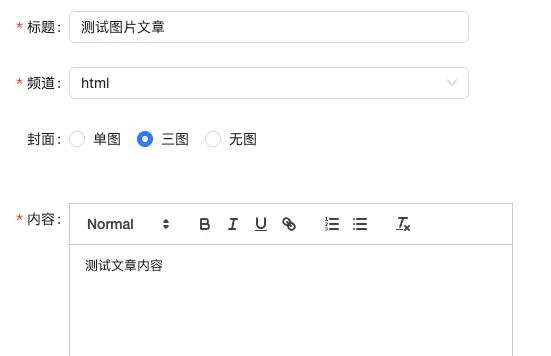
const Publish = ()=>{
const [searchParams] = useSearchParams()
const articleId = searchParams.get('id')
const [form] = Form.useForm()
useEffect(() => {
async function getArticle () {
const res = await http.get(`/mp/articles/${articleId}`)
const { cover, ...formValue } = res.data
form.setFieldsValue({ ...formValue, type: cover.type })
}
if (articleId) {
getArticle()
}
}, [articleId, form])
return (
<Form form={form}/>
)
}
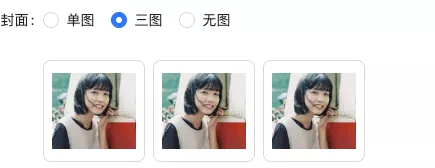
useEffect(() => {
async function getArticle () {
const res = await http.get(`/mp/articles/${articleId}`)
const { cover, ...formValue } = res.data
form.setFieldsValue({ ...formValue, type: cover.type })
setImageType(cover.type)
setImageList(cover.images.map(url => ({ url })))
}
if (articleId) {
getArticle()
}
}, [articleId, form])
<Card
title={
<Breadcrumb items={[
{ title: <Link to={'/'}>首页</Link> },
{ title: `${articleId ? '编辑文章' : '发布文章'}` },
]}
/>
}
>
{articleId ? '更新文章' : '发布文章'}
const onFinish = async (formValue) => {
const { channel_id, content, title } = formValue
const formatUrl = (list) => {
return list.map(item => {
if (item.response) {
return item.response.data.url
} else {
return item.url
}
})
}
const data = {
channel_id,
content,
title,
type: imageType,
cover: {
type: imageType,
images: formatUrl(imageList)
}
}
if (imageType !== imageList.length) return message.warning('图片类型和数量不一致')
if (articleId) {
await http.put(`/mp/articles/${articleId}?draft=false`, data)
} else {
await http.post('/mp/articles?draft=false', data)
}
message.success(`${articleId ? '编辑' : '发布'}文章成功`)
}