02-Annotation
1. @Deprecated
1. java9
- 使用它存在风险,可能导致错误
- 可能在未来版本中不兼容
- 可能在未来版本中删除
- 一个更好和更高效的方案已经取代它
2. @RequestHeader
1. 获取指定的请求头
@PostMapping("/ooxx")
ResultUtil<Boolean> ooxx(@RequestHeader("X-Ticket") String ticket, @RequestBody @Validated Ooxx ooxx);
2. 获取全部请求头
/**
* 接收省内请求省间同步接口,在省间同步省内数据
*
* @param paramList 字符串集合
* @return
*/
@PostMapping("/headers")
public ResultUtil headers(@RequestHeader Map<String, String> headers, @RequestBody String paramList) {
log.info("获取请求头参数User-Agent:{}", headers.get("user-agent"));
return null;
}
3. httpServletRequest
private void infoRequestHeaders() {
Enumeration<String> headerNames = httpServletRequest.getHeaderNames();
while (headerNames.hasMoreElements()) {
String headerName = headerNames.nextElement();
log.info("headerName: {}, => value: {}", headerName, httpServletRequest.getHeader(headerName));
}
}
3. @Value
1. 默认值
// String类型不可以有空格
@Value("${autoConfig:autoConfig}")
private String autoConfig;
// boolean类型可以有空格
@Value("${isDel: false}")
private boolean isDel;
4. @SuppressWarnings
Java魔法堂:注解用法详解——@SuppressWarnings
1. unchecked
@SuppressWarnings("unchecked")
- 抑制强转的警告
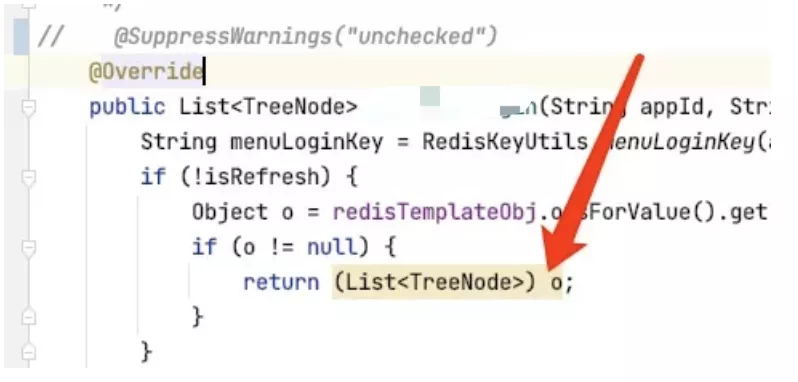
2. rawtypes
@SuppressWarnings("rawtypes")
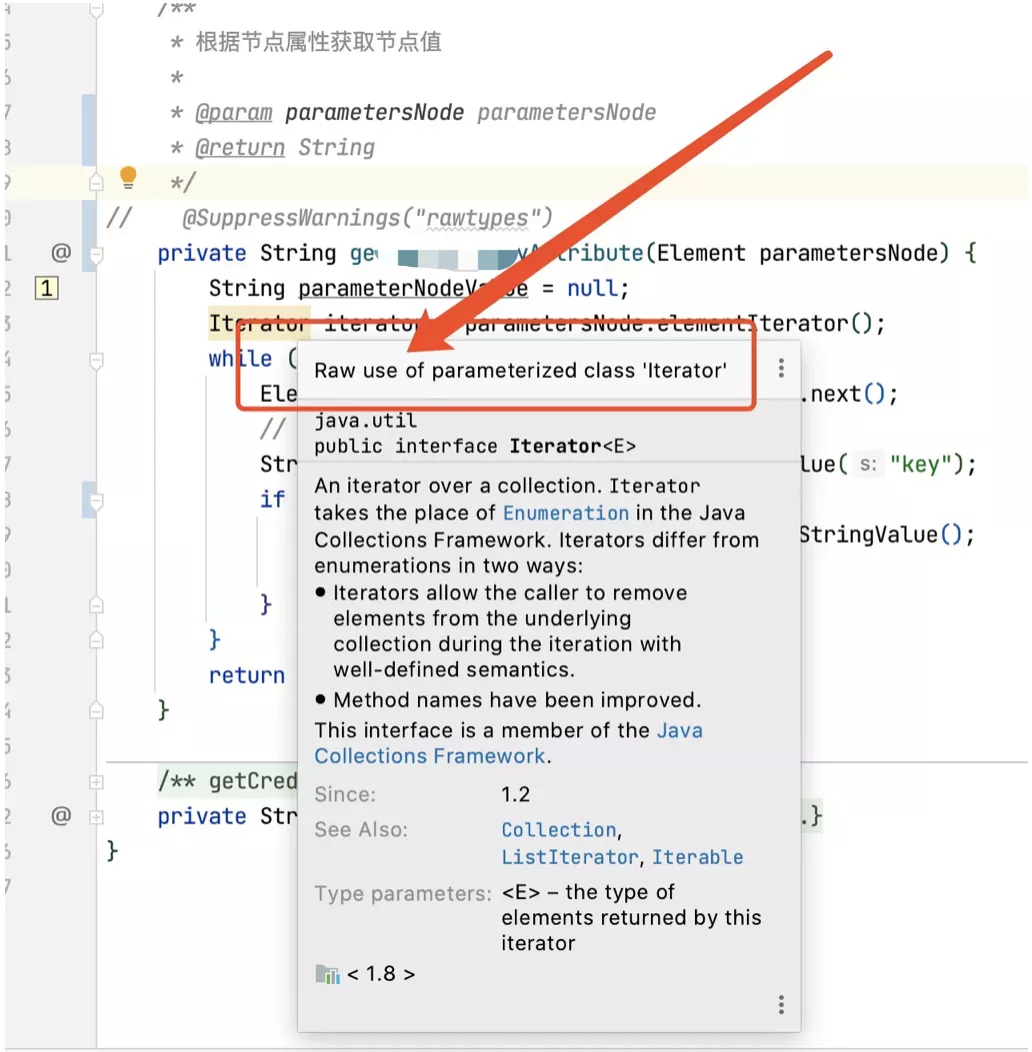
5. @Validated
1. String
@NotBlank
非空校验(不为null,也不为“”)@Length
长度校验@Pattern
正则校验
只有 @Length 没有 @NotBlank 是不可以的。
{"loginName": null}
{}
两种情况都会被校验通过
@NotBlank(message = "name为空")
@Length(min = 5, max = 20, message = "名称长度不小于5位,不大于20位")
@Pattern(regexp = "^((?=.*[a-zA-Z])(?=.*[0-9]))[A-Za-z0-9]*$", message = "名称格式不正确")
private String name;
2. byte
1. @Min
- 大于等于
- 《byte》 value != null && value >= min
- 《Byte》 value >= min
- 无默认值传null
- 《byte》 value == 0
- 《Byte》 value == null
/**
* type >= 2 && type != null
*/
@Min(value = 2, message = "类型有误")
private byte type = 11;
@NotNull
@Min(value = 2, message = "类型有误")
private Byte type = 11;
6. @Positive
- 正整数
/**
* 类型:1-ukey, 2-密码
*/
@Positive(message = "类型有误")
private Byte type = 1;
1. @PositiveOrZero
7. @WebServlet
@WebServlet(name = "AddServlet", urlPatterns = "/add")
8. @Async
9. @ConfigurationProperties
1. 直接返回注入对象
@Resource
private OoxxConfig ooxxConfig;
@RequestMapping("/ooxxConfig")
public OoxxConfig ooxxConfig() {
return ooxxConfig;
}
{
