equals(name) || AFTER_RETURNING_ELEMENT.equals(name) ||
AFTER_THROWING_ELEMENT.equals(name) || AROUND.equals(name));
}
}
// ------------------------------------------------------------------------------------------
private AbstractBeanDefinition parseAdvice(
String aspectName, int order, Element aspectElement, Element adviceElement, ParserContext parserContext,
List<BeanDefinition> beanDefinitions, List<BeanReference> beanReferences) {
try {
this.parseState.push(new AdviceEntry(parserContext.getDelegate().getLocalName(adviceElement)));
// create the method factory bean.
// 1. MethodLocatingFactoryBean => `<aop:around method="around" pointcut-ref="myPoint"/>`
RootBeanDefinition methodDefinition = new RootBeanDefinition(MethodLocatingFactoryBean.class);
methodDefinition.getPropertyValues().add("targetBeanName", aspectName); // logUtil
methodDefinition.getPropertyValues().add("methodName", adviceElement.getAttribute("method")); // around
methodDefinition.setSynthetic(true);
// create instance factory definition. SimpleBeanFactoryAwareAspectInstanceFactory对象
// 2. SimpleBeanFactoryAwareAspectInstanceFactory implements BeanFactoryAware包含BF
RootBeanDefinition aspectFactoryDef =
new RootBeanDefinition(SimpleBeanFactoryAwareAspectInstanceFactory.class);
aspectFactoryDef.getPropertyValues().add("aspectBeanName", aspectName); // logUtil
aspectFactoryDef.setSynthetic(true);
// register the pointcut.
// 3.. 包装AspectJAroundAdvice
AbstractBeanDefinition adviceDef = createAdviceDefinition(
adviceElement, parserContext, aspectName, order, methodDefinition, aspectFactoryDef,
beanDefinitions, beanReferences);
// configure the advisor.
// 4.1. 最终包装 AspectJPointcutAdvisor => AspectJAroundAdvice(AbstractAspectJAdvice)
RootBeanDefinition advisorDefinition = new RootBeanDefinition(AspectJPointcutAdvisor.class);
advisorDefinition.setSource(parserContext.extractSource(adviceElement));
// 4.2.
advisorDefinition.getConstructorArgumentValues().addGenericArgumentValue(adviceDef);
if (aspectElement.hasAttribute(ORDER_PROPERTY)) { // order
advisorDefinition.getPropertyValues().add(
ORDER_PROPERTY, aspectElement.getAttribute(ORDER_PROPERTY));
}
// 5. register the final advisor
parserContext.getReaderContext().registerWithGeneratedName(advisorDefinition);
return advisorDefinition;
} finally {
this.parseState.pop();
}
}
private AbstractBeanDefinition createAdviceDefinition(
Element adviceElement, ParserContext parserContext, String aspectName, int order,
RootBeanDefinition methodDef, RootBeanDefinition aspectFactoryDef,
List<BeanDefinition> beanDefinitions, List<BeanReference> beanReferences) {
// 1.. AdviceType
// `AspectJAroundAdvice|AspectJMethodBeforeAdvice|AspectJAfterAdvice|AspectJAfterReturningAdvice|AspectJAfterThrowingAdvice`
RootBeanDefinition adviceDefinition = new RootBeanDefinition(getAdviceClass(adviceElement, parserContext));
adviceDefinition.setSource(parserContext.extractSource(adviceElement));
// aspectName属性、declarationOrder属性
adviceDefinition.getPropertyValues().add(ASPECT_NAME_PROPERTY, aspectName); // aspectName, logUtil
adviceDefinition.getPropertyValues().add(DECLARATION_ORDER_PROPERTY, order); // declarationOrder, 3
// 节点是否含有`returning|throwing|arg-names`
if (adviceElement.hasAttribute(RETURNING)) {
adviceDefinition.getPropertyValues().add(
RETURNING_PROPERTY, adviceElement.getAttribute(RETURNING));
}
if (adviceElement.hasAttribute(THROWING)) {
adviceDefinition.getPropertyValues().add(
THROWING_PROPERTY, adviceElement.getAttribute(THROWING));
}
if (adviceElement.hasAttribute(ARG_NAMES)) {
adviceDefinition.getPropertyValues().add(
ARG_NAMES_PROPERTY, adviceElement.getAttribute(ARG_NAMES));
}
// 2. 有参构造函数填充
// AspectJAroundAdvice(MethodLocatingFactoryBean, AspectJExpressionPointcut, SimpleBeanFactoryAwareAspectInstanceFactory)
ConstructorArgumentValues cav = adviceDefinition.getConstructorArgumentValues();
// 2.1.
cav.addIndexedArgumentValue(METHOD_INDEX, methodDef); // index = 0
// <aop:around pointcut-ref="myPoint"/>
Object pointcut = parsePointcutProperty(adviceElement, parserContext);
if (pointcut instanceof BeanDefinition) {
cav.addIndexedArgumentValue(POINTCUT_INDEX, pointcut);
beanDefinitions.add((BeanDefinition) pointcut);
} else if (pointcut instanceof String) {
RuntimeBeanReference pointcutRef = new RuntimeBeanReference((String) pointcut);
// 2.2.
cav.addIndexedArgumentValue(POINTCUT_INDEX, pointcutRef); // index = 1
beanReferences.add(pointcutRef);
}
// 2.3.
cav.addIndexedArgumentValue(ASPECT_INSTANCE_FACTORY_INDEX, aspectFactoryDef); // index = 2
return adviceDefinition;
}
private Class<?> getAdviceClass(Element adviceElement, ParserContext parserContext) {
String elementName = parserContext.getDelegate().getLocalName(adviceElement);
if (BEFORE.equals(elementName)) {
return AspectJMethodBeforeAdvice.class;
} else if (AFTER.equals(elementName)) {
return AspectJAfterAdvice.class;
} else if (AFTER_RETURNING_ELEMENT.equals(elementName)) {
return AspectJAfterReturningAdvice.class;
} else if (AFTER_THROWING_ELEMENT.equals(elementName)) {
return AspectJAfterThrowingAdvice.class;
} else if (AROUND.equals(elementName)) {
// 1.2. around
return AspectJAroundAdvice.class;
} else {
throw new IllegalArgumentException("Unknown advice kind [" + elementName + "].");
}
}
@Nullable
private Object parsePointcutProperty(Element element, ParserContext parserContext) {
if (element.hasAttribute(POINTCUT) && element.hasAttribute(POINTCUT_REF)) {
parserContext.getReaderContext().error(
"Cannot define both 'pointcut' and 'pointcut-ref' on <advisor> tag.",
element, this.parseState.snapshot());
return null;
}
// pointcut
else if (element.hasAttribute(POINTCUT)) {
// Create a pointcut for the anonymous pc and register it.
String expression = element.getAttribute(POINTCUT);
AbstractBeanDefinition pointcutDefinition = createPointcutDefinition(expression);
pointcutDefinition.setSource(parserContext.extractSource(element));
return pointcutDefinition;
}
// pointcut-ref
else if (element.hasAttribute(POINTCUT_REF)) {
String pointcutRef = element.getAttribute(POINTCUT_REF);
if (!StringUtils.hasText(pointcutRef)) {
parserContext.getReaderContext().error(
"'pointcut-ref' attribute contains empty value.", element, this.parseState.snapshot());
return null;
}
return pointcutRef;
} else {
parserContext.getReaderContext().error(
"Must define one of 'pointcut' or 'pointcut-ref' on <advisor> tag.",
element, this.parseState.snapshot());
return null;
}
}
// --------------------------------------------------------------------------------------
private AbstractBeanDefinition parsePointcut(Element pointcutElement, ParserContext parserContext) {
// 1. id
String id = pointcutElement.getAttribute(ID);
// 2. expression
String expression = pointcutElement.getAttribute(EXPRESSION);
AbstractBeanDefinition pointcutDefinition = null;
try {
// 栈保存切入点
this.parseState.push(new PointcutEntry(id));
// 3. AspectJExpressionPointcut => prototype。并设置属性expression
pointcutDefinition = createPointcutDefinition(expression);
pointcutDefinition.setSource(parserContext.extractSource(pointcutElement));
String pointcutBeanName = id;
if (StringUtils.hasText(pointcutBeanName)) {
// 4. 注册bean
parserContext.getRegistry().registerBeanDefinition(pointcutBeanName, pointcutDefinition);
} else {
pointcutBeanName = parserContext.getReaderContext().registerWithGeneratedName(pointcutDefinition);
}
parserContext.registerComponent(
new PointcutComponentDefinition(pointcutBeanName, pointcutDefinition, expression));
} finally {
// 创建后移除
this.parseState.pop();
}
return pointcutDefinition;
}
protected AbstractBeanDefinition createPointcutDefinition(String expression) {
// 1. AspectJExpressionPointcut
RootBeanDefinition beanDefinition = new RootBeanDefinition(AspectJExpressionPointcut.class);
// 2. prototype
beanDefinition.setScope(BeanDefinition.SCOPE_PROTOTYPE);
beanDefinition.setSynthetic(true);
// 3. expression
beanDefinition.getPropertyValues().add(EXPRESSION, expression);
return beanDefinition;
}
}
1. AspectJPointcutAdvisor
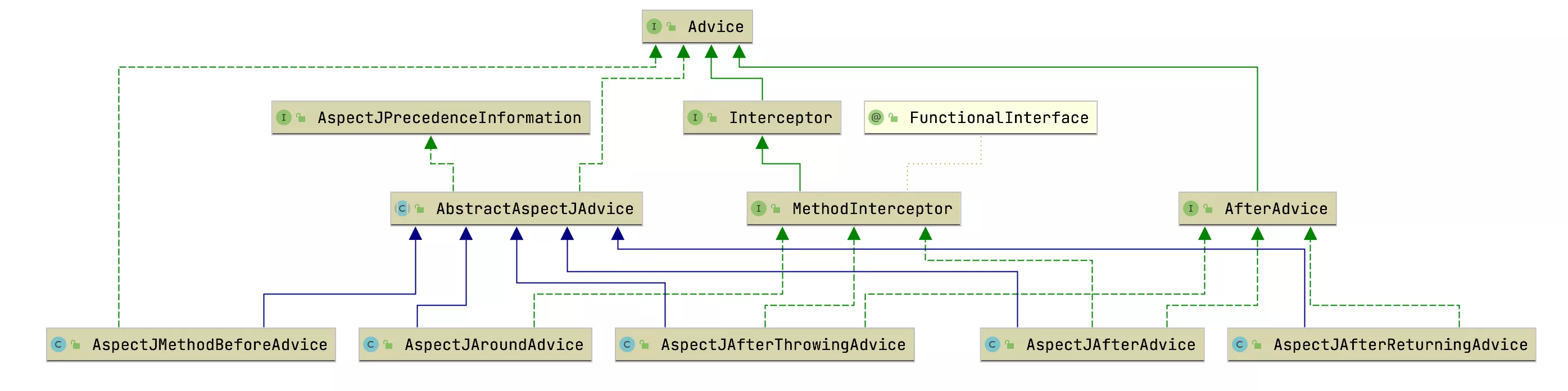
- AspectJPointcutAdvisor#0 => AspectJAroundAdvice(AbstractAspectJAdvice)
- MethodLocatingFactoryBean
- (targetBeanName = logUtil, methodName = around)
- AspectJExpressionPointcut
RuntimeBeanReference pointcutRef = new RuntimeBeanReference((String) pointcut);
(myPoint)
- SimpleBeanFactoryAwareAspectInstanceFactory
- (aspectBeanName = logUtil)
- MethodLocatingFactoryBean
- AspectJPointcutAdvisor#1 => AspectJAfterThrowingAdvice
- AspectJPointcutAdvisor#2 => AspectJAfterReturningAdvice
- AspectJPointcutAdvisor#3 => AspectJAfterAdvice
- AspectJPointcutAdvisor#4 => AspectJMethodBeforeAdvice
- 有参构造函数填充。AspectJAroundAdvice => AspectJPointcutAdvisor
public class AspectJPointcutAdvisor implements PointcutAdvisor, Ordered {
private final AbstractAspectJAdvice advice;
private final Pointcut pointcut;
@Nullable
private Integer order;
public AspectJPointcutAdvisor(AbstractAspectJAdvice advice) {
Assert.notNull(advice, "Advice must not be null");
this.advice = advice;
this.pointcut = advice.buildSafePointcut();
}
}
2. AspectJAroundAdvice
public class AspectJAroundAdvice extends AbstractAspectJAdvice implements MethodInterceptor, Serializable {
// 有参构造,需要提前准备三个类 <aop:around method="around" pointcut-ref="myPoint"/>
public AspectJAroundAdvice(
Method aspectJAroundAdviceMethod, // 1. around对象 => MethodLocatingFactoryBean
AspectJExpressionPointcut pointcut, // 2. myPoint => AspectJExpressionPointcut
AspectInstanceFactory aif // 3. BF => SimpleBeanFactoryAwareAspectInstanceFactory
) {
super(aspectJAroundAdviceMethod, pointcut, aif);
}
}
public abstract class AbstractAspectJAdvice implements Advice, AspectJPrecedenceInformation, Serializable {
// 99. ClassFilter, MethodMatcher
public final Pointcut buildSafePointcut() {
Pointcut pc = getPointcut();
MethodMatcher safeMethodMatcher = MethodMatchers.intersection(
new AdviceExcludingMethodMatcher(this.aspectJAdviceMethod), pc.getMethodMatcher());
return new ComposablePointcut(pc.getClassFilter(), safeMethodMatcher);
}
}
3. registerWithGeneratedName()
AspectJPointcutAdvisor
=> BF
// 5. register the final advisor
parserContext.getReaderContext().registerWithGeneratedName(advisorDefinition);
public class XmlReaderContext extends ReaderContext {
private final XmlBeanDefinitionReader reader;
public String registerWithGeneratedName(BeanDefinition beanDefinition) {
// org.springframework.aop.aspectj.AspectJPointcutAdvisor#0
String generatedName = generateBeanName(beanDefinition);
getRegistry().registerBeanDefinition(generatedName, beanDefinition);
return generatedName;
}
public String generateBeanName(BeanDefinition beanDefinition) {
return this.reader.getBeanNameGenerator().generateBeanName(beanDefinition, getRegistry());
}
}
4. AspectJExpressionPointcut
parsePointcut()
AspectJExpressionPointcut
- prototype

2. internalAutoProxyCreator
- xml是底层支撑,annotation是上层应用
- internal类加载。
AnnotationConfigUtils
(IOC)=>AopConfigUtils
(AOP)
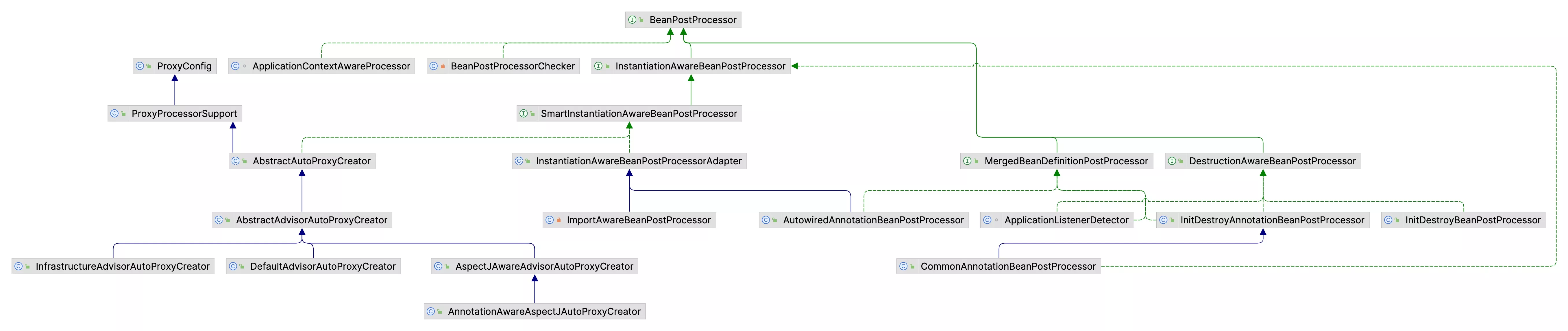
http\://www.springframework.org/schema/aop=org.springframework.aop.config.AopNamespaceHandler
public class AopNamespaceHandler extends NamespaceHandlerSupport {
@Override
public void init() {
// 1. <aop:config>
registerBeanDefinitionParser("config", new ConfigBeanDefinitionParser());
// 2. <aop:aspectj-autoproxy/> 注解解析器
registerBeanDefinitionParser("aspectj-autoproxy", new AspectJAutoProxyBeanDefinitionParser());
registerBeanDefinitionDecorator("scoped-proxy", new ScopedProxyBeanDefinitionDecorator());
registerBeanDefinitionParser("spring-configured", new SpringConfiguredBeanDefinitionParser());
}
}
1. AopNamespaceUtils
public abstract class AopNamespaceUtils {
// 1. <aop:config>
public static void registerAspectJAutoProxyCreatorIfNecessary(
ParserContext parserContext, Element sourceElement) {
// 1.1... => AspectJAwareAdvisorAutoProxyCreator
BeanDefinition beanDefinition = AopConfigUtils.registerAspectJAutoProxyCreatorIfNecessary(
parserContext.getRegistry(), parserContext.extractSource(sourceElement));
// proxy-target-class, expose-proxy属性处理
useClassProxyingIfNecessary(parserContext.getRegistry(), sourceElement);
registerComponentIfNecessary(beanDefinition, parserContext);
}
// 2. <aop:aspectj-autoproxy/>
public static void registerAspectJAnnotationAutoProxyCreatorIfNecessary(
ParserContext parserContext, Element sourceElement) {
// 2.1... => AnnotationAwareAspectJAutoProxyCreator
BeanDefinition beanDefinition = AopConfigUtils.registerAspectJAnnotationAutoProxyCreatorIfNecessary(
parserContext.getRegistry(), parserContext.extractSource(sourceElement));
// proxy-target-class, expose-proxy属性处理
useClassProxyingIfNecessary(parserContext.getRegistry(), sourceElement);
registerComponentIfNecessary(beanDefinition, parserContext);
}
}
1. <aop:config>
ConfigBeanDefinitionParser
=>AspectJAwareAdvisorAutoProxyCreator
class ConfigBeanDefinitionParser implements BeanDefinitionParser {
public BeanDefinition parse(Element element, ParserContext parserContext) {
// 1.. <aop:config> => AspectJAwareAdvisorAutoProxyCreator
configureAutoProxyCreator(parserContext, element);
// ...
}
private void configureAutoProxyCreator(ParserContext parserContext, Element element) {
// 1... <aop:config>
AopNamespaceUtils.registerAspectJAutoProxyCreatorIfNecessary(parserContext, element);
}
}
2. <aop:aspectj-autoproxy/>
AspectJAutoProxyBeanDefinitionParser
=>AnnotationAwareAspectJAutoProxyCreator
class AspectJAutoProxyBeanDefinitionParser implements BeanDefinitionParser {
// <aop:aspectj-autoproxy/>
public BeanDefinition parse(Element element, ParserContext parserContext) {
// 1... => AnnotationAwareAspectJAutoProxyCreator
AopNamespaceUtils.registerAspectJAnnotationAutoProxyCreatorIfNecessary(parserContext, element);
extendBeanDefinition(element, parserContext);
return null;
}
}
2. AopConfigUtils
public abstract class AopConfigUtils {
public static final String AUTO_PROXY_CREATOR_BEAN_NAME =
"org.springframework.aop.config.internalAutoProxyCreator";
static {
// Set up the escalation list...
APC_PRIORITY_LIST.add(InfrastructureAdvisorAutoProxyCreator.class);
APC_PRIORITY_LIST.add(AspectJAwareAdvisorAutoProxyCreator.class);
APC_PRIORITY_LIST.add(AnnotationAwareAspectJAutoProxyCreator.class);
}
// 1.. <aop:config> => AspectJAwareAdvisorAutoProxyCreator.class
public static BeanDefinition registerAspectJAutoProxyCreatorIfNecessary(
BeanDefinitionRegistry registry, @Nullable Object source) {
return registerOrEscalateApcAsRequired(AspectJAwareAdvisorAutoProxyCreator.class, registry, source);
}
// 2.. <aop:aspectj-autoproxy/>, @EnableAspectJAutoProxy => AnnotationAwareAspectJAutoProxyCreator.class
public static BeanDefinition registerAspectJAnnotationAutoProxyCreatorIfNecessary(
BeanDefinitionRegistry registry, @Nullable Object source) {
return registerOrEscalateApcAsRequired(AnnotationAwareAspectJAutoProxyCreator.class, registry, source);
}
// 3.. @EnableAspectJAutoProxy => 2.
public static BeanDefinition registerAspectJAnnotationAutoProxyCreatorIfNecessary(BeanDefinitionRegistry registry) {
return registerAspectJAnnotationAutoProxyCreatorIfNecessary(registry, null);
}
public static BeanDefinition registerAutoProxyCreatorIfNecessary(BeanDefinitionRegistry registry) {
// 4.1..
return registerAutoProxyCreatorIfNecessary(registry, null);
}
public static BeanDefinition registerAutoProxyCreatorIfNecessary(
BeanDefinitionRegistry registry, @Nullable Object source) {
// 4.2.. @EnableTransactionManagement => InfrastructureAdvisorAutoProxyCreator.class
return registerOrEscalateApcAsRequired(InfrastructureAdvisorAutoProxyCreator.class, registry, source);
}
// 最终 internalAutoProxyCreator => IOC
private static BeanDefinition registerOrEscalateApcAsRequired(
Class<?> cls, BeanDefinitionRegistry registry, @Nullable Object source) {
// 已经存在AutoProxyCreator且与现在不一致,根据优先级来判断使用哪个
if (registry.containsBeanDefinition(AUTO_PROXY_CREATOR_BEAN_NAME)) { // internalAutoProxyCreator
BeanDefinition apcDefinition = registry.getBeanDefinition(AUTO_PROXY_CREATOR_BEAN_NAME);
if (!cls.getName().equals(apcDefinition.getBeanClassName())) {
int currentPriority = findPriorityForClass(apcDefinition.getBeanClassName());
int requiredPriority = findPriorityForClass(cls);
if (currentPriority < requiredPriority) {
// 改变bean的className属性
apcDefinition.setBeanClassName(cls.getName());
}
}
// 一致,无须再次创建
return null;
}
// 1.
RootBeanDefinition beanDefinition = new RootBeanDefinition(cls);
beanDefinition.setSource(source);
beanDefinition.getPropertyValues().add("order", Ordered.HIGHEST_PRECEDENCE);
beanDefinition.setRole(BeanDefinition.ROLE_INFRASTRUCTURE);
// 99. internalAutoProxyCreator
registry.registerBeanDefinition(AUTO_PROXY_CREATOR_BEAN_NAME, beanDefinition);
return beanDefinition;
}
}
1. @EnableAspectJAutoProxy
public class T20_2_Aop_Anno {
public static void main(String[] args) throws NoSuchMethodException {
AnnotationConfigApplicationContext ac = new AnnotationConfigApplicationContext();
System.out.println("------------------- ac.over -------------------");
// 1...
ac.register(AopConfig.class);
ac.refresh();
MyCalc myCalc = ac.getBean("myCalc", MyCalc.class);
myCalc.add(1, 1);
ac.close();
}
}
@Configuration
@ComponentScan(basePackages = "com.listao.aop.annotation")
// 1...
@EnableAspectJAutoProxy
public class AopConfig {
}
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
// 1...
@Import(AspectJAutoProxyRegistrar.class)
public @interface EnableAspectJAutoProxy {}
class AspectJAutoProxyRegistrar implements ImportBeanDefinitionRegistrar {
@Override
public void registerBeanDefinitions(
AnnotationMetadata importingClassMetadata, BeanDefinitionRegistry registry) {
// 1... => AnnotationAwareAspectJAutoProxyCreator
AopConfigUtils.registerAspectJAnnotationAutoProxyCreatorIfNecessary(registry);
// ...
