- http://localhost:8080/swagger-ui.html
- https://editor.swagger.io/
- https://editor-next.swagger.io/
- Swagger是RESTful接口文档的规范和工具集,统一RESTful接口文档的格式和规范
- 方便开发者查看、理解接口的功能和参数,还能帮助前后端开发协同工作,提高开发效率
- SpringBoot中,通过集成Swagger实现接口文档的自动生成。Swagger通过注解来描述接口,根据注解自动生成接口文档
- ApiDoc定位:
ApiDoc = Postman + Swagger + mock + Jmeter
,是一款集API设计,接口文档管理、代码生成、API调试、API mock、API自动化为一体的接口一站式协作平台 - 比Swagger功能要更加广泛、齐全,不仅通过可视化界面设计接口生成接口文档和项目代码,还打通了接口数据的协作流程,一套接口数据,设计出来可以给前端、测试使用,减少了再不同系统间切换、导入导出数据、更新维护的麻烦
- 缺点:太繁琐,因为支持了太多的功能,并且也不符合注解式开发的规范,需要写大量的文档类东西
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
- 包括扫描的包路径、接口文档信息、全局参数、安全协议、安全上下文等
@Configuration
@EnableSwagger2
public class SwaggerConfig {
private static final Set<String> DEFAULT_PRODUCES_CONSUMES =
new HashSet<>(Arrays.asList("application/json", "application/xml", "application/x-www-form-urlencoded"));
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.listao.spring_boot.swagger"))
.paths(PathSelectors.any())
.build()
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("Swagger测试_接口文档")