"appKey": "app200817260019687291",
"secret": "6c81c568b03288341411f581546e52c69e977d137cbdbac05834739ed12adee6___",
"host": "http://ip:port",
"urls": {
"logout": "/biz/company/logout",
"freeze": "/biz/account/freeze",
"list": "/biz/company/account/list",
// ...
},
"targetSource": {
"targetBeanName": "scopedTarget.ooxxConfig",
"targetClass": "com.listao.config.OoxxConfig",
"beanFactory": {
"parentBeanFactory": {
"parentBeanFactory": null,
"beanClassLoader": {
"parent": {
"parent": null,
"urls": [
"file:/Library/Java/JavaVirtualMachines/jdk1.8.0_212.jdk/Contents/Home/jre/lib/ext/sunec.jar",
"file:/Library/Java/JavaVirtualMachines/jdk1.8.0_212.jdk/Contents/Home/jre/lib/ext/nashorn.jar",
// ...
]
},
"urls": [
"file:/Library/Java/JavaVirtualMachines/jdk1.8.0_212.jdk/Contents/Home/jre/lib/charsets.jar",
"file:/Library/Java/JavaVirtualMachines/jdk1.8.0_212.jdk/Contents/Home/jre/lib/deploy.jar",
// ...
{
"timestamp": "2021-04-08T07:55:36.146+0000",
"status": 200,
"error": "OK",
"message": "Type definition error: [simple type, class org.springframework.context.expression.StandardBeanExpressionResolver]; nested exception is com.fasterxml.jackson.databind.exc.InvalidDefinitionException: No serializer found for class org.springframework.context.expression.StandardBeanExpressionResolver and no properties discovered to create BeanSerializer (to avoid exception, disable SerializationFeature.FAIL_ON_EMPTY_BEANS) (through reference chain: com.listao.config.OoxxConfig$$EnhancerBySpringCGLIB$$77b1a5bd[\"targetSource\"]->org.springframework.aop.target.SimpleBeanTargetSource[\"beanFactory\"]->org.springframework.beans.factory.support.DefaultListableBeanFactory[\"parentBeanFactory\"]->org.springframework.beans.factory.support.DefaultListableBeanFactory[\"beanExpressionResolver\"])",
"path": "/ooxx/ooxxConfig"
},
@RequestMapping("/ooxxConfig")
public OoxxConfig ooxxConfig() {
OoxxConfig clone = new OoxxConfig();
BeanUtil.copyProperties(ooxxConfig, clone, false);
return clone;
}
2. debug
- debug模式下,显示的注册的属性都为
null
,可是已经注入了
10. @Transactional
- Spring 异常事务回滚@Transactional 注解的使用
- 作用在
Controller层
。本次请求有异常,本次请求的所有数据库操作回滚
11. @RefreshScope
12. @Pointcut
..
:多级包路径匹配*Ctl
:匹配类名后缀为Ctl的类
@Pointcut("execution(public * com.listao.controller..*Ctl.*(..))")
public void log() {
}