}
}
2. @EnableTransactionManagement
public class T21_2_Tx_Anno {
public static void main(String[] args) {
AnnotationConfigApplicationContext ac = new AnnotationConfigApplicationContext();
System.out.println("------------------- ac.over -------------------");
// 1... 注册IOC
ac.register(TxConfig.class);
ac.refresh();
// BookService bean = applicationContext.getBean(BookService.class);
// bean.checkout("zhangsan", 1);
TxDao txDao = ac.getBean(TxDao.class);
txDao.updDao(1);
ac.close();
}
}
// 1...
@EnableTransactionManagement
public class TxConfig {}
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
// 1...
@Import(TransactionManagementConfigurationSelector.class)
public @interface EnableTransactionManagement {}
public class TransactionManagementConfigurationSelector extends AdviceModeImportSelector<EnableTransactionManagement> {
@Override
protected String[] selectImports(AdviceMode adviceMode) {
switch (adviceMode) {
case PROXY:
// 1. org.springframework.context.annotation.AutoProxyRegistrar
// org.springframework.transaction.annotation.ProxyTransactionManagementConfiguration
return new String[]{AutoProxyRegistrar.class.getName(),
ProxyTransactionManagementConfiguration.class.getName()};
case ASPECTJ:
return new String[]{determineTransactionAspectClass()};
default:
return null;
}
}
}
public enum AdviceMode {
/**
* JDK proxy-based advice.
*/
PROXY,
/**
* AspectJ weaving-based advice.
*/
ASPECTJ
}
public class AutoProxyRegistrar implements ImportBeanDefinitionRegistrar {
@Override
public void registerBeanDefinitions(AnnotationMetadata importingClassMetadata, BeanDefinitionRegistry registry) {
// ...
if (mode == AdviceMode.PROXY) {
// 1... InfrastructureAdvisorAutoProxyCreator
AopConfigUtils.registerAutoProxyCreatorIfNecessary(registry);
}
}
}
2. applyBPPBeforeInstantiation()
registerBeanPostProcessors()
,已经将AspectJAwareAdvisorAutoProxyCreator
实例化
- 传统实例化
- 无参构造,
setter()
设置属性值
- 无参构造,
- AOP实例化(AspectJPointcutAdvisor#0)
- 有参构造,把实参对象提前创建好,包含无数个嵌套环节
InfrastructureAdvisorAutoProxyCreator, AspectJAwareAdvisorAutoProxyCreator, AnnotationAwareAspectJAutoProxyCreator
父类
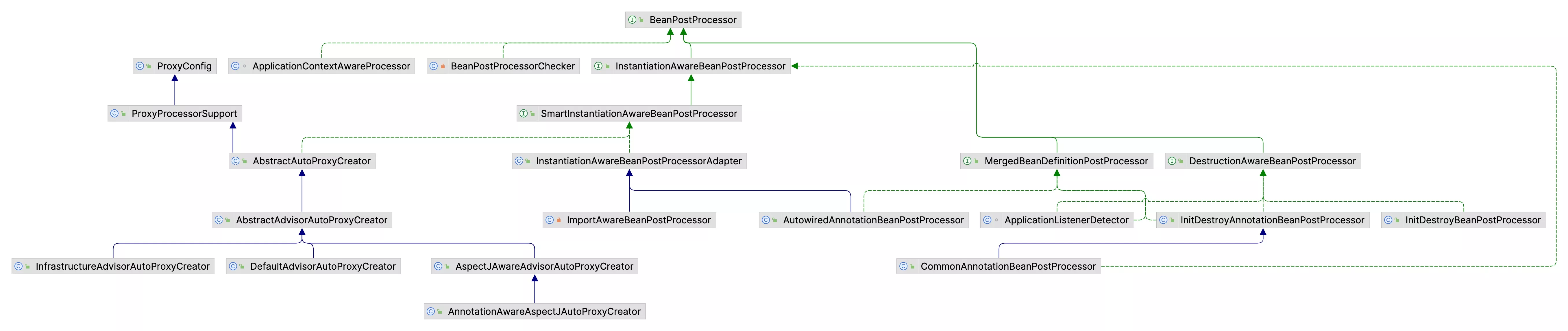
1. AbstractAutoProxyCreator
AspectJAwareAdvisorAutoProxyCreator.shouldSkip()
AbstractAutoProxyCreator#postProcessBeforeInstantiation()
public abstract class AbstractAutoProxyCreator extends ProxyProcessorSupport
implements SmartInstantiationAwareBeanPostProcessor, BeanFactoryAware {
public Object postProcessBeforeInstantiation(Class<?> beanClass, String beanName) {
Object cacheKey = getCacheKey(beanClass, beanName);
if (!StringUtils.hasLength(beanName) || !this.targetSourcedBeans.contains(beanName)) {
// 已处理
if (this.advisedBeans.containsKey(cacheKey)) {
return null;
}
/**
* 1.. isInfrastructureClass() => Advice, Pointcut, Advisor, AopInfrastructureBean
* 任何一个非基础对象,都进行`shouldSkip()`。从而进行`AspectJPointcutAdvisor#0`对象创建
* 2... AspectJAwareAdvisorAutoProxyCreator => shouldSkip(),非AOP对象执行,进而创建并缓存AOP对象
*/
if (isInfrastructureClass(beanClass) || shouldSkip(beanClass, beanName)) {
// 要跳过的直接设置FALSE
this.advisedBeans.put(cacheKey, Boolean.FALSE);
return null;
}
}
// Create proxy here if we have a custom TargetSource.
// Suppresses unnecessary default instantiation of the target bean:
// The TargetSource will handle target instances in a custom fashion.
// 3. 用户自定义proxy对象创建,默认`targetSource = null`
TargetSource targetSource = getCustomTargetSource(beanClass, beanName);
if (targetSource != null) {
if (StringUtils.hasLength(beanName)) {
this.targetSourcedBeans.add(beanName);
}
Object[] specificInterceptors = getAdvicesAndAdvisorsForBean(beanClass, beanName, targetSource);
Object proxy = createProxy(beanClass, beanName, specificInterceptors, targetSource);
this.proxyTypes.put(cacheKey, proxy.getClass());
return proxy;
}
return null;
}
protected boolean isInfrastructureClass(Class<?> beanClass) {
boolean retVal = Advice.class.isAssignableFrom(beanClass) ||
Pointcut.class.isAssignableFrom(beanClass) ||
Advisor.class.isAssignableFrom(beanClass) ||
AopInfrastructureBean.class.isAssignableFrom(beanClass);
if (retVal && logger.isTraceEnabled()) {
logger.trace("Did not attempt to auto-proxy infrastructure class [" + beanClass.getName() + "]");
}
return retVal;
}
protected boolean shouldSkip(Class<?> beanClass, String beanName) {
return AutoProxyUtils.isOriginalInstance(beanName, beanClass); // .ORIGINAL
}
}
2. AbstractAdvisorAutoProxyCreator
public abstract class AbstractAdvisorAutoProxyCreator extends AbstractAutoProxyCreator {
@Nullable
private BeanFactoryAdvisorRetrievalHelper advisorRetrievalHelper;
protected void initBeanFactory(ConfigurableListableBeanFactory beanFactory) {
this.advisorRetrievalHelper = new BeanFactoryAdvisorRetrievalHelperAdapter(beanFactory);
}
/**
* Find all candidate Advisors to use in auto-proxying.
* @return the List of candidate Advisors
*/
protected List<Advisor> findCandidateAdvisors() {
// 1... BeanFactoryAdvisorRetrievalHelper recursive子父类advisor
// `AspectJPointcutAdvisor#0-4`进行一级缓存
return this.advisorRetrievalHelper.findAdvisorBeans();
}
}
1. BeanFactoryAdvisorRetrievalHelper
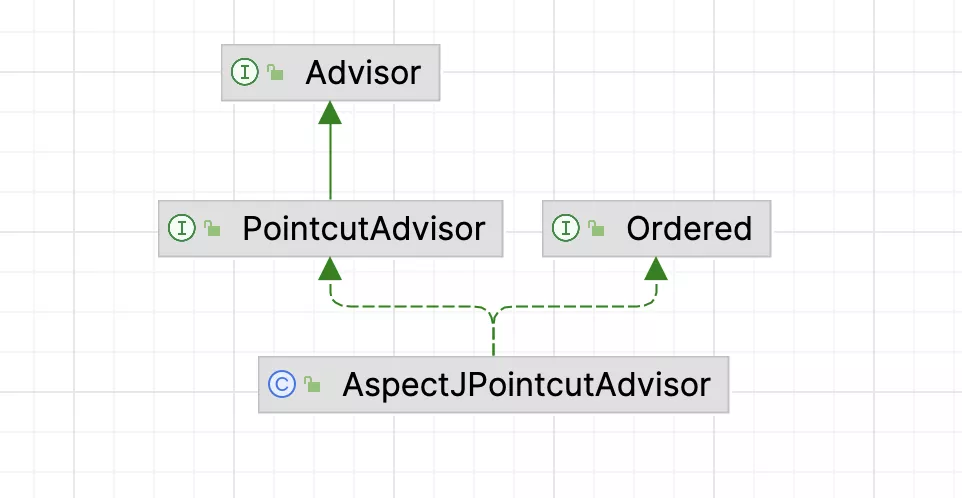
public class BeanFactoryAdvisorRetrievalHelper {
public List<Advisor> findAdvisorBeans() {
// Determine list of advisor bean names, if not cached already.
String[] advisorNames = this.cachedAdvisorBeanNames;
if (advisorNames == null) {
// Do not initialize FactoryBeans here: We need to leave all regular beans
// uninitialized to let the auto-proxy creator apply to them!
/**
* 1. recursive子父类advisor
*/
advisorNames = BeanFactoryUtils.beanNamesForTypeIncludingAncestors(
this.beanFactory, Advisor.class, true, false);
this.cachedAdvisorBeanNames = advisorNames;
}
if (advisorNames.length == 0) {
return new ArrayList<>();
}
List<Advisor> advisors = new ArrayList<>();
for (String name : advisorNames) {
if (isEligibleBean(name)) { // 子类提供的hook(),对Advisor进行过滤,默认为true
// 2. BF获取
advisors.add(this.beanFactory.getBean(name, Advisor.class));
}
}
return advisors;
}
protected boolean isEligibleBean(String beanName) {
return true;
}
}
3. AspectJAwareAdvisorAutoProxyCreator
public class AspectJAwareAdvisorAutoProxyCreator extends AbstractAdvisorAutoProxyCreator {
@Override
protected boolean shouldSkip(Class<?> beanClass, String beanName) {
// TODO: Consider optimization by caching the list of the aspect names
// 1. AbstractAdvisorAutoProxyCreator recursion获取所有子父类advisor,并缓存
List<Advisor> candidateAdvisors = findCandidateAdvisors();
// 2. `<aop:aspect ref="logUtil">`直接跳过
for (Advisor advisor : candidateAdvisors) {
if (advisor instanceof AspectJPointcutAdvisor &&
((AspectJPointcutAdvisor) advisor).getAspectName().equals(beanName)) {
return true;
}
}
// 3. `.ORIGINAL` 后缀对象判断
return super.shouldSkip(beanClass, beanName);
}
}
4. AnnoAwareAspectJAutoProxyCreator
- Advisor接口xml_bean
@Aspect
标注的java_bean,@Before, @After
方法
public class AnnotationAwareAspectJAutoProxyCreator extends AspectJAwareAdvisorAutoProxyCreator {
@Nullable
private AspectJAdvisorFactory aspectJAdvisorFactory;
@Nullable
private BeanFactoryAspectJAdvisorsBuilder aspectJAdvisorsBuilder;
@Override
protected void initBeanFactory(ConfigurableListableBeanFactory beanFactory) {
super.initBeanFactory(beanFactory);
if (this.aspectJAdvisorFactory == null) {
this.aspectJAdvisorFactory = new ReflectiveAspectJAdvisorFactory(beanFactory);
}
// 1. ReflectiveAspectJAdvisorFactory
this.aspectJAdvisorsBuilder =
new BeanFactoryAspectJAdvisorsBuilderAdapter(beanFactory, this.aspectJAdvisorFactory);
}
/**
* `<aop:aspectj-autoproxy/>`,xml里配置这个才走子类,否则走父类
*/
@Override
protected List<Advisor> findCandidateAdvisors() {
// Add all the Spring advisors found according to superclass rules.
// 1... AspectJAwareAdvisorAutoProxyCreator Advisor接口xml_bean
List<Advisor> advisors = super.findCandidateAdvisors();
// Build Advisors for all AspectJ aspects in the bean factory.
if (this.aspectJAdvisorsBuilder != null) {
// 2... BeanFactoryAspectJAdvisorsBuilder @Aspect标注的java_bean,`@Before, @After`方法
advisors.addAll(this.aspectJAdvisorsBuilder.buildAspectJAdvisors());
}
return advisors;
}
// --------------------------------------------------------------------------------------------------
private class BeanFactoryAspectJAdvisorsBuilderAdapter extends BeanFactoryAspectJAdvisorsBuilder {
public BeanFactoryAspectJAdvisorsBuilderAdapter(
ListableBeanFactory beanFactory, AspectJAdvisorFactory advisorFactory) {
super(beanFactory, advisorFactory);
}
@Override
protected boolean isEligibleBean(String beanName) {
return AnnotationAwareAspectJAutoProxyCreator.this.isEligibleAspectBean(beanName);
}
}
}
1. BeanFactoryAspectJAdvisorsBuilder
@Aspect
修饰类- 该类获取
Advisor
this.advisorsCache.put(beanName, classAdvisors);
进行缓存
public class BeanFactoryAspectJAdvisorsBuilder {
private final ListableBeanFactory beanFactory;
// 1. ReflectiveAspectJAdvisorFactory
private final AspectJAdvisorFactory advisorFactory;
@Nullable
private volatile List<String> aspectBeanNames;
private final Map<String, List<Advisor>> advisorsCache = new ConcurrentHashMap<>(); // 单例缓存
// prototype_factory缓存
private final Map<String, MetadataAwareAspectInstanceFactory> aspectFactoryCache = new ConcurrentHashMap<>();
public BeanFactoryAspectJAdvisorsBuilder(ListableBeanFactory beanFactory, AspectJAdvisorFactory advisorFactory) {
this.beanFactory = beanFactory;
this.advisorFactory = advisorFactory;
}
public List<Advisor> buildAspectJAdvisors() {
// 获取切面名字列表
List<String> aspectNames = this.aspectBeanNames;
// 缓存字段aspectNames没有值,注意实例化第一个单实例bean的时候就会触发解析切面
if (aspectNames == null) {
// 双重检查
synchronized (this) {
aspectNames = this.aspectBeanNames;
if (aspectNames == null) {
// 用于保存所有解析出来的Advisors集合对象
List<Advisor> advisors = new ArrayList<>();
// 用于保存切面的名称的集合
aspectNames = new ArrayList<>();
/*
* 1. AOP功能中在这里传入的是Object对象,代表去容器中获取到所有的组件的名称,然后再
* 进行遍历,这个过程是十分的消耗性能的,所以说Spring会再这里加入了保存切面信息的缓存。
* 但是事务功能不一样,事务模块的功能是直接去容器中获取Advisor类型的,选择范围小,且不消耗性能。
* 所以Spring在事务模块中没有加入缓存来保存我们的事务相关的advisor
*/
String[] beanNames = BeanFactoryUtils.beanNamesForTypeIncludingAncestors(
this.beanFactory, Object.class, true, false);
// 遍历我们从IOC容器中获取处的所有Bean的名称
for (String beanName : beanNames) {
// 判断当前bean是否为子类定制的需要过滤的bean
if (!isEligibleBean(beanName)) {
continue;
}
// We must be careful not to instantiate beans eagerly as in this case they
// would be cached by the Spring container but would not have been weaved.
// 2. 通过beanName去容器中获取到对应class对象
Class<?> beanType = this.beanFactory.getType(beanName, false);
if (beanType == null) {
continue;
}
// 3... AbstractAspectJAdvisorFactory java_bean是否被@Aspect修饰
if (this.advisorFactory.isAspect(beanType)) {
aspectNames.add(beanName);
// 对于使用了@Aspect注解标注的bean,将其封装为一个AspectMetadata类型。
// 这里在封装的过程中会解析@Aspect注解上的参数指定的切面类型,如perthis
// 和pertarget等。这些被解析的注解都会被封装到其perClausePointcut属性中
AspectMetadata amd = new AspectMetadata(beanType, beanName);
// 判断@Aspect注解中标注的是否为singleton类型,默认的切面类都是singleton类型
if (amd.getAjType().getPerClause().getKind() == PerClauseKind.SINGLETON) {
// 4. 当前bean封装为MetadataAwareAspectInstanceFactory对象,再次将@Aspect参数封装
MetadataAwareAspectInstanceFactory factory =
new BeanFactoryAspectInstanceFactory(this.beanFactory, beanName);
// 5... ReflectiveAspectJAdvisorFactory Advice解析并封装为Advisor
List<Advisor> classAdvisors = this.advisorFactory.getAdvisors(factory);
if (this.beanFactory.isSingleton(beanName)) {
// 6.1. Advisor进行缓存
this.advisorsCache.put(beanName, classAdvisors);
}
// 6.2. factory进行缓存
else {
this.aspectFactoryCache.put(beanName, factory);
}
advisors.addAll(classAdvisors);
}
else {
// Per target or per this.
// 如果@Aspect注解标注的是perthis和pertarget类型,说明当前切面
// 不可能是单例的,因而这里判断其如果是单例的则抛出异常
if (this.beanFactory.isSingleton(beanName)) {
throw new IllegalArgumentException("Bean with name '" + beanName +
"' is a singleton, but aspect instantiation model is not singleton");
}
// 将当前BeanFactory和切面bean封装为一个多例类型的Factory
MetadataAwareAspectInstanceFactory factory =
new PrototypeAspectInstanceFactory(this.beanFactory, beanName);
// 对当前bean和factory进行缓存
this.aspectFactoryCache.put(beanName, factory);
advisors.addAll(this.advisorFactory.getAdvisors(factory));
}
}
}
this.aspectBeanNames = aspectNames;
return advisors;
}
}
}
if (aspectNames.isEmpty()) {
return Collections.emptyList();
}
// 通过所有的aspectNames在缓存中获取切面对应的Advisor,这里如果是单例的,则直接从advisorsCache
// 获取,如果是多例类型的,则通过MetadataAwareAspectInstanceFactory立即生成一个
List<Advisor> advisors = new ArrayList<>();
for (String aspectName : aspectNames) {
List<Advisor> cachedAdvisors = this.advisorsCache.get(aspectName);
// 如果是单例的Advisor bean,则直接添加到返回值列表中
if (cachedAdvisors != null) {
advisors.addAll(cachedAdvisors);
}
else {
// 如果是多例的Advisor bean,则通过MetadataAwareAspectInstanceFactory生成
MetadataAwareAspectInstanceFactory factory = this.aspectFactoryCache.get(aspectName);
advisors.addAll(this.advisorFactory.getAdvisors(factory));
}
}
return advisors;
}
}
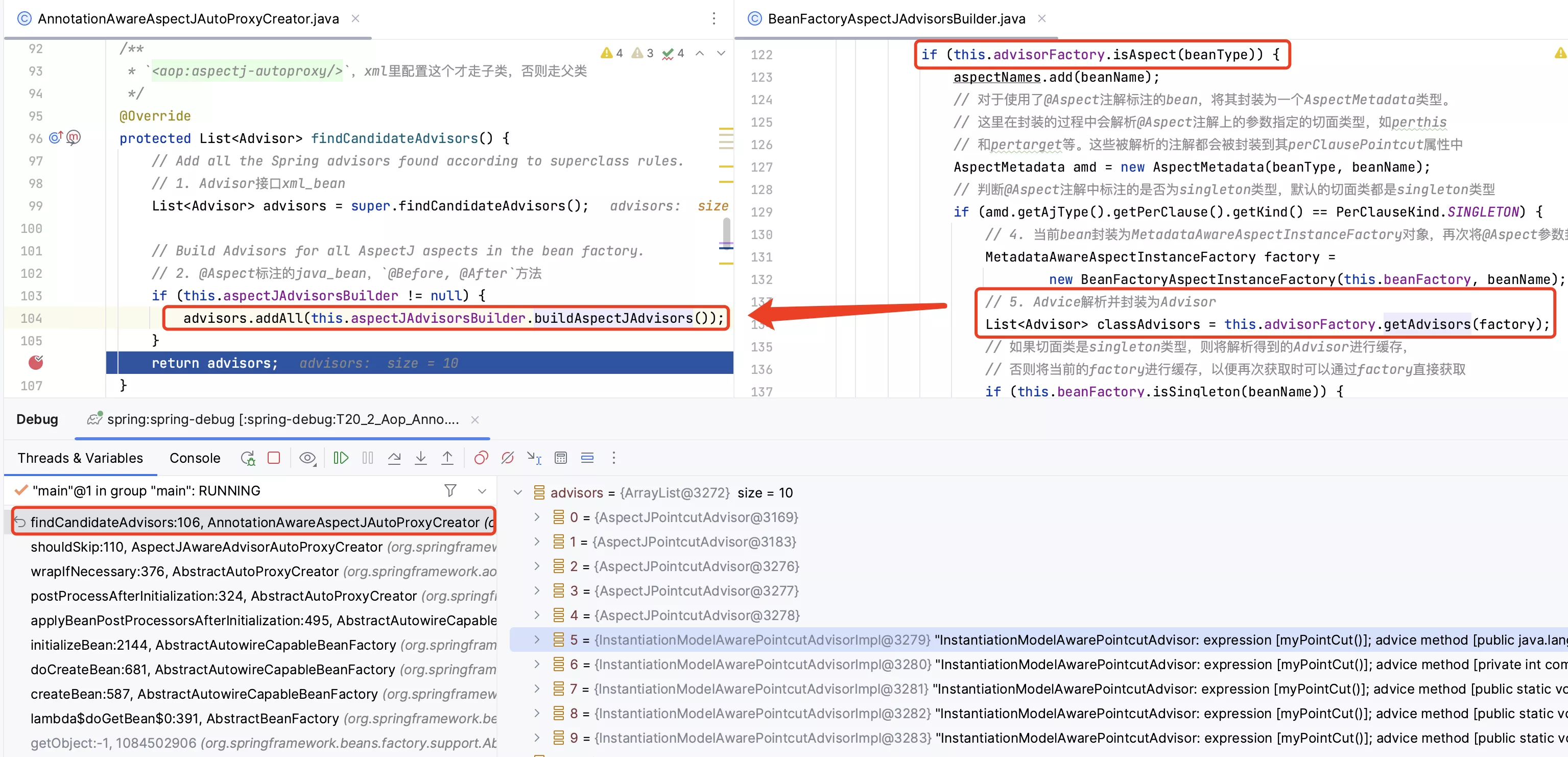
2. ReflectiveAspectJAdvisorFactory
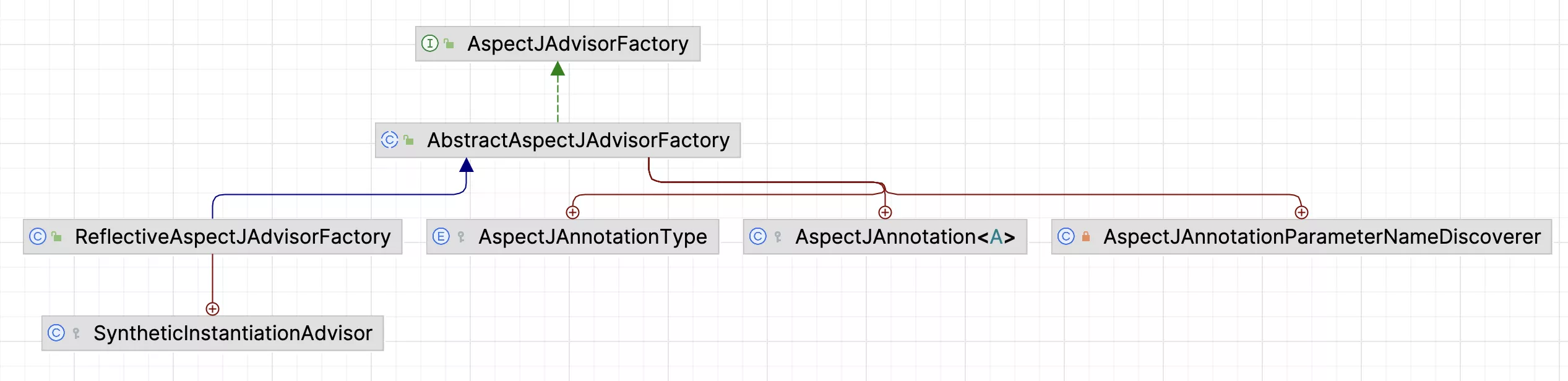
- 获取非
@Pointcut
注解的方法(包含无注解) @Before, @After, @Around, @AfterReturning, @AfterThrowing
=> InstantiationModelAwarePointcutAdvisorImpl(Advisor)排除无注解方法
public class ReflectiveAspectJAdvisorFactory extends AbstractAspectJAdvisorFactory implements Serializable {
private static final Comparator<Method> METHOD_COMPARATOR;
static {
// Note: although @After is ordered before @AfterReturning and @AfterThrowing,
// an @After advice method will actually be invoked after @AfterReturning and
// @AfterThrowing methods due to the fact that AspectJAfterAdvice.invoke(MethodInvocation)
// invokes proceed() in a `try` block and only invokes the @After advice method
// in a corresponding `finally` block.