.description("Swagger测试")
.version("1.0.0")
.build();
}
}
注解 | 含义 |
---|
@Api | 描述接口的类或接口 |
@ApiOperation | 描述接口的方法 |
@ApiParam | 描述接口的参数 |
@ApiModel | 描述数据模型 |
@ApiModelProperty | 描述数据模型的属性 |
@ApiImplicitParams | 方法参数的说明 |
@ApiImplicitParam | 指定单个参数的说明 |
@ApiResponses | 方法返回值的说明 |
@ApiResponse | 用于指定单个参数的说明 |
注解属性 | 含义 |
---|
value | url的路径值 |
tags | 如果设置这个值、value的值会被覆盖 |
description | 对api资源的描述 |
basePath | 基本路径 |
position | 如果配置多个Api 想改变显示的顺序位置 |
produces | application/json, application/xml |
consumes | application/json, application/xml |
protocols | http, https, ws, wss |
authorizations | 高级特性认证时配置 |
hidden | 配置为true,将在文档中隐藏 |
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
@ApiModel(description = "Swagger_req")
public class Swagger_req {
@ApiModelProperty(value = "入参id", example = "mock_入参id")
private String reqId;
@ApiModelProperty(value = "入参name", example = "mock_入参name")
private String reqName;
@ApiModelProperty(value = "入参date")
private Date date;
@ApiModelProperty(hidden = true)
public String pwd;
}
@ApiModel(description = "性别")