Comparator<Method> adviceKindComparator = new ConvertingComparator<>(
new InstanceComparator<>(
Around.class, Before.class, After.class, AfterReturning.class, AfterThrowing.class),
(Converter<Method, Annotation>) method -> {
AspectJAnnotation<?> ann = AbstractAspectJAdvisorFactory.findAspectJAnnotationOnMethod(method);
return (ann != null ? ann.getAnnotation() : null);
});
Comparator<Method> methodNameComparator = new ConvertingComparator<>(Method::getName);
METHOD_COMPARATOR = adviceKindComparator.thenComparing(methodNameComparator);
}
@Override
public List<Advisor> getAdvisors(MetadataAwareAspectInstanceFactory aspectInstanceFactory) {
// 1. 获取标记为AspectJ的类
Class<?> aspectClass = aspectInstanceFactory.getAspectMetadata().getAspectClass();
// 获取标记为AspectJ的name
String aspectName = aspectInstanceFactory.getAspectMetadata().getAspectName();
// 对当前切面bean进行校验,主要是判断其切点是否为perflow或者是percflowbelow,Spring暂时不支持
// 这两种类型的切点
validate(aspectClass);
// We need to wrap the MetadataAwareAspectInstanceFactory with a decorator
// so that it will only instantiate once.
// 将当前aspectInstanceFactory进行封装,这里LazySingletonAspectInstanceFactoryDecorator
// 使用装饰器模式,主要是对获取到的切面实例进行了缓存,保证每次获取到的都是同一个切面实例
MetadataAwareAspectInstanceFactory lazySingletonAspectInstanceFactory =
new LazySingletonAspectInstanceFactoryDecorator(aspectInstanceFactory);
List<Advisor> advisors = new ArrayList<>();
// 2.. 获取非@Pointcut注解的方法(包含无注解)
for (Method method : getAdvisorMethods(aspectClass)) {
// Prior to Spring Framework 5.2.7, advisors.size() was supplied as the declarationOrderInAspect
// to getAdvisor(...) to represent the "current position" in the declared methods list.
// However, since Java 7 the "current position" is not valid since the JDK no longer
// returns declared methods in the order in which they are declared in the source code.
// Thus, we now hard code the declarationOrderInAspect to 0 for all advice methods
// discovered via reflection in order to support reliable advice ordering across JVM launches.
// Specifically, a value of 0 aligns with the default value used in
// AspectJPrecedenceComparator.getAspectDeclarationOrder(Advisor).
// 3.. @Before, @After, @Around, @AfterReturning, @AfterThrowing => Advisor(排除无注解方法)
Advisor advisor = getAdvisor(method, lazySingletonAspectInstanceFactory, 0, aspectName);
if (advisor != null) {
advisors.add(advisor);
}
}
// If it's a per target aspect, emit the dummy instantiating aspect.
// 这里的isLazilyInstantiated()方法判断的是当前bean是否应该被延迟初始化,其主要是判断当前
// 切面类是否为perthis,pertarget或pertypewithiin等声明的切面。因为这些类型所环绕的目标bean
// 都是多例的,因而需要在运行时动态判断目标bean是否需要环绕当前的切面逻辑
if (!advisors.isEmpty() && lazySingletonAspectInstanceFactory.getAspectMetadata().isLazilyInstantiated()) {
// 如果Advisor不为空,并且是需要延迟初始化的bean,则在第0位位置添加一个同步增强器,
// 该同步增强器实际上就是一个BeforeAspect的Advisor
Advisor instantiationAdvisor = new SyntheticInstantiationAdvisor(lazySingletonAspectInstanceFactory);
advisors.add(0, instantiationAdvisor);
}
// Find introduction fields.
// 判断属性上是否包含有@DeclareParents注解标注的需要新添加的属性,如果有,则将其封装为一个Advisor
for (Field field : aspectClass.getDeclaredFields()) {
Advisor advisor = getDeclareParentsAdvisor(field);
if (advisor != null) {
advisors.add(advisor);
}
}
return advisors;
}
// ------------------------------------------------------------------------------------------
/**
* 1. 获取所有不包含Pointcut注解的方法
* 2. 进行排序。顺序Around, Before, After, AfterReturning, AfterThrowing
*/
private List<Method> getAdvisorMethods(Class<?> aspectClass) {
final List<Method> methods = new ArrayList<>();
// ReflectionUtils.MethodCallback的匿名实现
ReflectionUtils.doWithMethods(aspectClass, method -> {
// Exclude pointcuts
// 1. @Pointcut方法不处理
if (AnnotationUtils.getAnnotation(method, Pointcut.class) == null) {
methods.add(method);
}
}, ReflectionUtils.USER_DECLARED_METHODS);
if (methods.size() > 1) {
methods.sort(METHOD_COMPARATOR);
}
return methods;
}
@Override
@Nullable
public Advisor getAdvisor(Method candidateAdviceMethod, MetadataAwareAspectInstanceFactory aspectInstanceFactory,
int declarationOrderInAspect, String aspectName) {
// 校验当前切面类是否使用了perflow或者percflowbelow标识的切点,Spring暂不支持这两种切点
validate(aspectInstanceFactory.getAspectMetadata().getAspectClass());
// 1.. 封装AspectJExpressionPointcut
AspectJExpressionPointcut expressionPointcut = getPointcut(
candidateAdviceMethod, aspectInstanceFactory.getAspectMetadata().getAspectClass());
if (expressionPointcut == null) {
return null;
}
// 99. InstantiationModelAwarePointcutAdvisorImpl
return new InstantiationModelAwarePointcutAdvisorImpl(expressionPointcut, candidateAdviceMethod,
this, aspectInstanceFactory, declarationOrderInAspect, aspectName);
}
@Nullable
private AspectJExpressionPointcut getPointcut(Method candidateAdviceMethod, Class<?> candidateAspectClass) {
// 1... AbstractAspectJAdvisorFactory 获取方法上注解
AspectJAnnotation<?> aspectJAnnotation =
AbstractAspectJAdvisorFactory.findAspectJAnnotationOnMethod(candidateAdviceMethod);
if (aspectJAnnotation == null) {
return null;
}
// 2. 使用AspectJExpressionPointcut实例封装获取的信息
AspectJExpressionPointcut ajexp =
new AspectJExpressionPointcut(candidateAspectClass, new String[0], new Class<?>[0]);
// 提取得到的注解中的表达式
ajexp.setExpression(aspectJAnnotation.getPointcutExpression());
if (this.beanFactory != null) {
ajexp.setBeanFactory(this.beanFactory);
}
return ajexp;
}
}
1. AbstractAspectJAdvisorFactory
public abstract class AbstractAspectJAdvisorFactory implements AspectJAdvisorFactory {
private static final Class<?>[] ASPECTJ_ANNOTATION_CLASSES = new Class<?>[] {
Pointcut.class, Around.class, Before.class, After.class, AfterReturning.class, AfterThrowing.class};
@Override
public boolean isAspect(Class<?> clazz) {
// 1..
return (hasAspectAnnotation(clazz) && !compiledByAjc(clazz));
}
private boolean hasAspectAnnotation(Class<?> clazz) {
// 1. @Aspect
return (AnnotationUtils.findAnnotation(clazz, Aspect.class) != null);
}
// ------------------------------------------------------------------------------------
@Nullable
protected static AspectJAnnotation<?> findAspectJAnnotationOnMethod(Method method) {
// 方法注解匹配(Pointcut, Around, Before, After, AfterReturning, AfterThrowing)
for (Class<?> clazz : ASPECTJ_ANNOTATION_CLASSES) {
// 1.. Pointcut, Around, Before, After, AfterReturning, AfterThrowing
AspectJAnnotation<?> foundAnnotation = findAnnotation(method, (Class<Annotation>) clazz);
if (foundAnnotation != null) {
return foundAnnotation;
}
}
return null;
}
@Nullable
private static <A extends Annotation> AspectJAnnotation<A> findAnnotation(Method method, Class<A> toLookFor) {
// 1.
A result = AnnotationUtils.findAnnotation(method, toLookFor);
if (result != null) {
// 2.
return new AspectJAnnotation<>(result);
}
else {
return null;
}
}
}
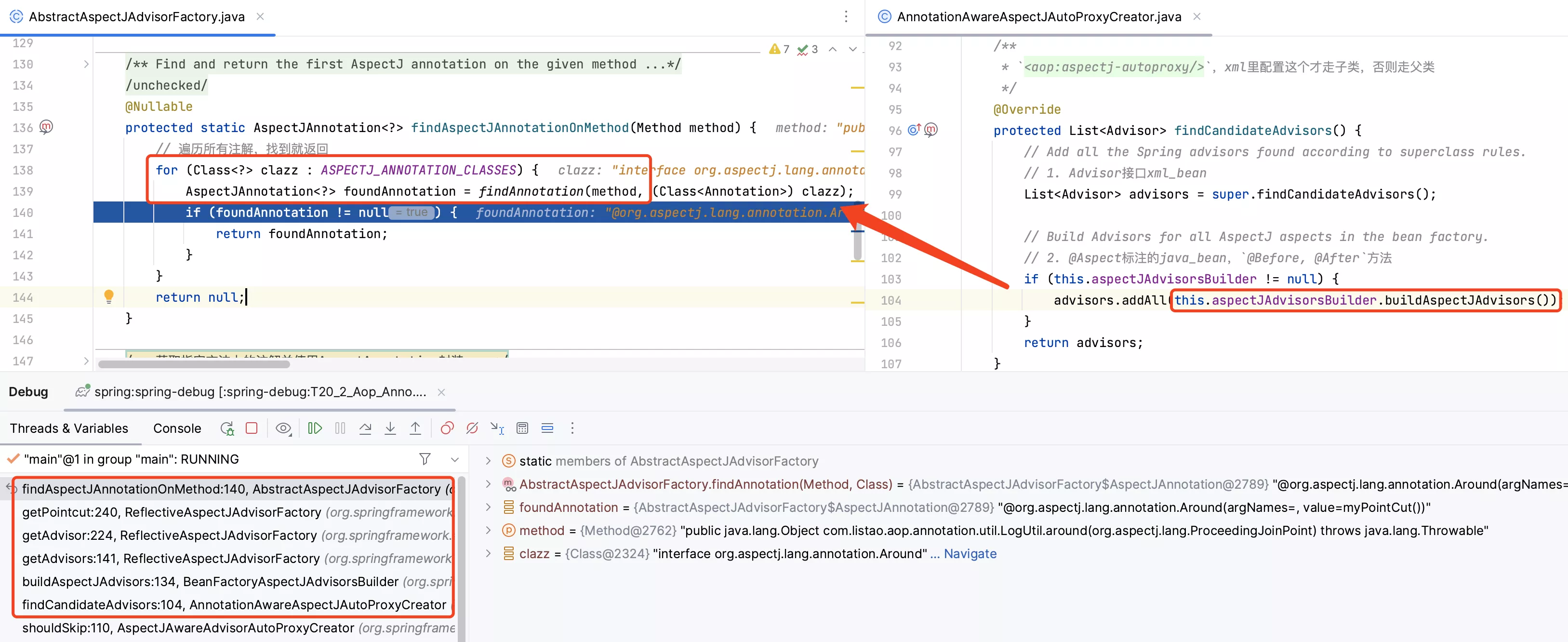
5. AspectJPointcutAdvisor#0
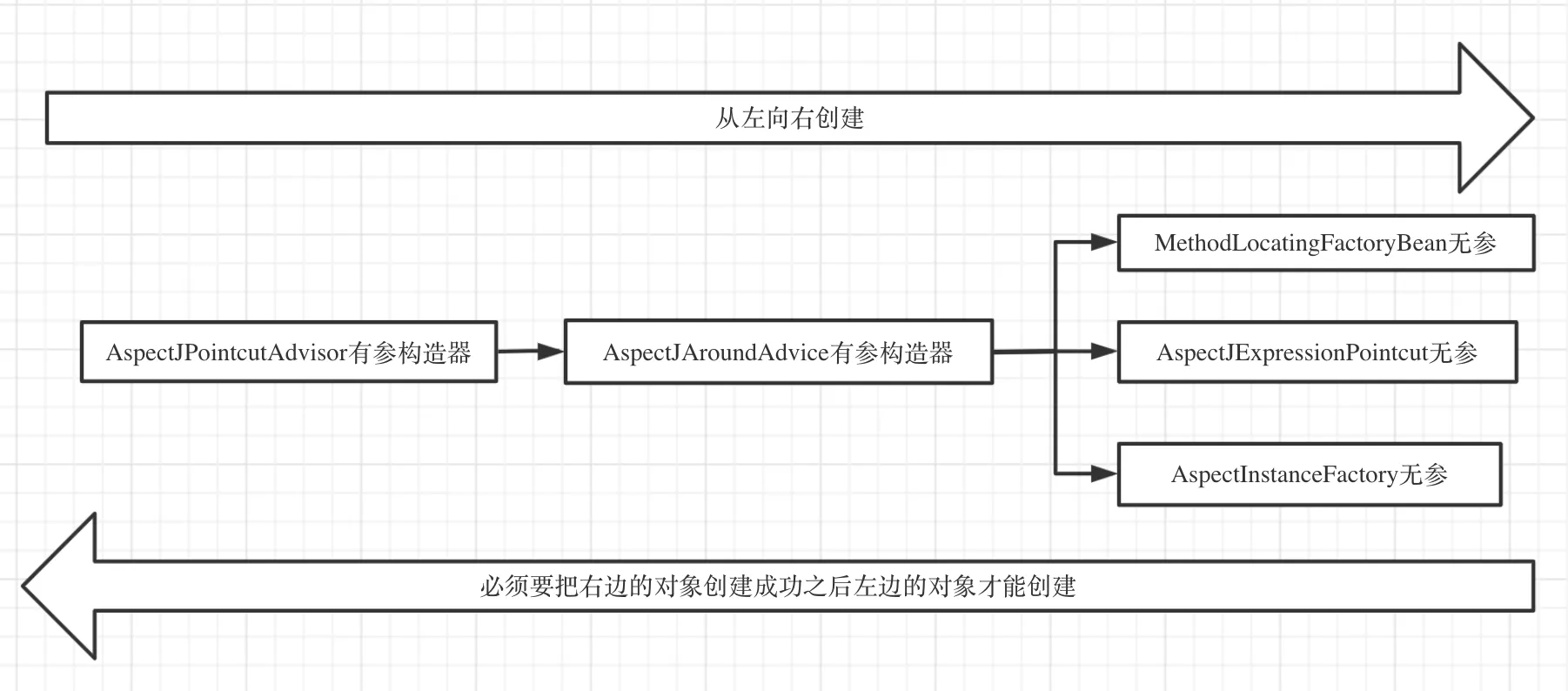
- 创建
AspectJPointcutAdvisor#0-4
,有参构造,创建内置对象AspectJAroundAdvice
AspectJAroundAdvice
,有参构造,创建三个内置对象- MethodLocatingFactoryBean,
instanceof BeanDefinition
- 无参构造,直接反射 =>
implements FactoryBean<Method>
进行getObject()
获取Method对象
- 无参构造,直接反射 =>
- AspectJExpressionPointcut,
instanceof RuntimeBeanReference
- 无参构造,直接反射 prototype
- SimpleBeanFactoryAwareAspectInstanceFactory,
instanceof BeanDefinition
- 无参构造,直接反射
- MethodLocatingFactoryBean,
AbstractAutowireCapableBeanFactory#createBean()
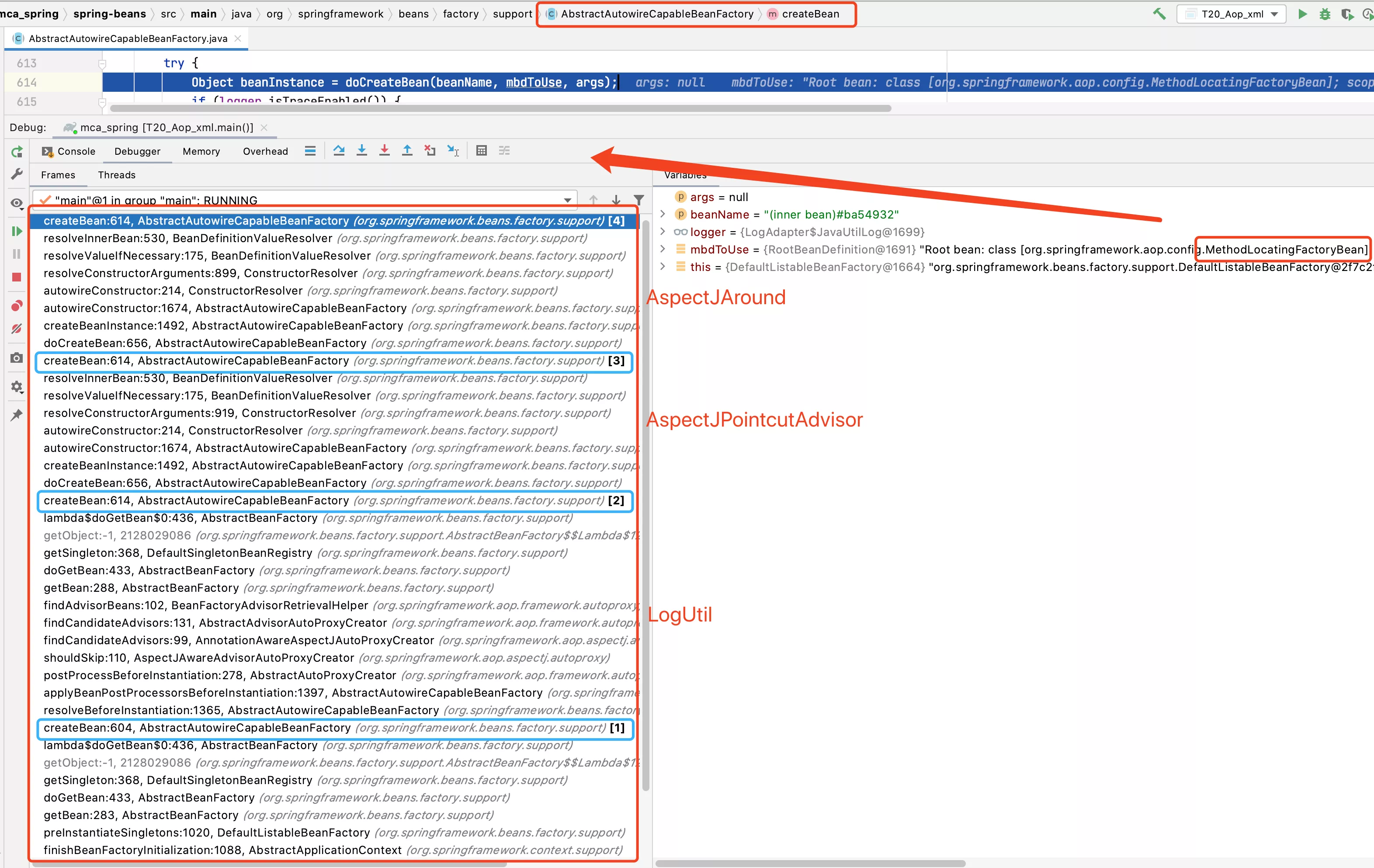
// MethodLocatingFactoryBean 中提取Method对象
// class MethodLocatingFactoryBean implements FactoryBean<Method>, BeanFactoryAware
Object innerBean = this.beanFactory.createBean(actualInnerBeanName, mbd, null);
if (innerBean instanceof FactoryBean) {
boolean synthetic = mbd.isSynthetic();
innerBean = this.beanFactory.getObjectFromFactoryBean(
(FactoryBean<?>) innerBean, actualInnerBeanName, !synthetic);
}
3. applyBPPAfterInitialization()
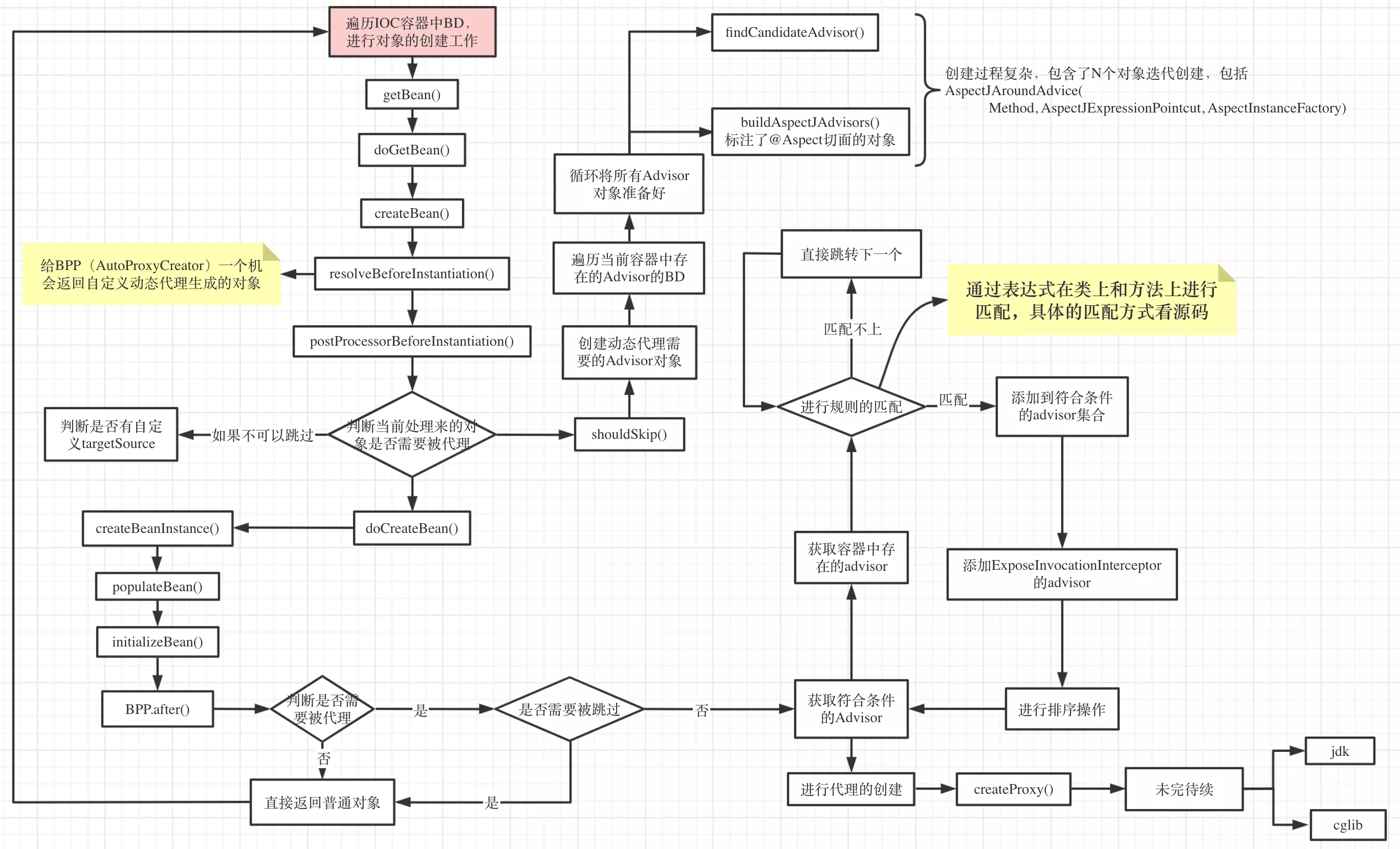
1. AbstractAutoProxyCreator
getAdvicesAndAdvisorsForBean()
通过bean.clazz获取匹配的InterceptorcreateProxy()
=> (specificInterceptors + 原bean) 生成代理对象
public abstract class AbstractAutoProxyCreator extends ProxyProcessorSupport
implements SmartInstantiationAwareBeanPostProcessor, BeanFactoryAware {
@Override
public Object getEarlyBeanReference(Object bean, String beanName) {
Object cacheKey = getCacheKey(bean.getClass(), beanName);
// 标志位`已AOP`
this.earlyProxyReferences.put(cacheKey, bean);
return wrapIfNecessary(bean, beanName, cacheKey);
}
@Override
public Object postProcessAfterInitialization(@Nullable Object bean, String beanName) {
if (bean != null) {
Object cacheKey = getCacheKey(bean.getClass(), beanName);
if (this.earlyProxyReferences.remove(cacheKey) != bean) {
return wrapIfNecessary(bean, beanName, cacheKey);
}
}
return bean;
}
// ---------------------------------------------------------------------------------------
protected Object wrapIfNecessary(Object bean, String beanName, Object cacheKey) {
// targetSourcedBeans
if (StringUtils.hasLength(beanName) && this.targetSourcedBeans.contains(beanName)) {
return bean;
}
// advisedBean为通知bean
if (Boolean.FALSE.equals(this.advisedBeans.get(cacheKey))) {
return bean;
}
// isInfrastructureClass() => 是否Spring_bean,不用代理
// shouldSkip() => 则用于判断当前bean是否应该被略过
if (isInfrastructureClass(bean.getClass()) || shouldSkip(bean.getClass(), beanName)) {
this.advisedBeans.put(cacheKey, Boolean.FALSE);
return bean;
}
// 1.. Create proxy if we have advice.
Object[] specificInterceptors = getAdvicesAndAdvisorsForBean(bean.getClass(), beanName, null);
if (specificInterceptors != DO_NOT_PROXY) { // null
this.advisedBeans.put(cacheKey, Boolean.TRUE);
// 2.. (specificInterceptors + 原bean) 生成代理对象
Object proxy = createProxy(
bean.getClass(), beanName, specificInterceptors, new SingletonTargetSource(bean));
this.proxyTypes.put(cacheKey, proxy.getClass());
return proxy;
}
this.advisedBeans.put(cacheKey, Boolean.FALSE);
return bean;
}
// ---------------------------------------------------------------------------------------
// 遍历advisors,bean中任意一个方法能匹配,保留该advisor
protected Object[] getAdvicesAndAdvisorsForBean(
Class<?> beanClass, String beanName, @Nullable TargetSource targetSource) {
// 1..
List<Advisor> advisors = findEligibleAdvisors(beanClass, beanName);
if (advisors.isEmpty()) {
return DO_NOT_PROXY;
}
return advisors.toArray();
}
protected List<Advisor> findEligibleAdvisors(Class<?> beanClass, String beanName) {
// 1... AbstractAdvisorAutoProxyCreator AnnotationAwareAspectJAutoProxyCreator => Advisor集合
List<Advisor> candidateAdvisors = findCandidateAdvisors();
// 2.. 适配bean的Advisor
List<Advisor> eligibleAdvisors = findAdvisorsThatCanApply(candidateAdvisors, beanClass, beanName);
// 3... AspectJAwareAdvisorAutoProxyCreator 提供hook(),增加advisor_ExposeInvocationInterceptor
extendAdvisors(eligibleAdvisors);
if (!eligibleAdvisors.isEmpty()) {
// 4... AspectJAwareAdvisorAutoProxyCreator topo-sort拓扑排序,有向无环图
eligibleAdvisors = sortAdvisors(eligibleAdvisors); // 没必要看排序算法,晕死...
}
return eligibleAdvisors;
}
protected List<Advisor> findAdvisorsThatCanApply(
List<Advisor> candidateAdvisors, Class<?> beanClass, String beanName) {
ProxyCreationContext.setCurrentProxiedBeanName(beanName);
try {
// 1... candidateAdvisors筛选当前beanClass的advisor
return AopUtils.findAdvisorsThatCanApply(candidateAdvisors, beanClass);
} finally {
ProxyCreationContext.setCurrentProxiedBeanName(null);
}
}
}
Advisor
PointcutAdvisor
=> 切点通知,方法级别增强IntroductionAdvisor
=> 引介通知,类级别增加
// 回调信息类型
class CallbackInfo {
static {
CALLBACKS = new CallbackInfo[]{
new CallbackInfo(NoOp.class, NoOpGenerator.INSTANCE),
new CallbackInfo(MethodInterceptor.class, MethodInterceptorGenerator.INSTANCE),
new CallbackInfo(InvocationHandler.class, InvocationHandlerGenerator.INSTANCE),
new CallbackInfo(LazyLoader.class, LazyLoaderGenerator.INSTANCE),
new CallbackInfo(Dispatcher.class, DispatcherGenerator.INSTANCE),
new CallbackInfo(FixedValue.class, FixedValueGenerator.INSTANCE),
new CallbackInfo(ProxyRefDispatcher.class, DispatcherGenerator.PROXY_REF_INSTANCE)};
}
}
1. AopUtils
expression="execution(Integer com.listao.aop.xml.service.MyCalc.* (..))"
public class AspectJExpressionPointcut extends AbstractExpressionPointcut
implements ClassFilter, IntroductionAwareMethodMatcher, BeanFactoryAware {
}
ClassFilter.java
类过滤MethodMatcher.java
方法过滤
public abstract class AopUtils {
public static List<Advisor> findAdvisorsThatCanApply(List<Advisor> candidateAdvisors, Class<?> clazz) {
if (candidateAdvisors.isEmpty()) {
return candidateAdvisors;
}
List<Advisor> eligibleAdvisors = new ArrayList<>();
for (Advisor candidate : candidateAdvisors) {
// 1. IntroductionAdvisor类级别
if (candidate instanceof IntroductionAdvisor && canApply(candidate, clazz)) {
eligibleAdvisors.add(candidate);
}
}
// 是否引介增强
boolean hasIntroductions = !eligibleAdvisors.isEmpty();
for (Advisor candidate : candidateAdvisors) {
if (candidate instanceof IntroductionAdvisor) {
// already processed
continue;
}
// 2.. 判断advisor是否应用当前bean
if (canApply(candidate, clazz, hasIntroductions)) {
eligibleAdvisors.add(candidate);
}
}
return eligibleAdvisors;
}
public static boolean canApply(Advisor advisor, Class<?> targetClass, boolean hasIntroductions) {
// 1. IntroductionAdvisor类型,ClassFilter.matches()
if (advisor instanceof IntroductionAdvisor) {
return ((IntroductionAdvisor) advisor).getClassFilter().matches(targetClass);
} else if (advisor instanceof PointcutAdvisor) {
PointcutAdvisor pca = (PointcutAdvisor) advisor;
// 2.. 从Advisor中获取Pointcut实现类 => AspectJExpressionPointcut
return canApply(pca.getPointcut(), targetClass, hasIntroductions);
} else {
// It doesn't have a pointcut so we assume it applies.
return true;
}
}
public static boolean canApply(Pointcut pc, Class<?> targetClass, boolean hasIntroductions) {
// 1. ClassFilter.matches()。表达式中类匹配,没必要看懂
if (!pc.getClassFilter().matches(targetClass)) {
return false;
}
// MethodMatcher.matches()
MethodMatcher methodMatcher = pc.getMethodMatcher();
if (methodMatcher == MethodMatcher.TRUE) {
// No need to iterate the methods if we're matching any method anyway...
return true;
}
IntroductionAwareMethodMatcher introductionAwareMethodMatcher = null;
if (methodMatcher instanceof IntroductionAwareMethodMatcher) {
introductionAwareMethodMatcher = (IntroductionAwareMethodMatcher) methodMatcher;
}
Set<Class<?>> classes = new LinkedHashSet<>();
if (!Proxy.isProxyClass(targetClass)) {
classes.add(ClassUtils.getUserClass(targetClass));
}
// targetClass实现接口的class对象
classes.addAll(ClassUtils.getAllInterfacesForClassAsSet(targetClass));
for (Class<?> clazz : classes) {
// clazz.Methods
Method[] methods = ReflectionUtils.getAllDeclaredMethods(clazz);
for (Method method : methods) {
// 2. bean中任意一个方法能匹配,保留该advisor
if (introductionAwareMethodMatcher != null ?
introductionAwareMethodMatcher.matches(method, targetClass, hasIntroductions) :
methodMatcher.matches(method, targetClass)) {
return true;
}
}
}
return false;
}
}
2. AspectJAwareAdvisorAutoProxyCreator
- 提供
hook()
,增加advisor_ExposeInvocationInterceptor
public class AspectJAwareAdvisorAutoProxyCreator extends AbstractAdvisorAutoProxyCreator {
@Override
protected void extendAdvisors(List<Advisor> candidateAdvisors) {
// 1...
AspectJProxyUtils.makeAdvisorChainAspectJCapableIfNecessary(candidateAdvisors);
}
@Override
protected List<Advisor> sortAdvisors(List<Advisor> advisors) {
List<PartiallyComparableAdvisorHolder> partiallyComparableAdvisors = new ArrayList<>(advisors.size());
for (Advisor advisor : advisors) {
partiallyComparableAdvisors.add(
new PartiallyComparableAdvisorHolder(advisor, DEFAULT_PRECEDENCE_COMPARATOR));
} // 老师都不理解why排序。Topo-sort不要尝试去理解,算法内容
List<PartiallyComparableAdvisorHolder> sorted = PartialOrder.sort(partiallyComparableAdvisors);
if (sorted != null) {
List<Advisor> result = new ArrayList<>(advisors.size());
for (PartiallyComparableAdvisorHolder pcAdvisor : sorted) {
result.add(pcAdvisor.getAdvisor());
}
return result;
} else {
return super.sortAdvisors(advisors);
}
}
}
1. AspectJProxyUtils
public abstract class AspectJProxyUtils {
public static boolean makeAdvisorChainAspectJCapableIfNecessary(List<Advisor> advisors) {
// Don't add advisors to an empty list; may indicate that proxying is just not required
if (!advisors.isEmpty()) {
boolean foundAspectJAdvice = false;
for (Advisor advisor : advisors) {
// Be careful not to get the Advice without a guard, as this might eagerly
// instantiate a non-singleton AspectJ aspect...
if (isAspectJAdvice(advisor)) {
foundAspectJAdvice = true;
break;
}
}
if (foundAspectJAdvice && !advisors.contains(ExposeInvocationInterceptor.ADVISOR)) {
// 1. 增加头部advisor_ExposeInvocationInterceptor,用于在任意chain_node处获取MethodInvocation
advisors.add(0, ExposeInvocationInterceptor.ADVISOR);
return true;
}
}
return false;
}
}
2. ExposeInvocationInterceptor
public final class ExposeInvocationInterceptor implements MethodInterceptor, PriorityOrdered, Serializable {
/**
* Singleton instance of this class.
*/
public static final ExposeInvocationInterceptor INSTANCE = new ExposeInvocationInterceptor();
/**
* Singleton advisor for this class. Use in preference to INSTANCE when using
* Spring AOP, as it prevents the need to create a new Advisor to wrap the instance.
*/
public static final Advisor ADVISOR = new DefaultPointcutAdvisor(INSTANCE) {
@Override
public String toString() {
return ExposeInvocationInterceptor.class.getName() + ".ADVISOR";
}
};
}
3. sortAdvisors()
- 数据结构---拓扑排序详解
- 拓扑排序,有向无环图。不需要研究
- 排序前
- ExposeInvocationInterceptor
- AspectJAroundAdvice
- AspectJMethodBeforeAdvice
- AspectJAfterAdvice
- AspectJAfterReturningAdvice
- AspectJAfterThrowingAdvice
- 排序后
- ExposeInvocationInterceptor
- AspectJAfterThrowingAdvice
- AspectJAfterReturningAdvice
- AspectJAfterAdvice
- AspectJAroundAdvice
- AspectJMethodBeforeAdvice
3. createProxy()
#4. createProxy()
proxyFactory.setTargetSource(targetSource);
原bean传入ProxyFactory
父类AdvisedSupport
。1. advised_chain- 将 ProxyFactory 传入
ObjenesisCglibAopProxy
父类CglibAopProxy.adviced
public abstract class AbstractAutoProxyCreator extends ProxyProcessorSupport
implements SmartInstantiationAwareBeanPostProcessor, BeanFactoryAware {
/**
* Default is global AdvisorAdapterRegistry.
*/
private AdvisorAdapterRegistry advisorAdapterRegistry = GlobalAdvisorAdapterRegistry.getInstance();
protected Object createProxy(Class<?> beanClass, @Nullable String beanName,
@Nullable Object[] specificInterceptors, TargetSource targetSource) {
// 给bean定义设置暴露属性
if (this.beanFactory instanceof ConfigurableListableBeanFactory) { // 设置originalTargetClass属性
AutoProxyUtils.exposeTargetClass((ConfigurableListableBeanFactory) this.beanFactory, beanName, beanClass);
}
// 1. 核心类
ProxyFactory proxyFactory = new ProxyFactory();
// 获取当前类中相关属性
proxyFactory.copyFrom(this);
// 2. 判断使用targetClass而不是接口代理,检查proxyTargetClass、preserverTargetClass
if (!proxyFactory.isProxyTargetClass()) {
// jdk_proxy、cglib_proxy
if (shouldProxyTargetClass(beanClass, beanName)) {
// <aop:config proxy-target-class=""> 采用jdk、cglib代理
proxyFactory.setProxyTargetClass(true);
} else {
// 添加代理接口
evaluateProxyInterfaces(beanClass, proxyFactory);
}
}
// 3.1.. 封装 Interceptors => Advisor[]
Advisor[] advisors = buildAdvisors(beanName, specificInterceptors);
// 3.2.
proxyFactory.addAdvisors(advisors);
// 4. 设置到要代理的类
proxyFactory.setTargetSource(targetSource);
// 定制代理
customizeProxyFactory(proxyFactory);
// 控制代理工程被配置之后,是否还允许修改通知,默认值是false
proxyFactory.setFrozen(this.freezeProxy);
if (advisorsPreFiltered()) {
proxyFactory.setPreFiltered(true);
}
// 5. 创建代理对象
return proxyFactory.getProxy(getProxyClassLoader());
}
protected Advisor[] buildAdvisors(@Nullable String beanName, @Nullable Object[] specificInterceptors) {
// Handle prototypes correctly...
// 解析注册的所有interceptorName
Advisor[] commonInterceptors = resolveInterceptorNames();
List<Object> allInterceptors = new ArrayList<>();
if (specificInterceptors != null) {
// 加入拦截器
allInterceptors.addAll(Arrays.asList(specificInterceptors));
if (commonInterceptors.length > 0) {
if (this.applyCommonInterceptorsFirst) {
allInterceptors.addAll(0, Arrays.asList(commonInterceptors));
} else {
allInterceptors.addAll(Arrays.asList(commonInterceptors));
}
}
}
if (logger.isTraceEnabled()) {
int nrOfCommonInterceptors = commonInterceptors.length;
int nrOfSpecificInterceptors = (specificInterceptors != null ? specificInterceptors.length : 0);
logger.trace("Creating implicit proxy for bean '" + beanName + "' with " + nrOfCommonInterceptors +
" common interceptors and " + nrOfSpecificInterceptors + " specific interceptors");
}
Advisor[] advisors = new Advisor[allInterceptors.size()];
for (int i = 0; i < allInterceptors.size(); i++) {
// 1... DefaultAdvisorAdapterRegistry 拦截器进行封装转化为Advisor
advisors[i] = this.advisorAdapterRegistry.wrap(allInterceptors.get(i));
}
return advisors;
}
}
1. GlobalAdvisorAdapterRegistry
public final class GlobalAdvisorAdapterRegistry {
private GlobalAdvisorAdapterRegistry() {
}
/**
* 单例模式的使用,使用静态类变量来保持一个唯一实例
* <p>
* Keep track of a single instance so we can return it to classes that request it.
*/
private static AdvisorAdapterRegistry instance = new DefaultAdvisorAdapterRegistry();
/**
* 返回单例对象
* <p>
* Return the singleton {@link DefaultAdvisorAdapterRegistry} instance.
*/
public static AdvisorAdapterRegistry getInstance() {
return instance;
}
/**
* Reset the singleton {@link DefaultAdvisorAdapterRegistry}, removing any
* {@link AdvisorAdapterRegistry#registerAdvisorAdapter(AdvisorAdapter) registered}
* adapters.
*/
static void reset() {
instance = new DefaultAdvisorAdapterRegistry();
}
}
1. DefaultAdvisorAdapterRegistry
public class DefaultAdvisorAdapterRegistry implements AdvisorAdapterRegistry, Serializable {
private final List<AdvisorAdapter> adapters = new ArrayList<>(3);
// 新增AdvisorAdapter
public DefaultAdvisorAdapterRegistry() {
registerAdvisorAdapter(new MethodBeforeAdviceAdapter());
registerAdvisorAdapter(new AfterReturningAdviceAdapter());
registerAdvisorAdapter(new ThrowsAdviceAdapter());
}
@Override
public Advisor wrap(Object adviceObject) throws UnknownAdviceTypeException {
// 如果要封装的对象本身就是Advisor类型,那么无须做任何处理
if (adviceObject instanceof Advisor) {
return (Advisor) adviceObject;
}
// 如果类型不是Advisor和Advice两种类型的数据,那么将不能进行封装
if (!(adviceObject instanceof Advice)) {
throw new UnknownAdviceTypeException(adviceObject);
}
Advice advice = (Advice) adviceObject;
if (advice instanceof MethodInterceptor) {
// So well-known it doesn't even need an adapter.
// 如果是MethodInterceptor类型则使用DefaultPointcutAdvisor封装
return new DefaultPointcutAdvisor(advice);
}
// 如果存在Advisor的适配器那么也同样需要进行封装
for (AdvisorAdapter adapter : this.adapters) {
// Check that it is supported.
if (adapter.supportsAdvice(advice)) {
return new DefaultPointcutAdvisor(advice);
}
}
throw new UnknownAdviceTypeException(advice);
}
@Override
public void registerAdvisorAdapter(AdvisorAdapter adapter) {
this.adapters.add(adapter);
}
// ---------------------------------------------------------------------------
// 将Advice转换为MethodInterceptor[]
@Override
public MethodInterceptor[] getInterceptors(Advisor advisor) throws UnknownAdviceTypeException {
List<MethodInterceptor> interceptors = new ArrayList<>(3);
// 1.
Advice advice = advisor.getAdvice();
// 2. AspectJAroundAdvice, AspectJAfterThrowingAdvice, AspectJAfterAdvice
if (advice instanceof MethodInterceptor) {
interceptors.add((MethodInterceptor) advice);
}
for (AdvisorAdapter adapter : this.adapters) {
if (adapter.supportsAdvice(advice)) {
// 3. AspectJAfterReturningAdvice => AfterReturningAdviceInterceptor
// AspectJMethodBeforeAdvice => MethodBeforeAdviceInterceptor
interceptors.add(adapter.getInterceptor(advisor));
}
}
if (interceptors.isEmpty()) {
throw new UnknownAdviceTypeException(advisor.getAdvice());
}
return interceptors.toArray(new MethodInterceptor[0]);
}
}
2. AdviceAdapter
- 一切都是为了扩展使用
- 原本所有advice可以都实现MethodInterceptor接口,但是为了提高扩展性,还提供适配器模式。进行MethodInterceptor组装需要多加额外的判断逻辑,不能添加两次
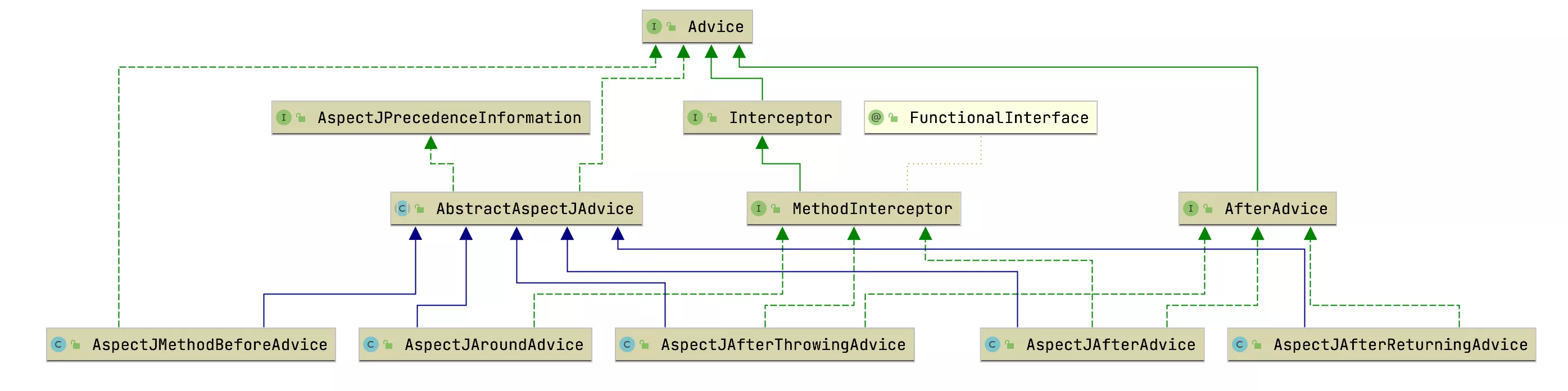
class AspectJAfterAdvice implements AfterAdvice
class AspectJAfterReturningAdvice implements AfterAdvice
class AspectJAfterThrowingAdvice implements AfterAdvice
// Advice implements MethodINterceptor
class AspectJAroundAdvice implements MethodInterceptor
class AspectJAfterThrowingAdvice implements MethodInterceptor
class AspectJAfterAdvice implements MethodInterceptor
// AdviceAdapter转换
class MethodBeforeAdviceInterceptor implements MethodInterceptor // MethodBeforeAdvice
class AfterReturningAdviceInterceptor implements MethodInterceptor // AfterReturningAdvice
class MethodBeforeAdviceAdapter implements AdvisorAdapter, Serializable {
@Override
public boolean supportsAdvice(Advice advice) {
return (advice instanceof MethodBeforeAdvice);
}
@Override
public MethodInterceptor getInterceptor(Advisor advisor) {
MethodBeforeAdvice advice = (MethodBeforeAdvice) advisor.getAdvice();
return new MethodBeforeAdviceInterceptor(advice);
}
}
class AfterReturningAdviceAdapter implements AdvisorAdapter, Serializable {
@Override
public boolean supportsAdvice(Advice advice) {
return (advice instanceof AfterReturningAdvice);
}
@Override
public MethodInterceptor getInterceptor(Advisor advisor) {
AfterReturningAdvice advice = (AfterReturningAdvice) advisor.getAdvice();
return new AfterReturningAdviceInterceptor(advice);
}
}
class ThrowsAdviceAdapter implements AdvisorAdapter, Serializable {
// `AspectJAfterThrowingAdvice implements AfterAdvice`,不属于ThrowsAdvice
@Override
public boolean supportsAdvice(Advice advice) {
return (advice instanceof ThrowsAdvice);
}
@Override
public MethodInterceptor getInterceptor(Advisor advisor) {
return new ThrowsAdviceInterceptor(advisor.getAdvice());
}
}
2. ProxyFactory
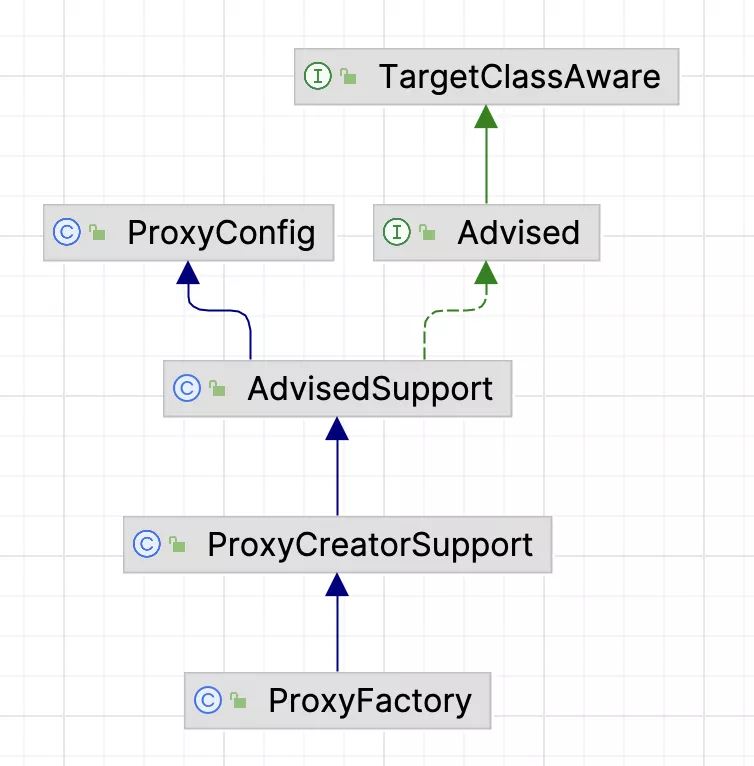
- advice:具体的某一个消息通知
- advisor:通知器包含advice和pointcut
- adviced:用来配置代理,指代ProxyFactory
public class ProxyFactory extends ProxyCreatorSupport {
public Object getProxy(@Nullable ClassLoader classLoader) {
/*
* 1... ProxyCreatorSupport createAopProxy() => 创建代理工厂,ObjenesisCglibAopProxy
* 2... CglibAopProxy getProxy() => 创建代理对象
*/
return createAopProxy().getProxy(classLoader);
}
}
1. AdvisedSupport
public class AdvisedSupport extends ProxyConfig implements Advised {
private transient Map<MethodCacheKey, List<Object>> methodCache;
private List<Advisor> advisors = new ArrayList<>();
/**
* Package-protected to allow direct access for efficiency.