public enum Swagger_enum {
@ApiModelProperty(value = "男")
MALE,
@ApiModelProperty(value = "女")
FEMALE;
}
@ApiResponse
@ApiResponses
@ApiResponse(code = 200, message = "请求成功", response = Swagger_resp.class)
@ApiResponses({
@ApiResponse(code = 200, message = "请求成功", response = Swagger_resp.class),
@ApiResponse(code = 404, message = "id不存在", response = Swagger_resp.class)
})
@Controller
@Api(value = "Swagger控制器", tags = "Swagger控制器")
public class SwaggerCtl {
@ResponseBody
@PostMapping(value = "/swagger_param", consumes = MediaType.APPLICATION_FORM_URLENCODED_VALUE)
@ApiOperation(value = "form类型接口")
@ApiResponse(code = 200, message = "请求成功", response = Swagger_resp.class)
public Result<Swagger_resp> swagger_param(
@ApiParam(value = "入参param1", example = "1324") @RequestParam Long param1,
@ApiParam(value = "入参param2", example = "mock_param2") @RequestParam String param2,
@ApiParam(value = "入参日期", example = "2021/12/31 11:04:15") @RequestParam Date date,
Swagger_req swaggerReq, Swagger_req swaggerReq1) {
System.out.println("param1 = " + param1);