*/
TargetSource targetSource = EMPTY_TARGET_SOURCE;
/**
* The AdvisorChainFactory to use.
*/
AdvisorChainFactory advisorChainFactory = new DefaultAdvisorChainFactory();
public List<Object> getInterceptorsAndDynamicInterceptionAdvice(Method method, @Nullable Class<?> targetClass) {
// 1. methodCache进行interceptor_cache
MethodCacheKey cacheKey = new MethodCacheKey(method);
List<Object> cached = this.methodCache.get(cacheKey);
if (cached == null) {
// 2. `DefaultAdvisorChainFactory` 筛选interceptor
cached = this.advisorChainFactory.getInterceptorsAndDynamicInterceptionAdvice(
this, method, targetClass);
// 3. 缓存添加
this.methodCache.put(cacheKey, cached);
}
return cached;
}
}
1. DefaultAdvisorChainFactory
- Advised => Advisor => PointcutAdvisor
ClassFilter, MethodMatcher
进行类和方法的匹配- DefaultAdvisorAdapterRegistry
public class DefaultAdvisorChainFactory implements AdvisorChainFactory, Serializable {
@Override
public List<Object> getInterceptorsAndDynamicInterceptionAdvice(
Advised config, Method method, @Nullable Class<?> targetClass) {
// This is somewhat tricky... We have to process introductions first,
// but we need to preserve order in the ultimate list.
// ** 单例,DefaultAdvisorAdapterRegistry。Spring把每个功能分的很细,相应类去处理,符合单一职责原则
// 1. DefaultAdvisorAdapterRegistry 将Advisor => MethodInterceptor
AdvisorAdapterRegistry registry = GlobalAdvisorAdapterRegistry.getInstance();
Advisor[] advisors = config.getAdvisors();
List<Object> interceptorList = new ArrayList<>(advisors.length);
Class<?> actualClass = (targetClass != null ? targetClass : method.getDeclaringClass());
Boolean hasIntroductions = null;
for (Advisor advisor : advisors) {
if (advisor instanceof PointcutAdvisor) {
// Add it conditionally.
PointcutAdvisor pointcutAdvisor = (PointcutAdvisor) advisor;
// 2. ClassFilter
if (config.isPreFiltered() || pointcutAdvisor.getPointcut().getClassFilter().matches(actualClass)) {
// 3.1.
MethodMatcher mm = pointcutAdvisor.getPointcut().getMethodMatcher();
boolean match;
if (mm instanceof IntroductionAwareMethodMatcher) {
if (hasIntroductions == null) {
hasIntroductions = hasMatchingIntroductions(advisors, actualClass);
}
// 3.2 MethodMatcher#matches()匹配目标方法
match = ((IntroductionAwareMethodMatcher) mm).matches(method, actualClass, hasIntroductions);
}
// TrueMethodMatcher <= ExposeInvocationInterceptor
else {
match = mm.matches(method, actualClass);
}
if (match) {
// 4... DefaultAdvisorAdapterRegistry advisor => MethodInterceptor[]
MethodInterceptor[] interceptors = registry.getInterceptors(advisor);
if (mm.isRuntime()) {
// Creating a new object instance in the getInterceptors() method
// isn't a problem as we normally cache created chains.
for (MethodInterceptor interceptor : interceptors) { // 动态切入点
interceptorList.add(new InterceptorAndDynamicMethodMatcher(interceptor, mm));
}
}
// 5. MethodInterceptor[]添加到interceptorList
else {
interceptorList.addAll(Arrays.asList(interceptors));
}
}
}
}
// 引介增强
else if (advisor instanceof IntroductionAdvisor) {
IntroductionAdvisor ia = (IntroductionAdvisor) advisor;
if (config.isPreFiltered() || ia.getClassFilter().matches(actualClass)) {
// Advisor转换为Interceptor
Interceptor[] interceptors = registry.getInterceptors(advisor);
interceptorList.addAll(Arrays.asList(interceptors));
}
}
// 以上两种都不是
else {
// 将Advisor转换为Interceptor
Interceptor[] interceptors = registry.getInterceptors(advisor);
interceptorList.addAll(Arrays.asList(interceptors));
}
}
return interceptorList;
}
}
1. MethodMatchers
public abstract class MethodMatchers {
@SuppressWarnings("serial")
private static class IntersectionIntroductionAwareMethodMatcher extends IntersectionMethodMatcher
implements IntroductionAwareMethodMatcher {
public IntersectionIntroductionAwareMethodMatcher(MethodMatcher mm1, MethodMatcher mm2) {
super(mm1, mm2);
}
@Override
public boolean matches(Method method, Class<?> targetClass, boolean hasIntroductions) {
// 99. LogUtil#around(), MyCalc#add() 是否相等
return (MethodMatchers.matches(this.mm1, method, targetClass, hasIntroductions) &&
MethodMatchers.matches(this.mm2, method, targetClass, hasIntroductions));
}
}
}
2. ProxyCreatorSupport
public class ProxyCreatorSupport extends AdvisedSupport {
private AopProxyFactory aopProxyFactory;
public ProxyCreatorSupport() {
// 1.
this.aopProxyFactory = new DefaultAopProxyFactory();
}
public AopProxyFactory getAopProxyFactory() {
return this.aopProxyFactory;
}
protected final synchronized AopProxy createAopProxy() {
if (!this.active) {
// 监听调用AdvisedSupportListener实现类的activated方法
activate();
}
/*
* 1.. getAopProxyFactory() => DefaultAopProxyFactory
* 2... createAopProxy() => ObjenesisCglibAopProxy
*/
return getAopProxyFactory().createAopProxy(this);
}
}
1. DefaultAopProxyFactory
public class DefaultAopProxyFactory implements AopProxyFactory, Serializable {
public AopProxy createAopProxy(AdvisedSupport config) throws AopConfigException {
if (config.isOptimize() || config.isProxyTargetClass() || hasNoUserSuppliedProxyInterfaces(config)) {
Class<?> targetClass = config.getTargetClass();
if (targetClass == null) {
throw new AopConfigException("TargetSource cannot determine target class: " +
"Either an interface or a target is required for proxy creation.");
}
if (targetClass.isInterface() || Proxy.isProxyClass(targetClass)) {
return new JdkDynamicAopProxy(config);
}
// 1. Cglib动态代理
return new ObjenesisCglibAopProxy(config);
} else {
// JDK动态代理
return new JdkDynamicAopProxy(config);
}
}
}
3. ObjenesisCglibAopProxy
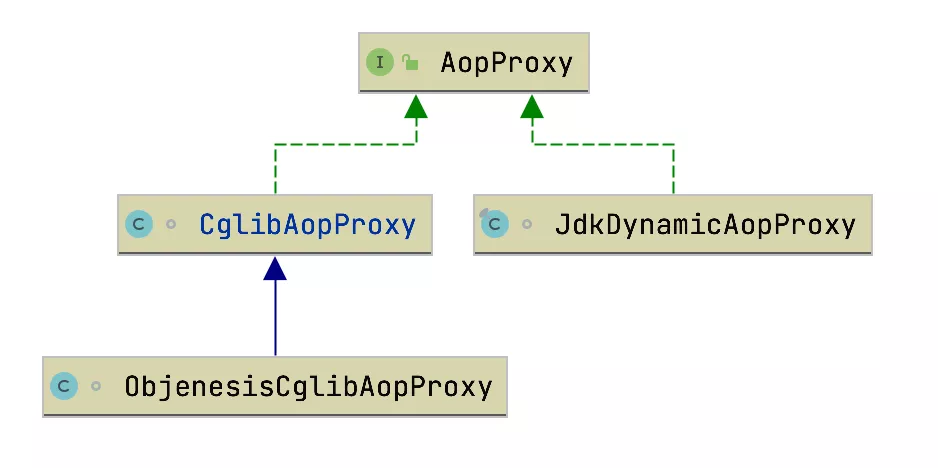
CglibAopProxy()
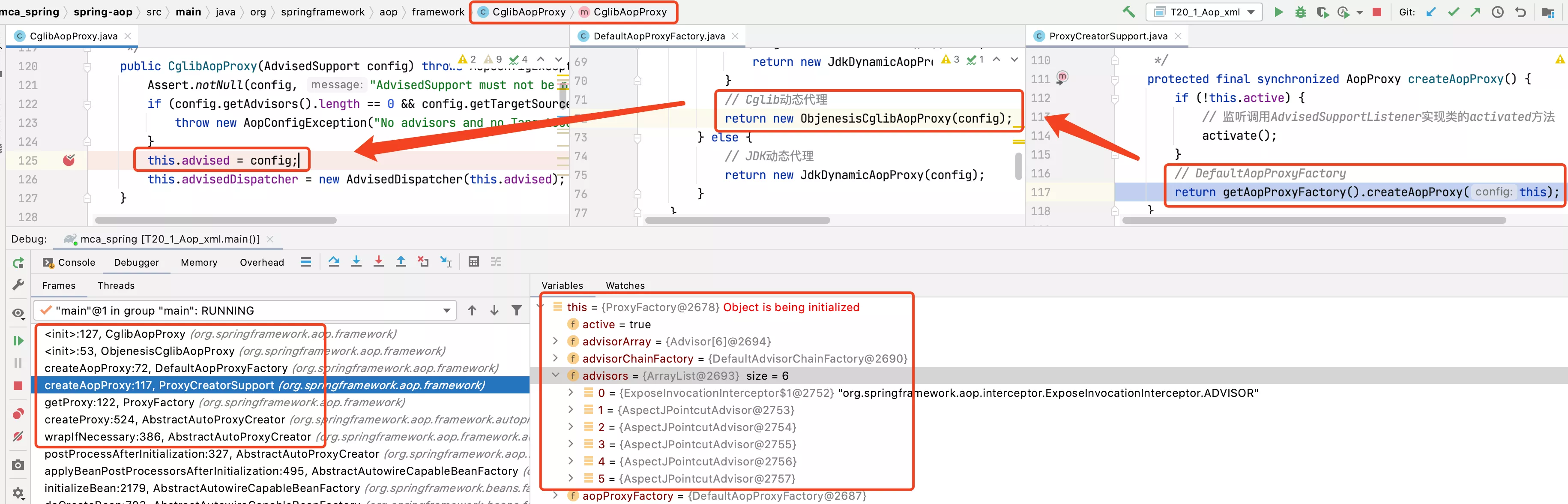
enhancer.createClass();
创建代理类setCallbacks()
class ObjenesisCglibAopProxy extends CglibAopProxy {
private static final SpringObjenesis objenesis = new SpringObjenesis();
public ObjenesisCglibAopProxy(AdvisedSupport config) {
super(config);
}
protected Object createProxyClassAndInstance(Enhancer enhancer, Callback[] callbacks) {
// 1. 生成proxy_Class
Class<?> proxyClass = enhancer.createClass();
Object proxyInstance = null;
if (objenesis.isWorthTrying()) {
try {
// 2. 反射实例化
proxyInstance = objenesis.newInstance(proxyClass, enhancer.getUseCache());
} catch (Throwable ex) {
logger.debug("Unable to instantiate proxy using Objenesis, " +
"falling back to regular proxy construction", ex);
}
}
if (proxyInstance == null) {
// Regular instantiation via default constructor...
try {
Constructor<?> ctor = (this.constructorArgs != null ?
proxyClass.getDeclaredConstructor(this.constructorArgTypes) :
proxyClass.getDeclaredConstructor());
ReflectionUtils.makeAccessible(ctor);
proxyInstance = (this.constructorArgs != null ?
ctor.newInstance(this.constructorArgs) : ctor.newInstance());
} catch (Throwable ex) {
throw new AopConfigException("Unable to instantiate proxy using Objenesis, " +
"and regular proxy instantiation via default constructor fails as well", ex);
}
}
// 3. setCallbacks()
((Factory) proxyInstance).setCallbacks(callbacks);
return proxyInstance;
}
}
1. CglibAopProxy
class CglibAopProxy implements AopProxy, Serializable {
// ProxyFactory
protected final AdvisedSupport advised;
public CglibAopProxy(AdvisedSupport config) throws AopConfigException {
if (config.getAdvisors().length == 0 && config.getTargetSource() == AdvisedSupport.EMPTY_TARGET_SOURCE) {
throw new AopConfigException("No advisors and no TargetSource specified");
}
this.advised = config;
this.advisedDispatcher = new AdvisedDispatcher(this.advised);
}
protected Enhancer createEnhancer() {
return new Enhancer();
}
// -----------------------------------------------------------------------------
public Object getProxy(@Nullable ClassLoader classLoader) {
// 0. MyCalc.class
Class<?> rootClass = this.advised.getTargetClass();
Class<?> proxySuperClass = rootClass;
// rootClass已经是代理对象,SuperClass来处理
if (rootClass.getName().contains(ClassUtils.CGLIB_CLASS_SEPARATOR)) { // $$
proxySuperClass = rootClass.getSuperclass();
// 获取原始父类的接口
Class<?>[] additionalInterfaces = rootClass.getInterfaces();
for (Class<?> additionalInterface : additionalInterfaces) {
this.advised.addInterface(additionalInterface);
}
}
// Validate the class, writing log messages as necessary.
// log不能代理的method_name。CGLIB继承来实现,所以final, static不能被增强
validateClassIfNecessary(proxySuperClass, classLoader);
// 1.. Configure CGLIB Enhancer...
Enhancer enhancer = createEnhancer();
if (classLoader != null) {
enhancer.setClassLoader(classLoader);
if (classLoader instanceof SmartClassLoader &&
((SmartClassLoader) classLoader).isClassReloadable(proxySuperClass)) {
enhancer.setUseCache(false);
}
}
// 2. 配置超类,代理类实现的接口,回调方法等
enhancer.setSuperclass(proxySuperClass);
// 增加SpringProxy, Advised接口,目前没用
enhancer.setInterfaces(AopProxyUtils.completeProxiedInterfaces(this.advised));
// 3. 命名规则替换,ByCGLIB => BySpringCGLIB
enhancer.setNamingPolicy(SpringNamingPolicy.INSTANCE);
enhancer.setStrategy(new ClassLoaderAwareGeneratorStrategy(classLoader));
// 4.. 获取所有回调函数(6个)
Callback[] callbacks = getCallbacks(rootClass);
Class<?>[] types = new Class<?>[callbacks.length];
for (int x = 0; x < types.length; x++) {
types[x] = callbacks[x].getClass();
}
// fixedInterceptorMap only populated at this point, after getCallbacks call above
enhancer.setCallbackFilter(new ProxyCallbackFilter(
this.advised.getConfigurationOnlyCopy(), this.fixedInterceptorMap, this.fixedInterceptorOffset));
enhancer.setCallbackTypes(types);
// Generate the proxy class and create a proxy instance.
// 5... ObjenesisCglibAopProxy Enhancer生成代理对象,并设置回调
return createProxyClassAndInstance(enhancer, callbacks);
}
private Callback[] getCallbacks(Class<?> rootClass) throws Exception {
// Parameters used for optimization choices...
// 1. expose-proxy属性处理,是否暴露当前对象为ThreadLocal,当前上下文中能够进行引用
boolean exposeProxy = this.advised.isExposeProxy();
boolean isFrozen = this.advised.isFrozen();
boolean isStatic = this.advised.getTargetSource().isStatic();
// Choose an "aop" interceptor (used for AOP calls).
// 2. ProxyFactory => DynamicAdvisedInterceptor.advised
Callback aopInterceptor = new DynamicAdvisedInterceptor(this.advised);
// Choose a "straight to target" interceptor. (used for calls that are
// unadvised but can return this). May be required to expose the proxy.
Callback targetInterceptor;
if (exposeProxy) {
targetInterceptor = (isStatic ?
new StaticUnadvisedExposedInterceptor(this.advised.getTargetSource().getTarget()) :
new DynamicUnadvisedExposedInterceptor(this.advised.getTargetSource()));
} else {
targetInterceptor = (isStatic ?
new StaticUnadvisedInterceptor(this.advised.getTargetSource().getTarget()) :
new DynamicUnadvisedInterceptor(this.advised.getTargetSource()));
}
// Choose a "direct to target" dispatcher (used for
// unadvised calls to static targets that cannot return this).
Callback targetDispatcher = (isStatic ?
new StaticDispatcher(this.advised.getTargetSource().getTarget()) : new SerializableNoOp());
Callback[] mainCallbacks = new Callback[]{
// 3. 拦截器链 => Callback
aopInterceptor, // for normal advice
targetInterceptor, // invoke target without considering advice, if optimized
new SerializableNoOp(), // no override for methods mapped to this
targetDispatcher, this.advisedDispatcher,
new EqualsInterceptor(this.advised),
new HashCodeInterceptor(this.advised)
};
Callback[] callbacks;
// If the target is a static one and the advice chain is frozen,
// then we can make some optimizations by sending the AOP calls
// direct to the target using the fixed chain for that method.
if (isStatic && isFrozen) {
Method[] methods = rootClass.getMethods();
Callback[] fixedCallbacks = new Callback[methods.length];
this.fixedInterceptorMap = new HashMap<>(methods.length);
// TODO: small memory optimization here (can skip creation for methods with no advice)
for (int x = 0; x < methods.length; x++) {
Method method = methods[x];
List<Object> chain = this.advised.getInterceptorsAndDynamicInterceptionAdvice(method, rootClass);
fixedCallbacks[x] = new FixedChainStaticTargetInterceptor(
chain, this.advised.getTargetSource().getTarget(), this.advised.getTargetClass());
this.fixedInterceptorMap.put(method, x);
}
// Now copy both the callbacks from mainCallbacks
// and fixedCallbacks into the callbacks array.
callbacks = new Callback[mainCallbacks.length + fixedCallbacks.length];
System.arraycopy(mainCallbacks, 0, callbacks, 0, mainCallbacks.length);
System.arraycopy(fixedCallbacks, 0, callbacks, mainCallbacks.length, fixedCallbacks.length);
this.fixedInterceptorOffset = mainCallbacks.length;
}
// 跳到这里
else {
callbacks = mainCallbacks;
}
return callbacks;
}
}
1. expose-proxy
<aop:aspectj-autoproxy expose-proxy="true"/>
- 某个代理类包含
m1()
和m2()
,当分别调用两个方法的时候,能否执行通知?肯定可以 - 如果
m1()
中调用m2()
,调用m2()
会执行通知吗?不会执行,如果想让他执行,就必须要设置expose-proxy="true"
2. Callback[]
// ProxyFactory => DynamicAdvisedInterceptor.advised
Callback aopInterceptor = new DynamicAdvisedInterceptor(this.advised);
- DynamicAdvisedInterceptor =>
new DynamicAdvisedInterceptor(this.advised);
- StaticUnadvisedInterceptor
- SerializableNoOp
- StaticDispatcher
- AdvisedDispatcher
- EqualsInterceptor
- HashCodeInterceptor
4. EnhancerBySpringCGLIB
Enhancer$EnhancerKey$$KeyFactoryByCGLIB$$4ce19e8f@2062
Enhancer#createHelper()
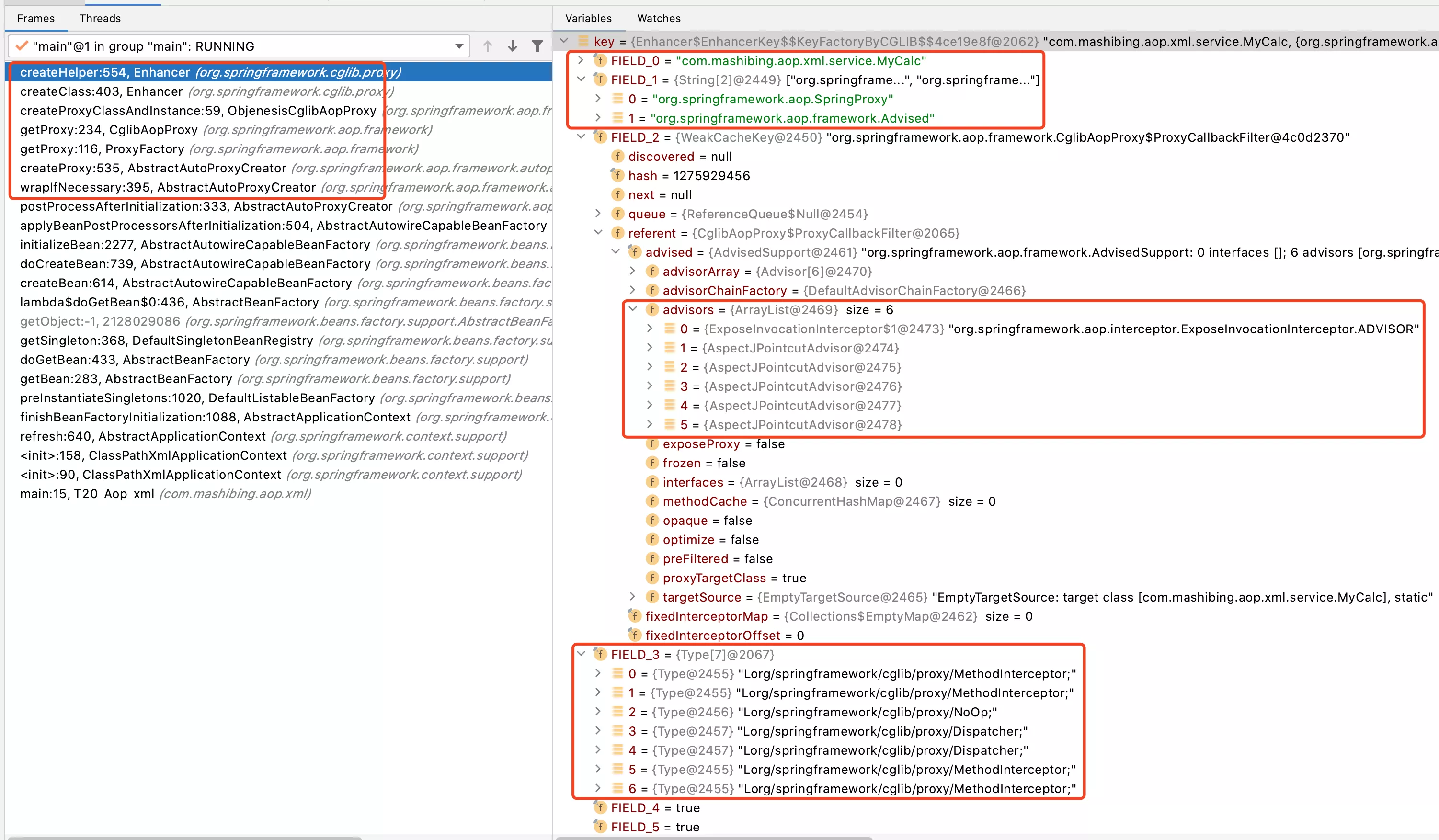
com.listao.aop.xml.service.MyCalc$$EnhancerBySpringCGLIB$$c3f9ed6c
Enhancer#generateClass()
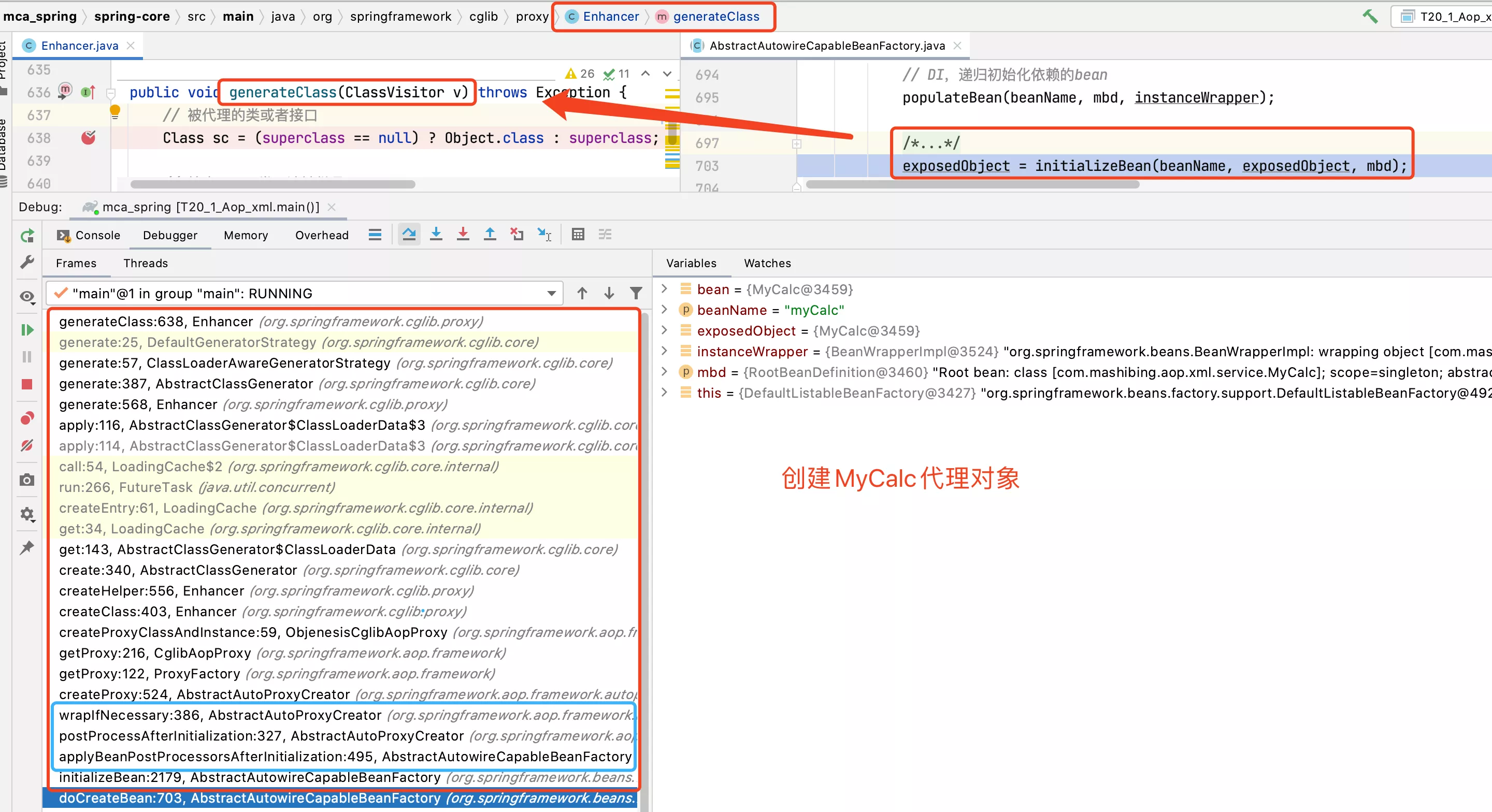
5. MyCalc#add()
- 代理对象中对保存原bean
- AbstractAutoProxyCreator 包装原对象
new SingletonTargetSource(bean)
- AbstractAutoProxyCreator =>
proxyFactory.setTargetSource(targetSource);
- DefaultAopProxyFactory =>
new ObjenesisCglibAopProxy(config);
- CglibAopProxy#getCallbacks() =>
Callback aopInterceptor = new DynamicAdvisedInterceptor(this.advised);
- DynamicAdvisedInterceptor =>
retVal = new CglibMethodInvocation(proxy, target, method, args, targetClass, chain, methodProxy).proceed();
- ReflectiveMethodInvocation.target
invokeJoinpoint()
=>return this.methodProxy.invoke(this.target, this.arguments);
- AbstractAutoProxyCreator 包装原对象
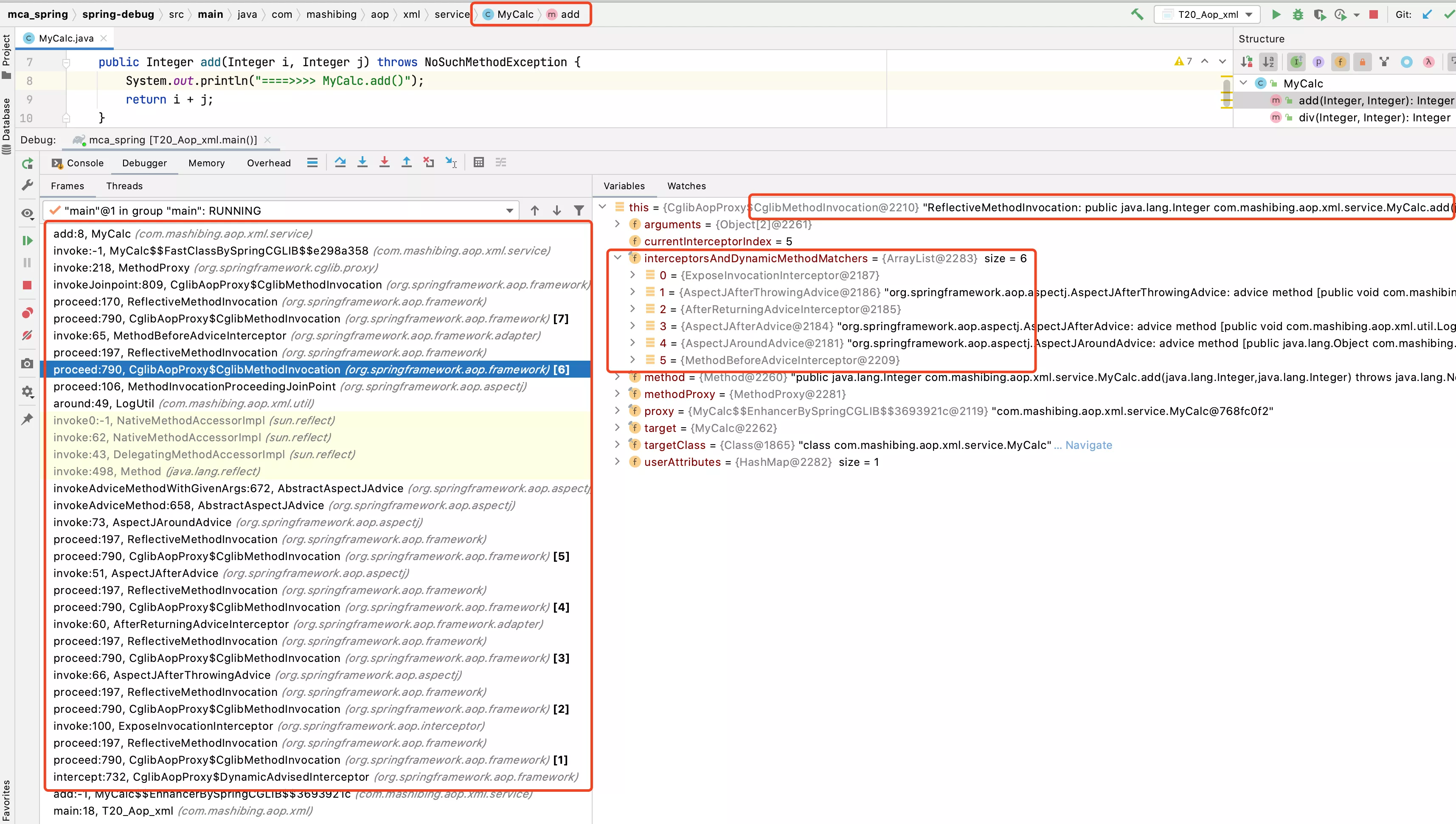
1. MyCalc$$EnhancerBySpringCGLIB
public class MyCalc$$EnhancerBySpringCGLIB$$ef4f5fa6 extends MyCalc implements SpringProxy, Advised, Factory {
private MethodInterceptor CGLIB$CALLBACK_0;
private static final Method CGLIB$add$3$Method;
private static final MethodProxy CGLIB$add$3$Proxy;
static {
CGLIB$STATICHOOK1();
}
static void CGLIB$STATICHOOK1() {
CGLIB$THREAD_CALLBACKS = new ThreadLocal();
CGLIB$emptyArgs = new Object[0];
// 1. 代理对象
Class var0 = Class.forName("com.listao.aop.xml.service.MyCalc$$EnhancerBySpringCGLIB$$ef4f5fa6");
// 2. 被代理对象
Class var1;
Method[] var10000 = //...
var10000 = ReflectUtils.findMethods(new String[]{
"mul", "(Ljava/lang/Integer;Ljava/lang/Integer;)Ljava/lang/Integer;",
"div", "(Ljava/lang/Integer;Ljava/lang/Integer;)Ljava/lang/Integer;",
"sub", "(Ljava/lang/Integer;Ljava/lang/Integer;)Ljava/lang/Integer;",
"add", "(Ljava/lang/Integer;Ljava/lang/Integer;)Ljava/lang/Integer;"},
(var1 = Class.forName("com.listao.aop.xml.service.MyCalc")).getDeclaredMethods());
// ...
// 3. 被代理方法
CGLIB$add$3$Method = var10000[3];
// 4. MethodProxy
CGLIB$add$3$Proxy = MethodProxy.create(var1, var0,
"(Ljava/lang/Integer;Ljava/lang/Integer;)Ljava/lang/Integer;", "add", "CGLIB$add$3");
}
final Integer CGLIB$add$3(Integer var1, Integer var2) throws NoSuchMethodException {
return super.add(var1, var2);
}
// 1. 代理方法
public final Integer add(Integer var1, Integer var2) throws NoSuchMethodException {
MethodInterceptor var10000 = this.CGLIB$CALLBACK_0;
if (var10000 == null) {
CGLIB$BIND_CALLBACKS(this);
var10000 = this.CGLIB$CALLBACK_0;
}
return var10000 != null ?
/**
* 4. 责任链执行
* @param proxy 代理对象
* @param method 被代理方法
* @param args 实参
* @param methodProxy methodProxy
*/
(Integer)var10000.intercept(this, CGLIB$add$3$Method, new Object[]{var1, var2}, CGLIB$add$3$Proxy) :
super.add(var1, var2);
}
public void setCallbacks(Callback[] var1) {
this.CGLIB$CALLBACK_0 = (MethodInterceptor)var1[0]; // 3... DynamicAdvisedInterceptor(Advised)
this.CGLIB$CALLBACK_1 = (MethodInterceptor)var1[1];
this.CGLIB$CALLBACK_2 = (NoOp)var1[2];
this.CGLIB$CALLBACK_3 = (Dispatcher)var1[3];
this.CGLIB$CALLBACK_4 = (Dispatcher)var1[4];
this.CGLIB$CALLBACK_5 = (MethodInterceptor)var1[5];
this.CGLIB$CALLBACK_6 = (MethodInterceptor)var1[6];
}
}
2. DynamicAdvisedInterceptor
class CglibAopProxy implements AopProxy, Serializable {
private static class DynamicAdvisedInterceptor implements MethodInterceptor, Serializable {
private final AdvisedSupport advised; // ProxyFactory
/**
* @param proxy 代理对象
* @param method 被代理方法
* @param args 实参
* @param methodProxy 方法代理
*/
public Object intercept(Object proxy, Method method, Object[] args, MethodProxy methodProxy) throws Throwable {
Object oldProxy = null;
boolean setProxyContext = false;
Object target = null;
// 1.1. MyCalc的bean对象
TargetSource targetSource = this.advised.getTargetSource();
try {
// 1.2. 两方法相互调用,是否都AOP
if (this.advised.exposeProxy) {
// Make invocation available if necessary.
oldProxy = AopContext.setCurrentProxy(proxy);
setProxyContext = true;
}
// Get as late as possible to minimize the time we "own" the target, in case it comes from a pool...
// 1.3. MyCalc_bean
target = targetSource.getTarget();
Class<?> targetClass = (target != null ? target.getClass() : null);
// 2... AdvisedSupport 从advised(ProxyFactory)中获取配置好的AOP通知
List<Object> chain = this.advised.getInterceptorsAndDynamicInterceptionAdvice(method, targetClass);
Object retVal;
// Check whether we only have one InvokerInterceptor: that is,
// no real advice, but just reflective invocation of the target.
// chain为空则直接调用原方法
if (chain.isEmpty() && Modifier.isPublic(method.getModifiers())) {
// We can skip creating a MethodInvocation: just invoke the target directly.
// Note that the final invoker must be an InvokerInterceptor, so we know
// it does nothing but a reflective operation on the target, and no hot
// swapping or fancy proxying.
Object[] argsToUse = AopProxyUtils.adaptArgumentsIfNecessary(method, args);
retVal = methodProxy.invoke(target, argsToUse);
} else {
// 3... `CglibAopProxy` We need to create a method invocation...)
retVal = new CglibMethodInvocation(proxy, target, method, args, targetClass, chain, methodProxy).proceed();
}
// 4. retureType处理
retVal = processReturnType(proxy, target, method, retVal);
return retVal;
} finally {
if (target != null && !targetSource.isStatic()) {
targetSource.releaseTarget(target);
}
if (setProxyContext) {
// Restore old proxy.
AopContext.setCurrentProxy(oldProxy);
}
}
}
}
private static class CglibMethodInvocation extends ReflectiveMethodInvocation {
// 被代理、代理类的FastClass封装
private final MethodProxy methodProxy;
/**
* @param proxy 代理对象
* @param target 被代理对象
* @param method 被代理方法
* @param arguments 实参
* @param targetClass 被代理Class
* @param interceptorsAndDynamicMethodMatchers chain
* @param methodProxy methodProxy
*/
public CglibMethodInvocation(Object proxy, @Nullable Object target, Method method,
Object[] arguments, @Nullable Class<?> targetClass,
List<Object> interceptorsAndDynamicMethodMatchers, MethodProxy methodProxy) {
super(proxy, target, method, arguments, targetClass, interceptorsAndDynamicMethodMatchers);
// Only use method proxy for public methods not derived from java.lang.Object
this.methodProxy = (Modifier.isPublic(method.getModifiers()) &&
method.getDeclaringClass() != Object.class && !AopUtils.isEqualsMethod(method) &&
!AopUtils.isHashCodeMethod(method) && !AopUtils.isToStringMethod(method) ?
methodProxy : null);
}
@Override
@Nullable
public Object proceed() throws Throwable {
try {
// 2. ReflectiveMethodInvocation
return super.proceed();
} catch (RuntimeException ex) {
throw ex;
} catch (Exception ex) {
if (ReflectionUtils.declaresException(getMethod(), ex.getClass())) {
throw ex;
} else {
throw new UndeclaredThrowableException(ex);
}
}
}
// ------------------------------------------------------------------------
// 调用连接点方法
@Override
protected Object invokeJoinpoint() throws Throwable {
if (this.methodProxy != null) {
// 3. invoke()被代理对象的FastClass。target被代理对象,arguments实参
return this.methodProxy.invoke(this.target, this.arguments);
} else {
return super.invokeJoinpoint();
}
}
}
}
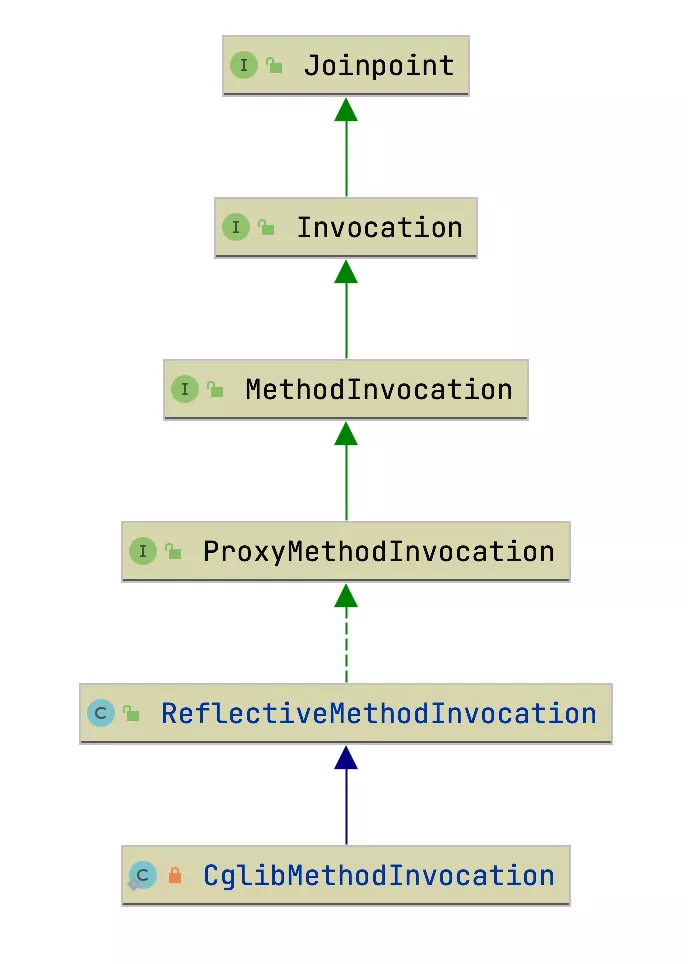
3. ReflectiveMethodInvocation
LogUtil#before()
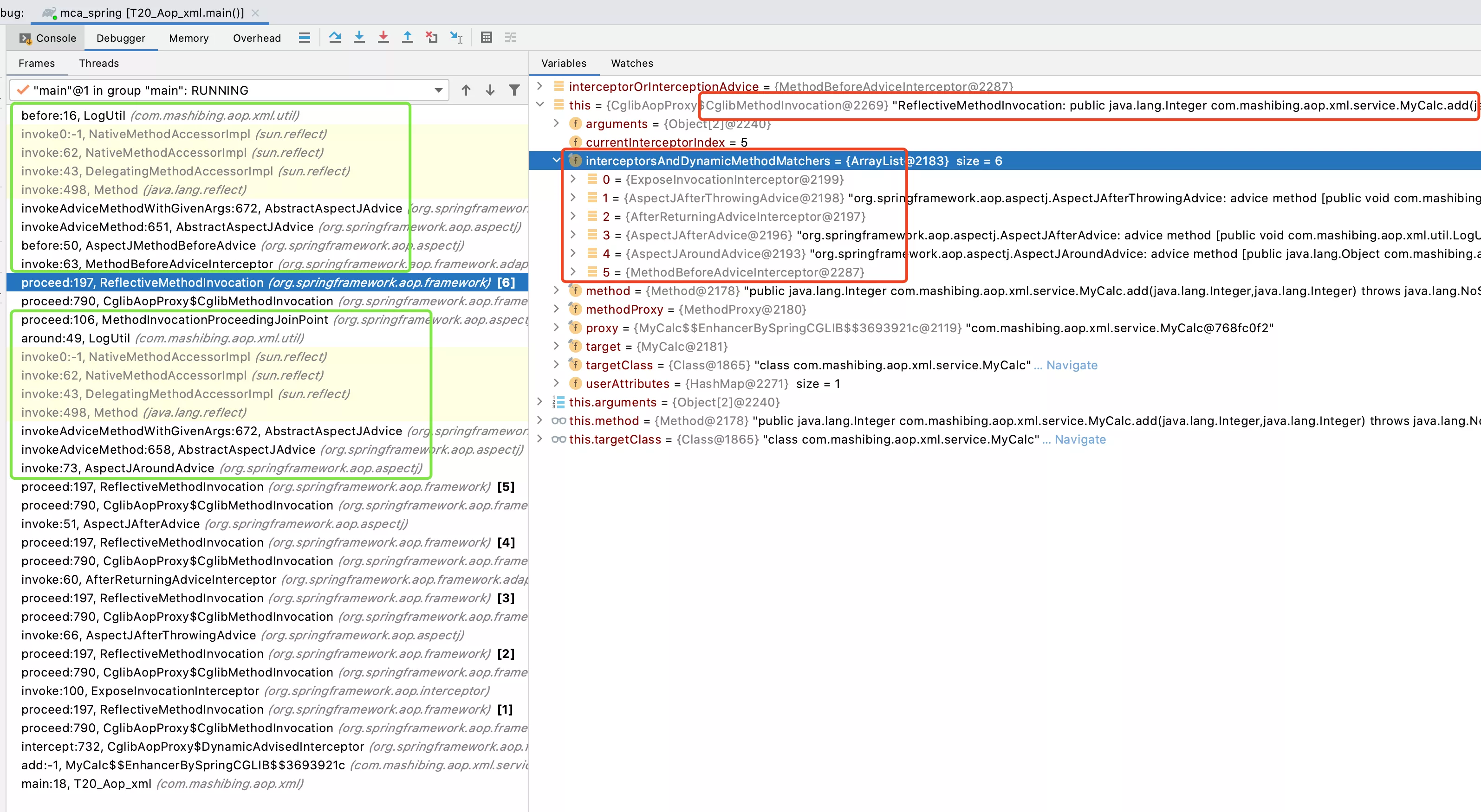
this.methodProxy.invoke(this.target, this.arguments);
MethodProxy直接调用原对象方法
public class ReflectiveMethodInvocation implements ProxyMethodInvocation, Cloneable {
protected final Object proxy;
private final Class<?> targetClass;
protected final Method method;
protected final Object target; // 原对象
protected Object[] arguments; // 实参
// chain
protected final List<?> interceptorsAndDynamicMethodMatchers;
private int currentInterceptorIndex = -1;
protected ReflectiveMethodInvocation(
Object proxy, @Nullable Object target, Method method, @Nullable Object[] arguments,
@Nullable Class<?> targetClass, List<Object> interceptorsAndDynamicMethodMatchers) {
this.proxy = proxy; // 代理对象
this.target = target; // 被代理对象
this.targetClass = targetClass; // 被代理Class
this.method = BridgeMethodResolver.findBridgedMethod(method); // 被代理方法
this.arguments = AopProxyUtils.adaptArgumentsIfNecessary(method, arguments); // 实参
this.interceptorsAndDynamicMethodMatchers = interceptorsAndDynamicMethodMatchers; // chain
}
@Override
@Nullable
public Object proceed() throws Throwable {
// We start with an index of -1 and increment early.
// 1... CglibMethodInvocation 调用原对象method
if (this.currentInterceptorIndex == this.interceptorsAndDynamicMethodMatchers.size() - 1) {
return invokeJoinpoint();
}
// 2. chain下一个node
Object interceptorOrInterceptionAdvice =
this.interceptorsAndDynamicMethodMatchers.get(++this.currentInterceptorIndex);
if (interceptorOrInterceptionAdvice instanceof InterceptorAndDynamicMethodMatcher) {
// Evaluate dynamic method matcher here: static part will already have
// been evaluated and found to match.
InterceptorAndDynamicMethodMatcher dm =
(InterceptorAndDynamicMethodMatcher) interceptorOrInterceptionAdvice;
Class<?> targetClass = (this.targetClass != null ? this.targetClass : this.method.getDeclaringClass());
if (dm.methodMatcher.matches(this.method, targetClass, this.arguments)) {
return dm.interceptor.invoke(this);
}
// 不匹配,那么proceed会被递归调用,直到所有的拦截器都被运行过
else {
// Dynamic matching failed.
// Skip this interceptor and invoke the next in the chain.
return proceed();
}
}
// 3. GenericInterceptor直接invoke(),执行advice
else {
// It's an interceptor, so we just invoke it: The pointcut will have
// been evaluated statically before this object was constructed.
return ((MethodInterceptor) interceptorOrInterceptionAdvice).invoke(this);
}
}
}
0. ExposeInvocationInterceptor
interceptorList
1. ExposeInvocationInterceptor
2. AspectJAfterThrowingAdvice
3. AfterReturningAdviceInterceptor // adepter生成
4. AspectJAfterAdvice
5. AspectJAroundAdvice
6. MethodBeforeAdviceInterceptor // adapter生成
public final class ExposeInvocationInterceptor implements MethodInterceptor, PriorityOrdered, Serializable {
private static final ThreadLocal<MethodInvocation> invocation = new NamedThreadLocal<>("Current AOP method invocation");
@Override
public Object invoke(MethodInvocation mi) throws Throwable {
MethodInvocation oldInvocation = invocation.get();
invocation.set(mi);
try {
// 1.
return mi.proceed();
} finally {
invocation.set(oldInvocation);
}
}
public static MethodInvocation currentInvocation() throws IllegalStateException {
MethodInvocation mi = invocation.get();
// ...
return mi;
}
}
1. AspectJAfterThrowingAdvice
public class AspectJAfterThrowingAdvice extends AbstractAspectJAdvice
implements MethodInterceptor, AfterAdvice, Serializable {
@Override
public Object invoke(MethodInvocation mi) throws Throwable {
try {
// 1.
return mi.proceed();
} catch (Throwable ex) {
if (shouldInvokeOnThrowing(ex)) {
// 2. 执行异常通知
invokeAdviceMethod(getJoinPointMatch(), null, ex);
}
throw ex;
}
}
}
2. AfterReturningAdviceInterceptor
public class AfterReturningAdviceInterceptor implements MethodInterceptor
, AfterAdvice, Serializable {
private final AfterReturningAdvice advice;
@Override
public Object invoke(MethodInvocation mi) throws Throwable {
// 1.
Object retVal = mi.proceed();
// 2... AspectJAfterReturningAdvice 返回通知方法执行
this.advice.afterReturning(retVal, mi.getMethod(), mi.getArguments(), mi.getThis());
return retVal;
}
}
1. AspectJAfterReturningAdvice
public class AspectJAfterReturningAdvice extends AbstractAspectJAdvice
implements AfterReturningAdvice, AfterAdvice, Serializable {
@Override
public void afterReturning(@Nullable Object returnValue, Method method, Object[] args, @Nullable Object target) throws Throwable {
if (shouldInvokeOnReturnValueOf(method, returnValue)) {
// 1.
invokeAdviceMethod(getJoinPointMatch(), returnValue, null);
}
}
}
3. AspectJAfterAdvice
- 后置通知总会执行,在
finally{}
中
public class AspectJAfterAdvice extends AbstractAspectJAdvice
implements MethodInterceptor, AfterAdvice, Serializable {
@Override
public Object invoke(MethodInvocation mi) throws Throwable {
try {
// 1.
return mi.proceed();
} finally {
// 2. 后置通知总会执行,在finally{}中
invokeAdviceMethod(getJoinPointMatch(), null, null);
}
}
}
4. AspectJAroundAdvice
public class AspectJAroundAdvice extends AbstractAspectJAdvice implements MethodInterceptor, Serializable {
@Override
public Object invoke(MethodInvocation mi) throws Throwable {
if (!(mi instanceof ProxyMethodInvocation)) {
throw new IllegalStateException("MethodInvocation is not a Spring ProxyMethodInvocation: " + mi);
}
ProxyMethodInvocation pmi = (ProxyMethodInvocation) mi;
// 1.. MethodInvocationProceedingJoinPoint
ProceedingJoinPoint pjp = lazyGetProceedingJoinPoint(pmi);
JoinPointMatch jpm = getJoinPointMatch(pmi);
// 2. 调用父类AbstractAspectJAdvice
return invokeAdviceMethod(pjp, jpm, null, null);
}
protected ProceedingJoinPoint lazyGetProceedingJoinPoint(ProxyMethodInvocation rmi) {
return new MethodInvocationProceedingJoinPoint(rmi);
}
}
1. LogUtil
/**
* ProceedingJoinPoint extends JoinPoint
* MethodInvocationProceedingJoinPoint implements ProceedingJoinPoint
*/
public Object around(ProceedingJoinPoint pjp) throws Throwable {
Signature signature = pjp.getSignature();
Object[] args = pjp.getArgs();
Object result;
try {
System.out.println("around() -> before, " + signature.getName() + "(), 参数为: " + Arrays.asList(args));
// 1. 反射调用目标方法,相当于执行method.invoke(),可以自己修改结果值
result = pjp.proceed(args);
// result = 100;
System.out.println("around() -> after, " + signature.getName() + "()结果: " + result);
} catch (Throwable throwable) {
System.out.println("around() -> throwable, " + signature.getName() + "()异常");
throw throwable;
}
return result;
}
2. MtdInvocationProceedingJoinPoint
public class MethodInvocationProceedingJoinPoint implements ProceedingJoinPoint, JoinPoint.StaticPart {
private final ProxyMethodInvocation methodInvocation;
public MethodInvocationProceedingJoinPoint(ProxyMethodInvocation methodInvocation) {
Assert.notNull(methodInvocation, "MethodInvocation must not be null");
this.methodInvocation = methodInvocation;
}
@Override
public Object proceed() throws Throwable {
return this.methodInvocation.invocableClone().proceed();
}
@Override
public Object proceed(Object[] arguments) throws Throwable {
Assert.notNull(arguments, "Argument array passed to proceed cannot be null");
if (arguments.length != this.methodInvocation.getArguments().length) {
throw new IllegalArgumentException("Expecting " +
this.methodInvocation.getArguments().length + " arguments to proceed, " +
"but was passed " + arguments.length + " arguments");
}
this.methodInvocation.setArguments(arguments);
// 1. clone新的CglibMethodInvocation
return this.methodInvocation.invocableClone(arguments).proceed();
}
}
public interface ProxyMethodInvocation extends MethodInvocation {
}
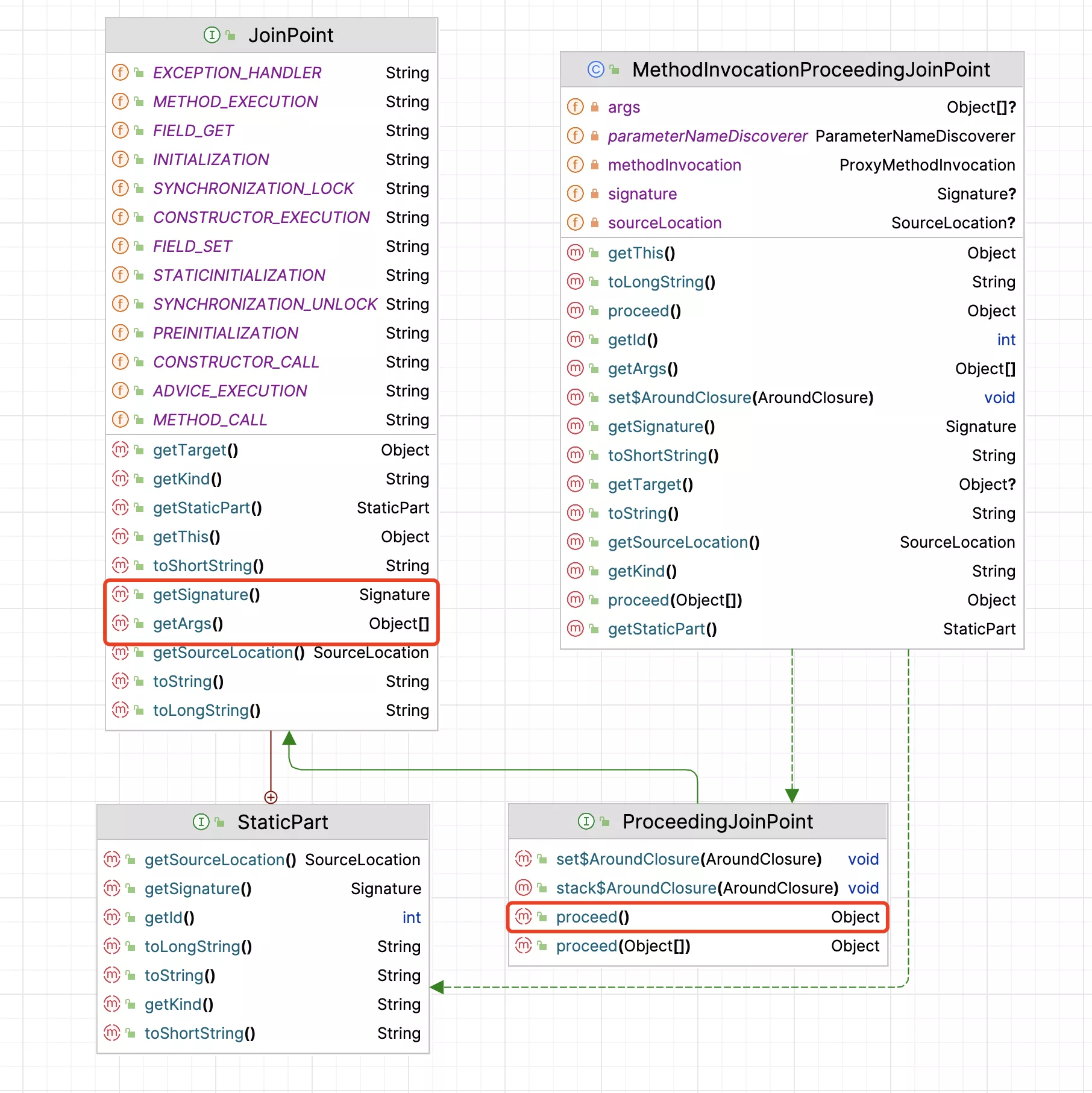
5. MethodBeforeAdviceInterceptor
public class MethodBeforeAdviceInterceptor implements MethodInterceptor, BeforeAdvice, Serializable {
private final MethodBeforeAdvice advice;
@Override
public Object invoke(MethodInvocation mi) throws Throwable {
// 1. 反射aspect.advice()
this.advice.before(mi.getMethod(), mi.getArguments(), mi.getThis());
// 2. callback
return mi.proceed();
}
}
1. AspectJMethodBeforeAdvice
public class AspectJMethodBeforeAdvice extends AbstractAspectJAdvice implements MethodBeforeAdvice, Serializable {
@Override
public void before(Method method, Object[] args, @Nullable Object target) throws Throwable {
// 1. 入参,目标对象、目标参数、目标方法都没有用到
invokeAdviceMethod(getJoinPointMatch(), null, null);
}
}
99. AbstractAspectJAdvice
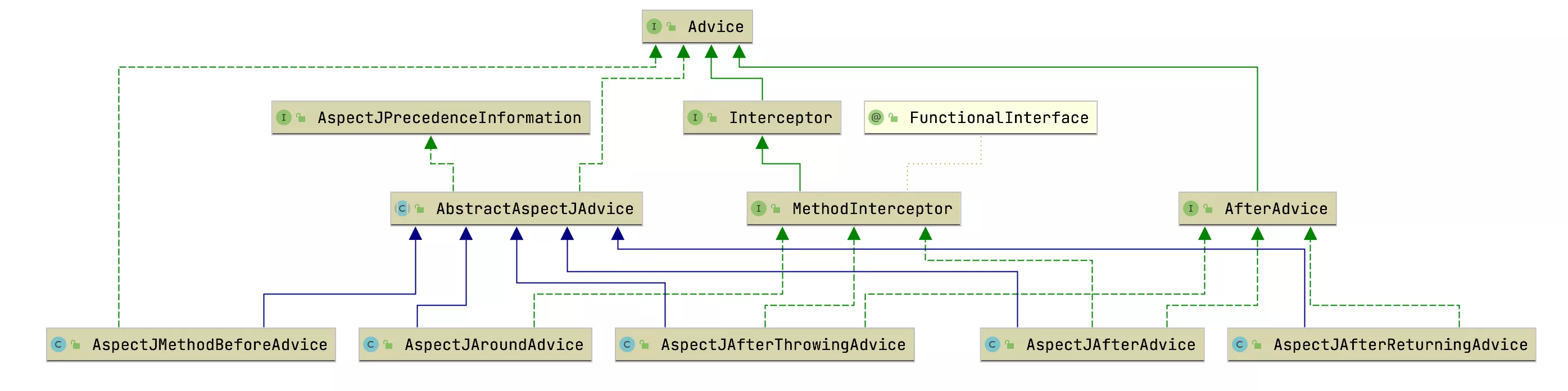
- 子类
AspectJ___Advice
调用父类AbstractAspectJAdvice.invokeAdviceMethodWithGivenArgs()
方法,反射aspect的advice
public abstract class AbstractAspectJAdvice implements Advice, AspectJPrecedenceInformation, Serializable {
protected transient Method aspectJAdviceMethod;
private final AspectInstanceFactory aspectInstanceFactory;
public AbstractAspectJAdvice(
Method aspectJAdviceMethod, AspectJExpressionPointcut pointcut, AspectInstanceFactory aspectInstanceFactory) {
Assert.notNull(aspectJAdviceMethod, "Advice method must not be null");
this.declaringClass = aspectJAdviceMethod.getDeclaringClass();
this.methodName = aspectJAdviceMethod.getName();
this.parameterTypes = aspectJAdviceMethod.getParameterTypes();
this.aspectJAdviceMethod = aspectJAdviceMethod;
this.pointcut = pointcut;
this.aspectInstanceFactory = aspectInstanceFactory;
}
/**
* 1. AspectJAroundAdvice调用
*/
// As above, but in this case we are given the join point.
protected Object invokeAdviceMethod(JoinPoint jp, @Nullable JoinPointMatch jpMatch,
@Nullable Object returnValue, @Nullable Throwable t) throws Throwable {
return invokeAdviceMethodWithGivenArgs(argBinding(jp, jpMatch, returnValue, t));
}
/**
* 3. AspectJMethod___Advice调用
*/
protected Object invokeAdviceMethod(
@Nullable JoinPointMatch jpMatch, @Nullable Object returnValue, @Nullable Throwable ex)
throws Throwable {
return invokeAdviceMethodWithGivenArgs(argBinding(getJoinPoint(), jpMatch, returnValue, ex));
}
// ---------------------------------------------------------------------------
/**
* 2. 反射aspect的advice核心方法
*/
protected Object invokeAdviceMethodWithGivenArgs(Object[] args) throws Throwable {
Object[] actualArgs = args;
// 是否无参
if (this.aspectJAdviceMethod.getParameterCount() == 0) {
actualArgs = null;
}
try {
ReflectionUtils.makeAccessible(this.aspectJAdviceMethod);
// TODO AopUtils.invokeJoinpointUsingReflection
// 99. 反射调用aspect.advice()
return this.aspectJAdviceMethod.invoke(this.aspectInstanceFactory.getAspectInstance(), actualArgs);
} catch (IllegalArgumentException ex) {
throw new AopInvocationException("Mismatch on arguments to advice method [" +
this.aspectJAdviceMethod + "]; pointcut expression [" +
this.pointcut.getPointcutExpression() + "]", ex);
} catch (InvocationTargetException ex) {
throw ex.getTargetException();
}
}
// ---------------------------------------------------------------------------
/**
* Overridden in around advice to return proceeding join point.
*/
protected JoinPoint getJoinPoint() {
return currentJoinPoint();
}
public static JoinPoint currentJoinPoint() {
MethodInvocation mi = ExposeInvocationInterceptor.currentInvocation();
if (!(mi instanceof ProxyMethodInvocation)) {
throw new IllegalStateException("MethodInvocation is not a Spring ProxyMethodInvocation: " + mi);
}
ProxyMethodInvocation pmi = (ProxyMethodInvocation) mi;
JoinPoint jp = (JoinPoint) pmi.getUserAttribute(JOIN_POINT_KEY);
if (jp == null) {
jp = new MethodInvocationProceedingJoinPoint(pmi);
pmi.setUserAttribute(JOIN_POINT_KEY, jp); // org.aspectj.lang.JoinPoint
}
return jp;
}
/**
* Get the current join point match at the join point we are being dispatched on.
*/
@Nullable
protected JoinPointMatch getJoinPointMatch() {
MethodInvocation mi = ExposeInvocationInterceptor.currentInvocation();
if (!(mi instanceof ProxyMethodInvocation)) {
throw new IllegalStateException("MethodInvocation is not a Spring ProxyMethodInvocation: " + mi);
}
// 这里主要是获取 JoinPointMatch
return getJoinPointMatch((ProxyMethodInvocation) mi);
}
@Nullable
protected JoinPointMatch getJoinPointMatch(ProxyMethodInvocation pmi) {
String expression = this.pointcut.getExpression();
return (expression != null ? (JoinPointMatch) pmi.getUserAttribute(expression) : null);
}
/**
* 参数绑定
* <p>
* Take the arguments at the method execution join point and output a set of arguments
* to the advice method.
*
* @param jp the current JoinPoint
* @param jpMatch the join point match that matched this execution join point
* @param returnValue the return value from the method execution (may be null)
* @param ex the exception thrown by the method execution (may be null)
* @return the empty array if there are no arguments
*/
protected Object[] argBinding(JoinPoint jp, @Nullable JoinPointMatch jpMatch,
@Nullable Object returnValue, @Nullable Throwable ex) {
calculateArgumentBindings();
// AMC start
Object[] adviceInvocationArgs = new Object[this.parameterTypes.length];
int numBound = 0;
// 这个默认值是 -1 重新赋值是在calculateArgumentBindings中进行的
// 1. round, before, after, afterReturning
if (this.joinPointArgumentIndex != -1) {
adviceInvocationArgs[this.joinPointArgumentIndex] = jp;
numBound++;
} else if (this.joinPointStaticPartArgumentIndex != -1) {
adviceInvocationArgs[this.joinPointStaticPartArgumentIndex] = jp.getStaticPart();
numBound++;
}
// 取通知方法中的参数类型 是除了 JoinPoint和ProceedingJoinPoint参数之外的参数
if (!CollectionUtils.isEmpty(this.argumentBindings)) {
// binding from pointcut match
if (jpMatch != null) {
PointcutParameter[] parameterBindings = jpMatch.getParameterBindings();
for (PointcutParameter parameter : parameterBindings) {
String name = parameter.getName();
Integer index = this.argumentBindings.get(name);
adviceInvocationArgs[index] = parameter.getBinding();
numBound++;
}
}
// binding from returning clause
// 2. 后置返回通知参数
if (this.returningName != null) {
Integer index = this.argumentBindings.get(this.returningName);
adviceInvocationArgs[index] = returnValue;
numBound++;
}
// binding from thrown exception
// 3. 异常通知参数
if (this.throwingName != null) {
Integer index = this.argumentBindings.get(this.throwingName);
adviceInvocationArgs[index] = ex;
numBound++;
}
}
if (numBound != this.parameterTypes.length) {
throw new IllegalStateException("Required to bind " + this.parameterTypes.length +
" arguments, but only bound " + numBound + " (JoinPointMatch " +
(jpMatch == null ? "was NOT" : "WAS") + " bound in invocation)");
}
return adviceInvocationArgs;
}
}
6. Anno_AOP
- 注解
add()
打断点,不能直接进入
public class T20_2_Aop_Anno {
public static void main(String[] args) throws NoSuchMethodException {
AnnotationConfigApplicationContext ac = new AnnotationConfigApplicationContext();
System.out.println("------------------- ac.over -------------------");
// 1.
ac.register(AopConfig.class);
ac.refresh();
MyCalc myCalc = ac.getBean("myCalc", MyCalc.class);
myCalc.add(1, 1);
ac.close();
}
}
1. AnnotationConfigAC
public class AnnotationConfigApplicationContext extends GenericApplicationContext implements AnnotationConfigRegistry {
private final AnnotatedBeanDefinitionReader reader;
private final ClassPathBeanDefinitionScanner scanner;
public AnnotationConfigApplicationContext() {
// 1.
this.reader = new AnnotatedBeanDefinitionReader(this);
// 2.
this.scanner = new ClassPathBeanDefinitionScanner(this);
}
@Override
public void register(Class<?>... componentClasses) {
Assert.notEmpty(componentClasses, "At least one component class must be specified");
// 1.
this.reader.register(componentClasses);
}
}
BeanDefinitionReaderUtils#registerBeanDefinition()
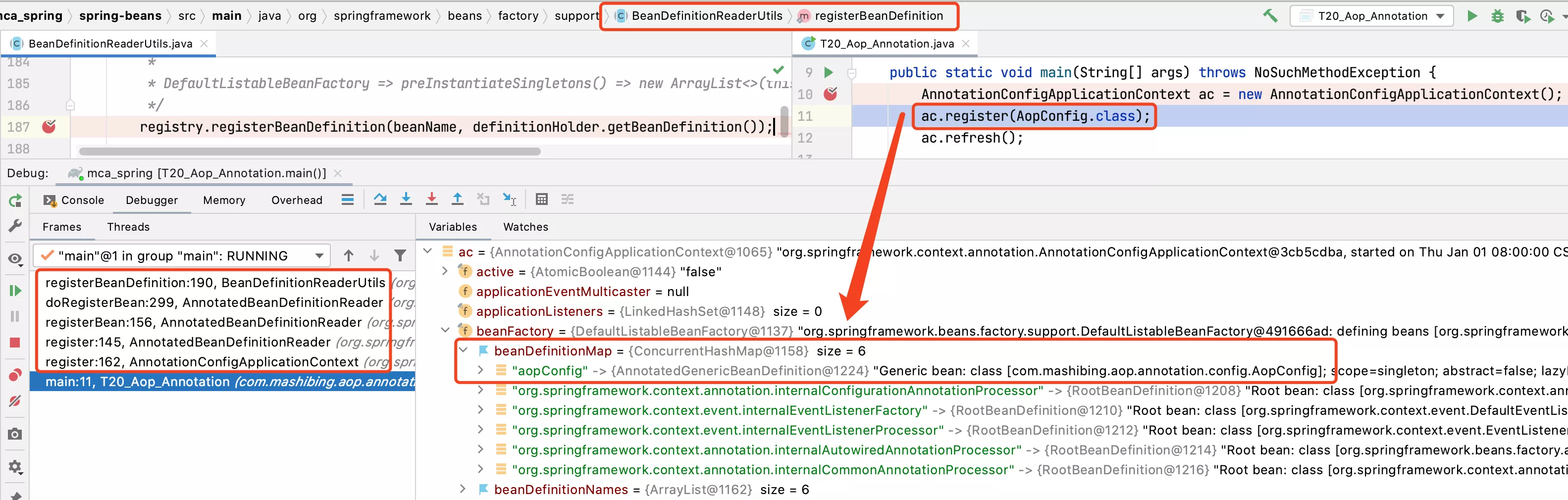
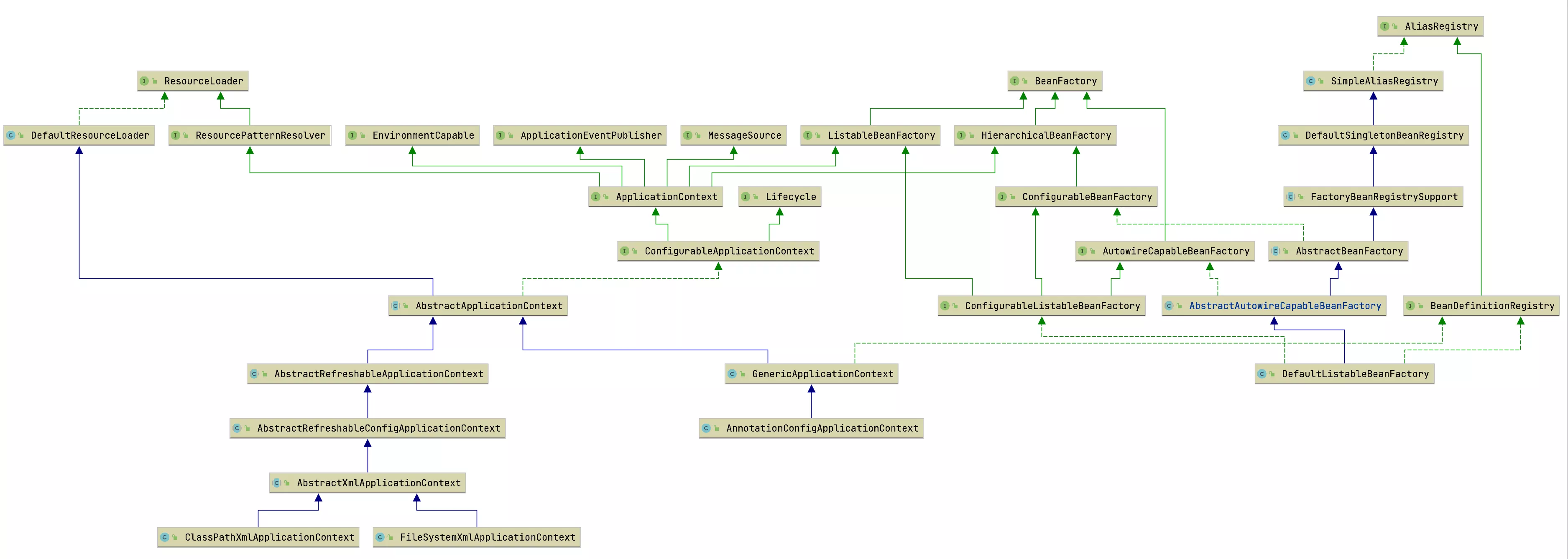
1. DefaultListableBF
public class GenericApplicationContext extends AbstractApplicationContext implements BeanDefinitionRegistry {
private final DefaultListableBeanFactory beanFactory;
// IOC容器创建: DefaultListableBeanFactory
public GenericApplicationContext() {
this.beanFactory = new DefaultListableBeanFactory();
}
}
2. AnnotatedBeanDefinitionReader
public class AnnotatedBeanDefinitionReader {
public AnnotatedBeanDefinitionReader(BeanDefinitionRegistry registry) {
this(registry, getOrCreateEnvironment(registry));
}
public AnnotatedBeanDefinitionReader(BeanDefinitionRegistry registry, Environment environment) {
this.registry = registry;
this.conditionEvaluator = new ConditionEvaluator(registry, environment, null); // @Conditional
// 1. AnnotationConfigUtils => internal类
AnnotationConfigUtils.registerAnnotationConfigProcessors(this.registry);
}
}
1. AnnotationConfigUtils
// AnnotationConfigUtils注入的internal类
1. internalConfigurationAnnotationProcessor => ConfigurationClassPostProcessor
2. internalAutowiredAnnotationProcessor => AutowiredAnnotationBeanPostProcessor
3. internalCommonAnnotationProcessor => CommonAnnotationBeanPostProcessor
4. internalEventListenerProcessor => EventListenerMethodProcessor
5. internalEventListenerFactory => DefaultEventListenerFactory
// AopConfigUtils注入的internal类
6. internalAutoProxyCreator => AspectJAwareAdvisorAutoProxyCreator
public abstract class AnnotationConfigUtils {
public static final String CONFIGURATION_ANNOTATION_PROCESSOR_BEAN_NAME = // 1.
"org.springframework.context.annotation.internalConfigurationAnnotationProcessor";
public static final String CONFIGURATION_BEAN_NAME_GENERATOR =
"org.springframework.context.annotation.internalConfigurationBeanNameGenerator";
public static final String AUTOWIRED_ANNOTATION_PROCESSOR_BEAN_NAME = // 2.
"org.springframework.context.annotation.internalAutowiredAnnotationProcessor";
@Deprecated
public static final String REQUIRED_ANNOTATION_PROCESSOR_BEAN_NAME =
"org.springframework.context.annotation.internalRequiredAnnotationProcessor";
public static final String COMMON_ANNOTATION_PROCESSOR_BEAN_NAME = // 3.
"org.springframework.context.annotation.internalCommonAnnotationProcessor";
public static final String PERSISTENCE_ANNOTATION_PROCESSOR_BEAN_NAME =
"org.springframework.context.annotation.internalPersistenceAnnotationProcessor";
private static final String PERSISTENCE_ANNOTATION_PROCESSOR_CLASS_NAME = // 4.
"org.springframework.orm.jpa.support.PersistenceAnnotationBeanPostProcessor";
public static final String EVENT_LISTENER_PROCESSOR_BEAN_NAME = // 5.
"org.springframework.context.event.internalEventListenerProcessor";
public static final String EVENT_LISTENER_FACTORY_BEAN_NAME = // 6.
"org.springframework.context.event.internalEventListenerFactory";
public static void registerAnnotationConfigProcessors(BeanDefinitionRegistry registry) {
registerAnnotationConfigProcessors(registry, null);
}
public static Set<BeanDefinitionHolder> registerAnnotationConfigProcessors(
BeanDefinitionRegistry registry, @Nullable Object source) {
// 获取BF
DefaultListableBeanFactory beanFactory = unwrapDefaultListableBeanFactory(registry);
if (beanFactory != null) {
if (!(beanFactory.getDependencyComparator() instanceof AnnotationAwareOrderComparator)) {
// 设置依赖比较器
beanFactory.setDependencyComparator(AnnotationAwareOrderComparator.INSTANCE);
}
if (!(beanFactory.getAutowireCandidateResolver() instanceof ContextAnnotationAutowireCandidateResolver)) {
// 设置自动装配解析器
beanFactory.setAutowireCandidateResolver(new ContextAnnotationAutowireCandidateResolver());
}
}
Set<BeanDefinitionHolder> beanDefs = new LinkedHashSet<>(8);
/*
* 1. internalConfigurationAnnotationProcessor => ConfigurationClassPostProcessor
*/
if (!registry.containsBeanDefinition(CONFIGURATION_ANNOTATION_PROCESSOR_BEAN_NAME)) {
RootBeanDefinition def = new RootBeanDefinition(ConfigurationClassPostProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, CONFIGURATION_ANNOTATION_PROCESSOR_BEAN_NAME));
}
/*
* 2. internalAutowiredAnnotationProcessor => AutowiredAnnotationBeanPostProcessor
*/
if (!registry.containsBeanDefinition(AUTOWIRED_ANNOTATION_PROCESSOR_BEAN_NAME)) {
RootBeanDefinition def = new RootBeanDefinition(AutowiredAnnotationBeanPostProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, AUTOWIRED_ANNOTATION_PROCESSOR_BEAN_NAME));
}
/*
* Check for JSR-250 support, and if present add the CommonAnnotationBeanPostProcessor.
* 3. internalCommonAnnotationProcessor => CommonAnnotationBeanPostProcessor
*/
if (jsr250Present && !registry.containsBeanDefinition(COMMON_ANNOTATION_PROCESSOR_BEAN_NAME)) {
RootBeanDefinition def = new RootBeanDefinition(CommonAnnotationBeanPostProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, COMMON_ANNOTATION_PROCESSOR_BEAN_NAME));
}
/*
* Check for JPA support, and if present add the PersistenceAnnotationBeanPostProcessor.
* 4. PersistenceAnnotationBeanPostProcessor
*/
if (jpaPresent && !registry.containsBeanDefinition(PERSISTENCE_ANNOTATION_PROCESSOR_BEAN_NAME)) {
RootBeanDefinition def = new RootBeanDefinition();
try {
def.setBeanClass(ClassUtils.forName(PERSISTENCE_ANNOTATION_PROCESSOR_CLASS_NAME,
AnnotationConfigUtils.class.getClassLoader()));
} catch (ClassNotFoundException ex) {
throw new IllegalStateException(
"Cannot load optional framework class: " + PERSISTENCE_ANNOTATION_PROCESSOR_CLASS_NAME, ex);
}
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, PERSISTENCE_ANNOTATION_PROCESSOR_BEAN_NAME));
}
// 5. @EventListener => EventListenerMethodProcessor
if (!registry.containsBeanDefinition(EVENT_LISTENER_PROCESSOR_BEAN_NAME)) {
RootBeanDefinition def = new RootBeanDefinition(EventListenerMethodProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, EVENT_LISTENER_PROCESSOR_BEAN_NAME));
}
// 6. DefaultEventListenerFactory
if (!registry.containsBeanDefinition(EVENT_LISTENER_FACTORY_BEAN_NAME)) {
RootBeanDefinition def = new RootBeanDefinition(DefaultEventListenerFactory.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, EVENT_LISTENER_FACTORY_BEAN_NAME));
}
return beanDefs;
}
}
2. refresh()
1. AnnotationConfigAC
- 不需要创建BF
- 不需要加载xml
refresh() => obtainFreshBeanFactory() => refreshBeanFactory()
/**
* 1. AbstractApplicationContext.refresh();
* 2. AbstractApplicationContext.obtainFreshBeanFactory();
* 3. GenericApplicationContext.refreshBeanFactory();
*/
public class GenericApplicationContext extends AbstractApplicationContext implements BeanDefinitionRegistry {
@Override
protected final void refreshBeanFactory() throws IllegalStateException {
if (!this.refreshed.compareAndSet(false, true)) {
throw new IllegalStateException(
"GenericApplicationContext does not support multiple refresh attempts: just call 'refresh' once");
}
this.beanFactory.setSerializationId(getId());
}
}
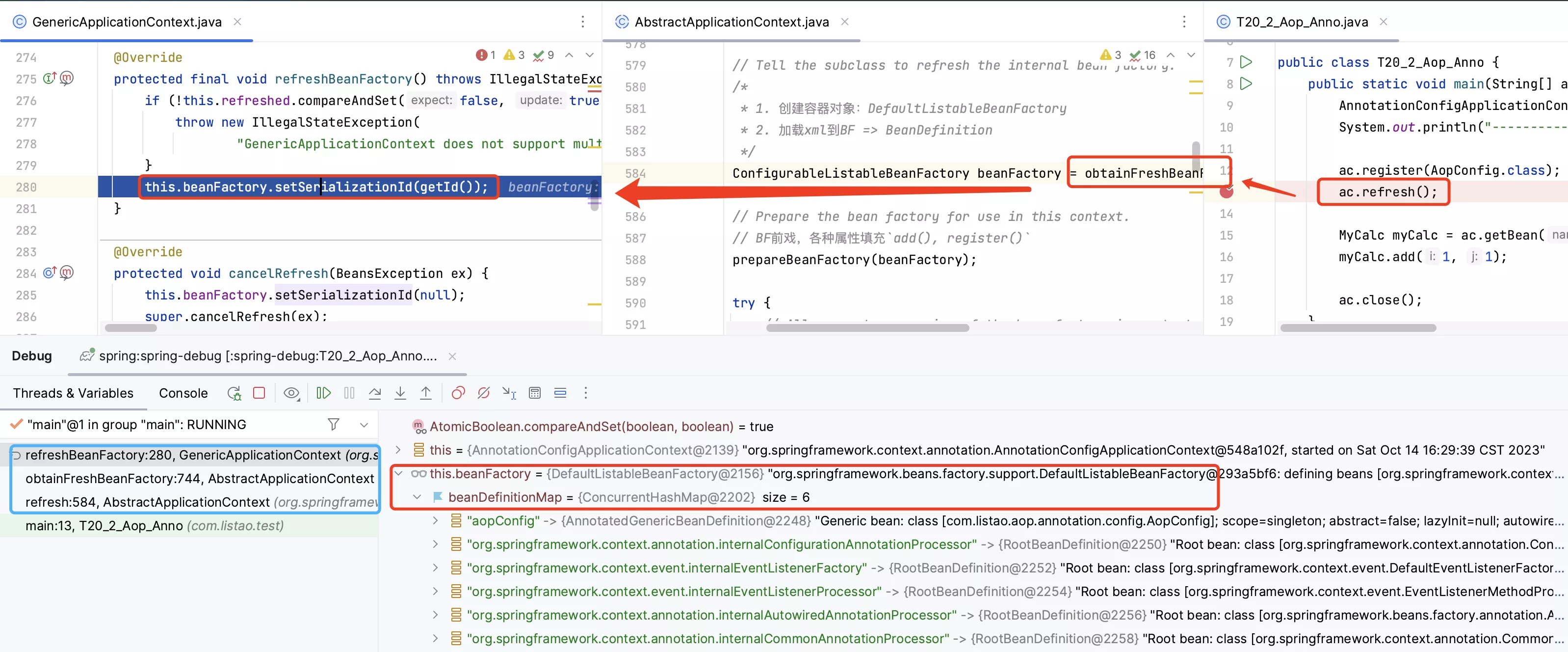
2. ClassPathXmlAC
/**
* 1. AbstractApplicationContext.refresh();
* 2. AbstractApplicationContext.obtainFreshBeanFactory();
* 3. AbstractRefreshableApplicationContext.refreshBeanFactory();
*/
public abstract class AbstractRefreshableApplicationContext extends AbstractApplicationContext {
@Override
protected final void refreshBeanFactory() throws BeansException {
// 如果存在BF,销毁BF
if (hasBeanFactory()) {
destroyBeans();
closeBeanFactory();
}
try {
/*
* 1. AbstractAutowireCapableBeanFactory => `allowCircularReferences = true;`
* ignoreDependencyInterface()忽略Aware接口的实现类进行IOC,由invokeAwareMethod()统一处理
* 2. DefaultListableBeanFactory => `allowBeanDefinitionOverriding = true;`
*/
DefaultListableBeanFactory beanFactory = createBeanFactory();
// 为序列化指定id,可以从id反序列化到BF对象
beanFactory.setSerializationId(getId());
/*
* 定制BF,设置相关属性
* 1. 是否允许覆盖BD`allowBeanDefinitionOverriding = true`
* 2. 是否允许循环依赖`allowCircularReferences = true`
*/
customizeBeanFactory(beanFactory);
/*
* 1. BF => 初始化BDR => 读取并解析xml
* 2. 命名空间解析,标签解析
*/
loadBeanDefinitions(beanFactory);
// debug,关注`beanDefinitionMap, beanDefinitionNames`
this.beanFactory = beanFactory;
} catch (IOException ex) {
throw new ApplicationContextException("I/O error parsing bean definition source for " + getDisplayName(), ex);
}
}
}
3. invokeBFPP()
ConfigurationClassPostProcessor
处理BD_map中ConfigClass类- 1. @EnableAspectJAutoProxy
@Configuration
@ComponentScan(basePackages = "com.listao.aop.annotation")
@EnableAspectJAutoProxy
public class AopConfig {
}
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Import(AspectJAutoProxyRegistrar.class)
public @interface EnableAspectJAutoProxy {}
7. advice排序问题
@Order
注解无用,不能影响拓扑排序顺序- xml顺序影响Topo-sort的无关结果
// -------- xml
around() -> before, add(), 参数为: [1, 1]
before() -> add(), 参数为: [1, 1]
====>>>> MyCalc.add()
around() -> after, add()结果: 2
after() -> add(), 结束
afterReturning() -> add(), 结果为: 2
// -------- anno
around() -> before, add(), 参数为: [1, 1]
before() -> add(), 参数为: [1, 1]
====>>>> MyCalc.add()
afterReturning() -> add(), 结果为: 2
after() -> add(), 结束
around() -> after, add()结果: 2
1. xml顺序
ConfigBeanDefinitionParser#createAdviceDefinition()
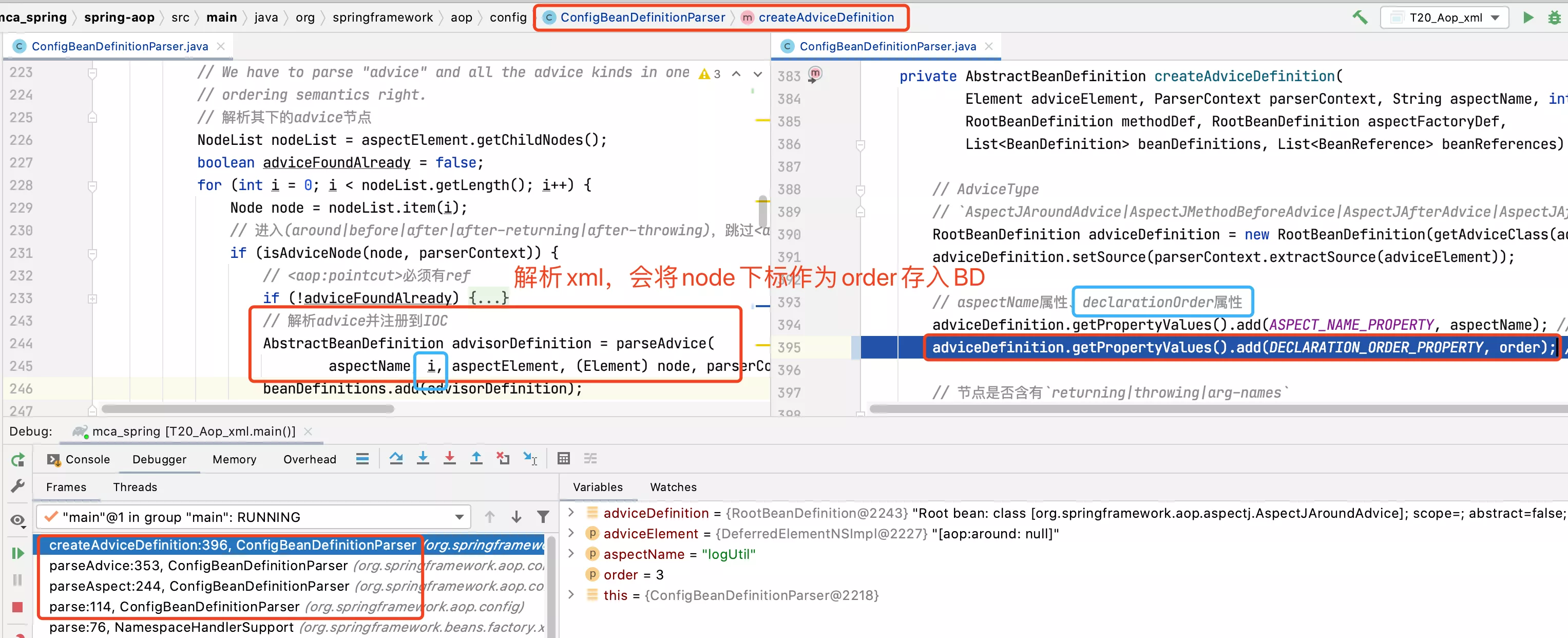
AspectJAwareAdvisorAutoProxyCreator#sortAdvisors()
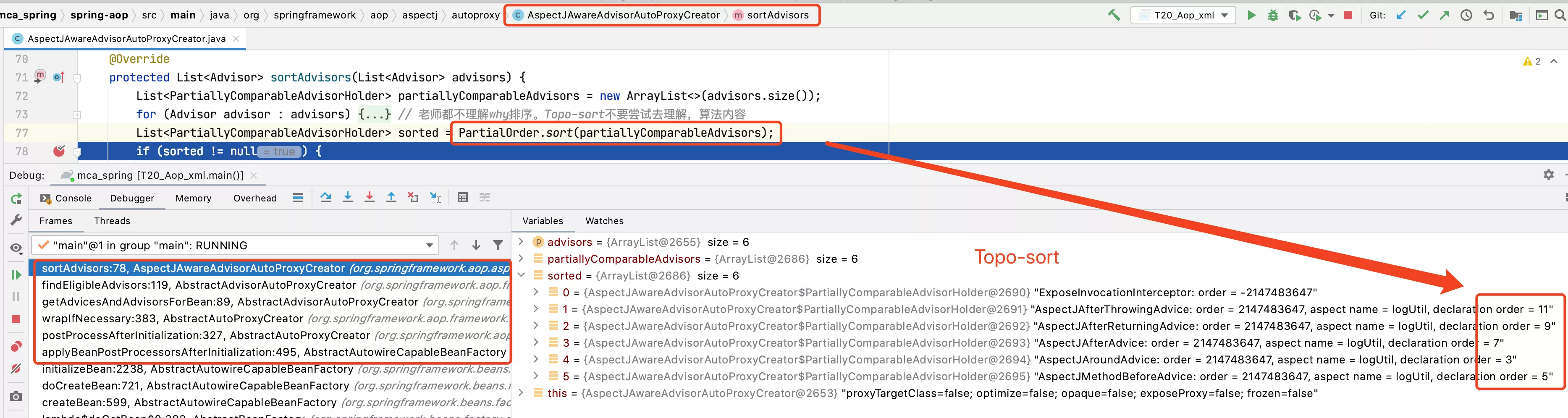
<aop:around method="around" pointcut-ref="myPoint"/>
<aop:before method="before" pointcut-ref="myPoint"/>
around() -> before, add(), 参数为: [1, 1]
before() -> add(), 参数为: [1, 1]
====>>>> MyCalculator.add()
around() -> after, add()结果: 2
after() -> add(), 结束
afterReturning() -> add(), 结果为: 2
<aop:before method="before" pointcut-ref="myPoint"/>
<aop:around method="around" pointcut-ref="myPoint"/>
before() -> add(), 参数为: [1, 1]
around() -> before, add(), 参数为: [1, 1]
====>>>> MyCalculator.add()
around() -> after, add()结果: 2
after() -> add(), 结束
afterReturning() -> add(), 结果为: 2