System.out.println("param2 = " + param2);
System.out.println("date = " + date);
System.out.println("swaggerReq = " + swaggerReq);
System.out.println("swaggerReq1 = " + swaggerReq1);
return Result.ok(new Swagger_resp(swaggerReq.getReqId(), param2));
}
@ResponseBody
@PostMapping("/swagger_obj")
@ApiOperation("json类型接口")
@ApiResponse(code = 200, message = "请求成功", response = Swagger_resp.class)
public Swagger_resp swagger_obj(@RequestBody Swagger_req swaggerReq) {
System.out.println("swaggerReq = " + swaggerReq);
return new Swagger_resp(swaggerReq.getReqId(), swaggerReq.getReqName());
}
}
globalOperationParameters()
配置全局参数。eg:配置一个全局Authorization
用于授权
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.listao.spring_boot.swagger"))
.paths(PathSelectors.any())
.build()
.globalOperationParameters(Arrays.asList(
new ParameterBuilder()
.name("Authorization")
.description("授权")
.modelRef(new ModelRef("string"))
.parameterType("header")
.required(false)
.build()
))
.apiInfo(apiInfo());
}
securitySchemes()
配置Bearer Token
安全协议
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.listao.spring_boot.swagger"))
.paths(PathSelectors.any())
.build()
.securitySchemes(Arrays.asList(
new ApiKey("Bearer", "Authorization", "header")
))
.apiInfo(apiInfo());
}
securityContexts()
方法来配置安全上下文。用于在Swagger UI
中显示认证按钮
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.listao.spring_boot.swagger"))
.paths(PathSelectors.any())
.build()
.securitySchemes(Arrays.asList(
new ApiKey("Bearer", "Authorization", "header")
))
.securityContexts(Collections.singletonList(
SecurityContext.builder()
.securityReferences(Collections.singletonList(
new SecurityReference("Bearer", new AuthorizationScope[0])
))
.build()
))
.apiInfo(apiInfo());
}
有些参数可能是敏感信息,不希望在接口文档中显示
@ApiIgnore
:类、方法、方法参数上进行忽略@ApiModelProperty(hidden = true)
:该参数是隐藏的
@ApiModelProperty(hidden = true)
public String pwd;
- https://editor.swagger.io/
- https://editor-next.swagger.io/
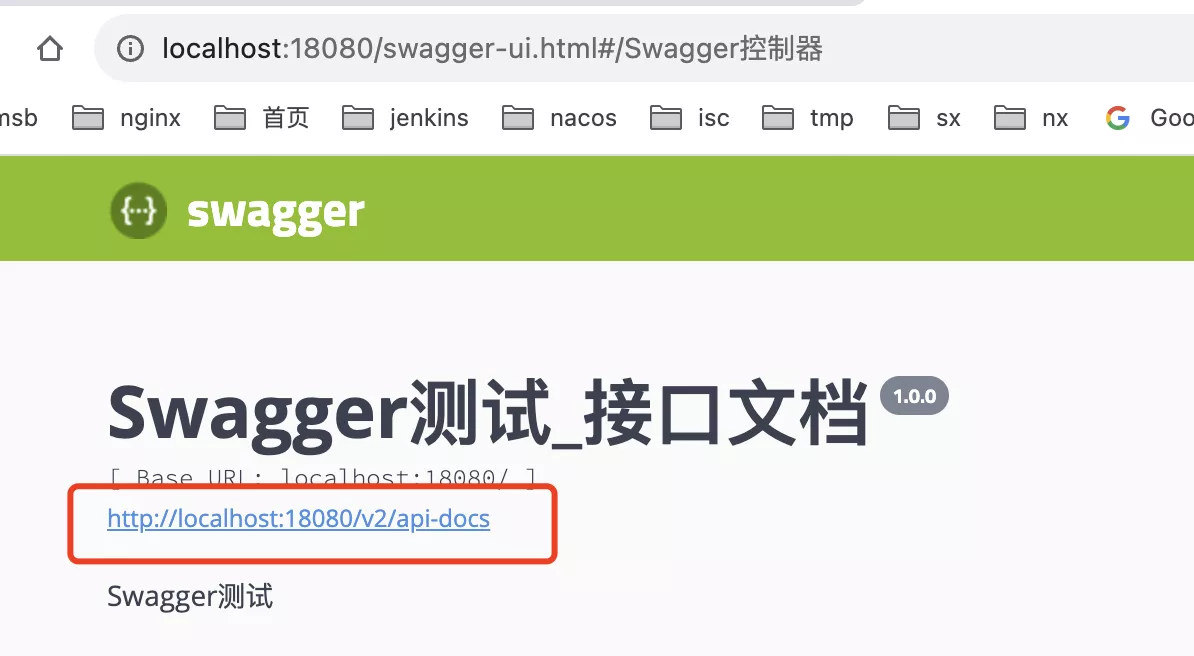
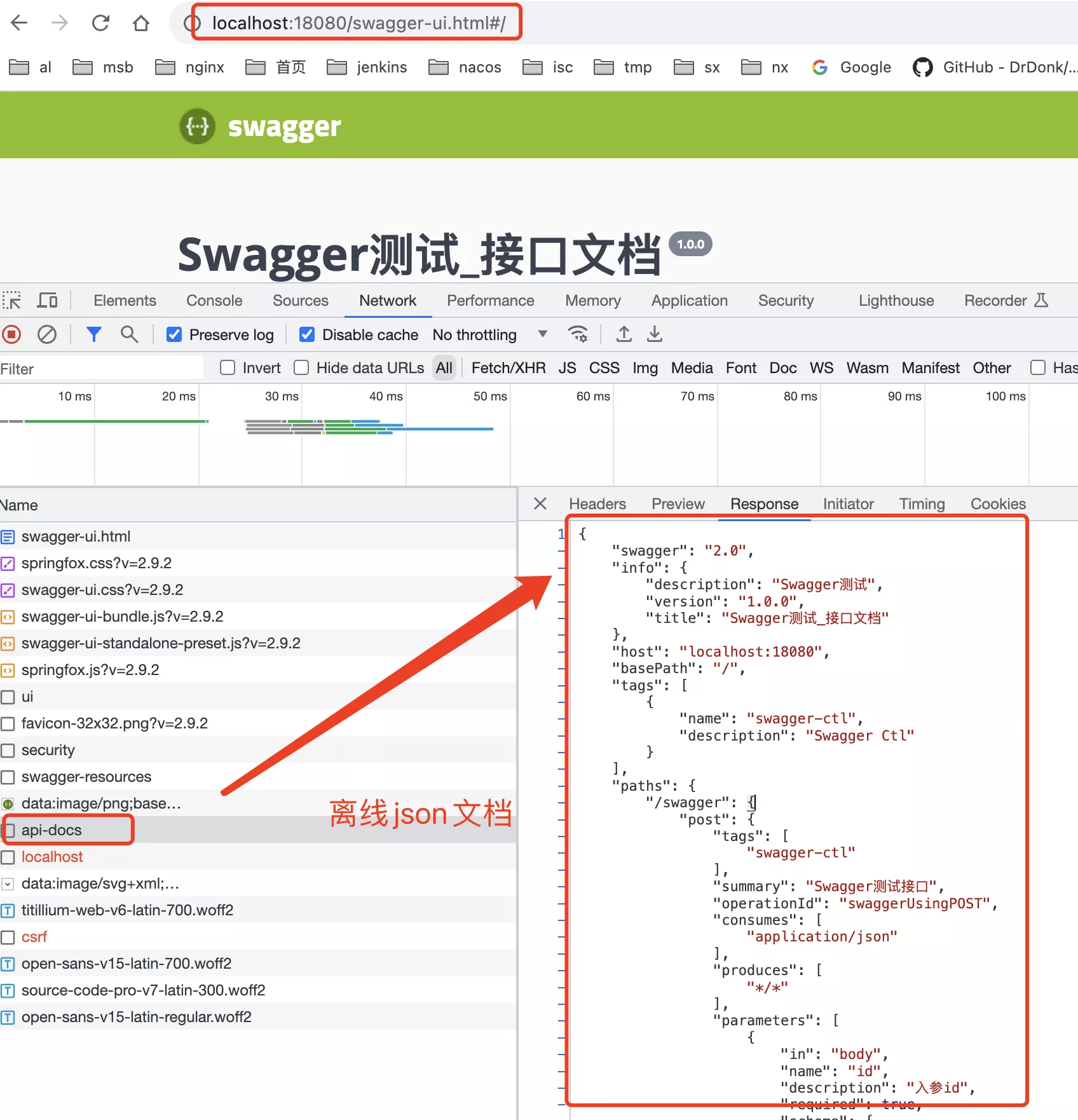
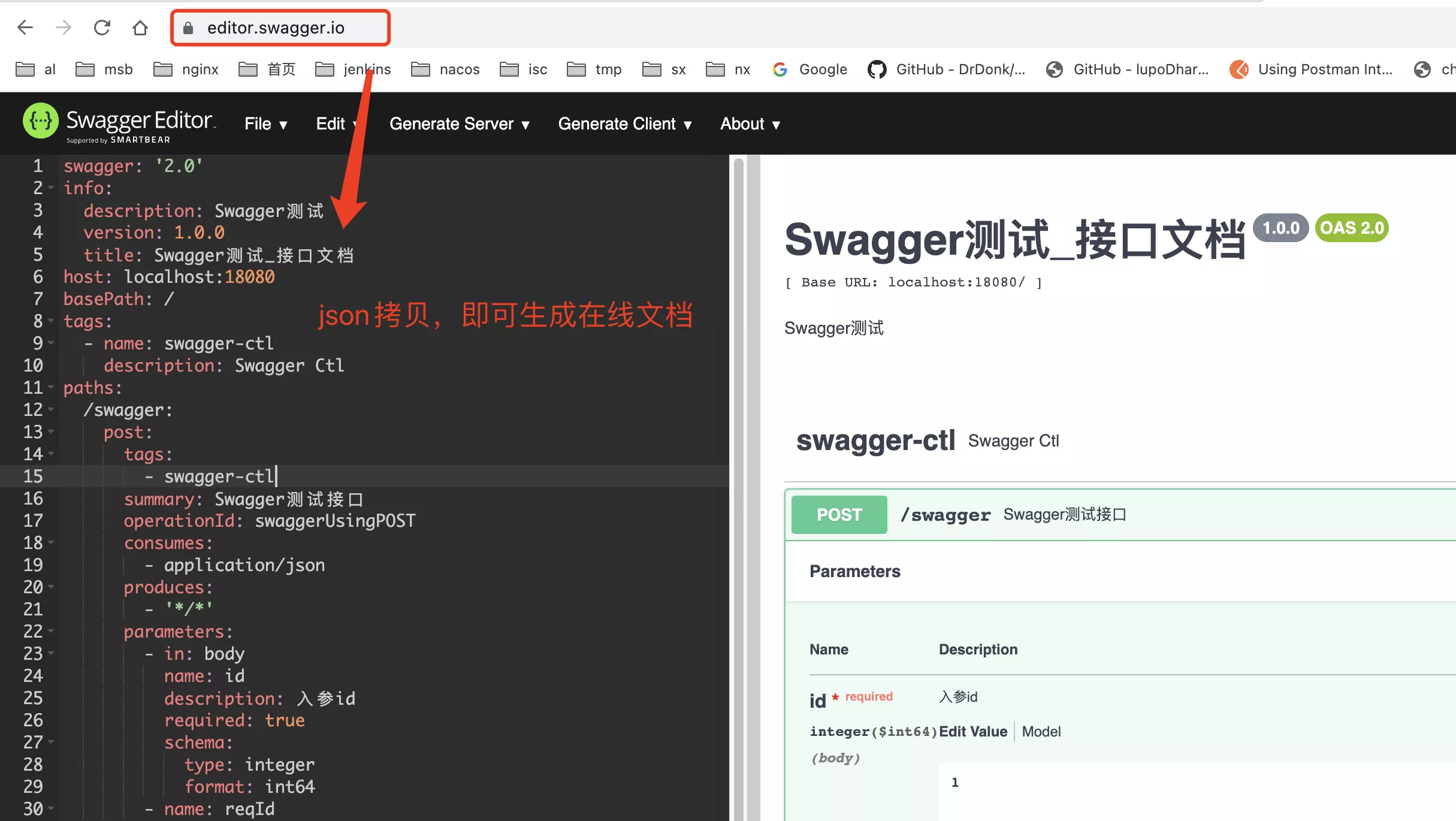
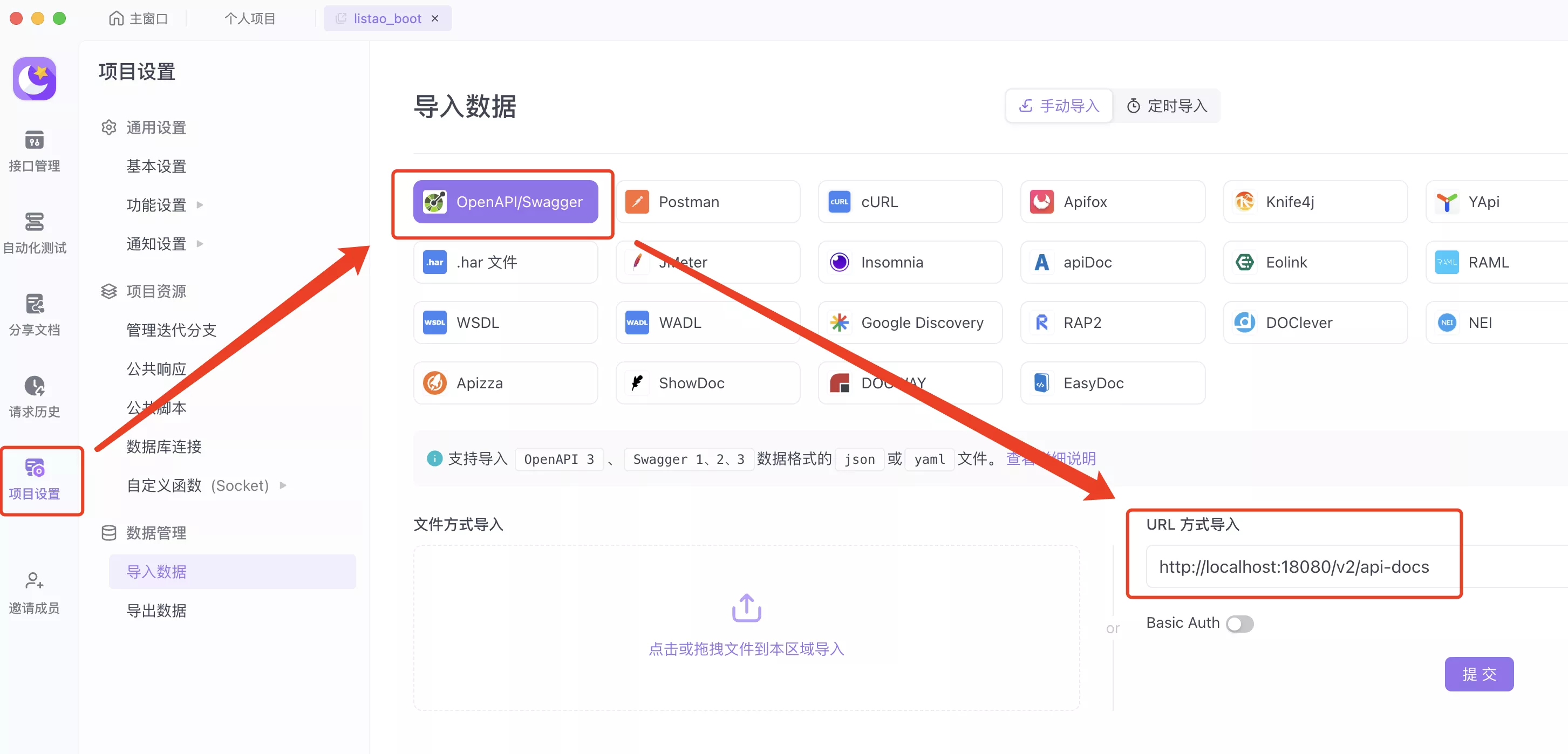
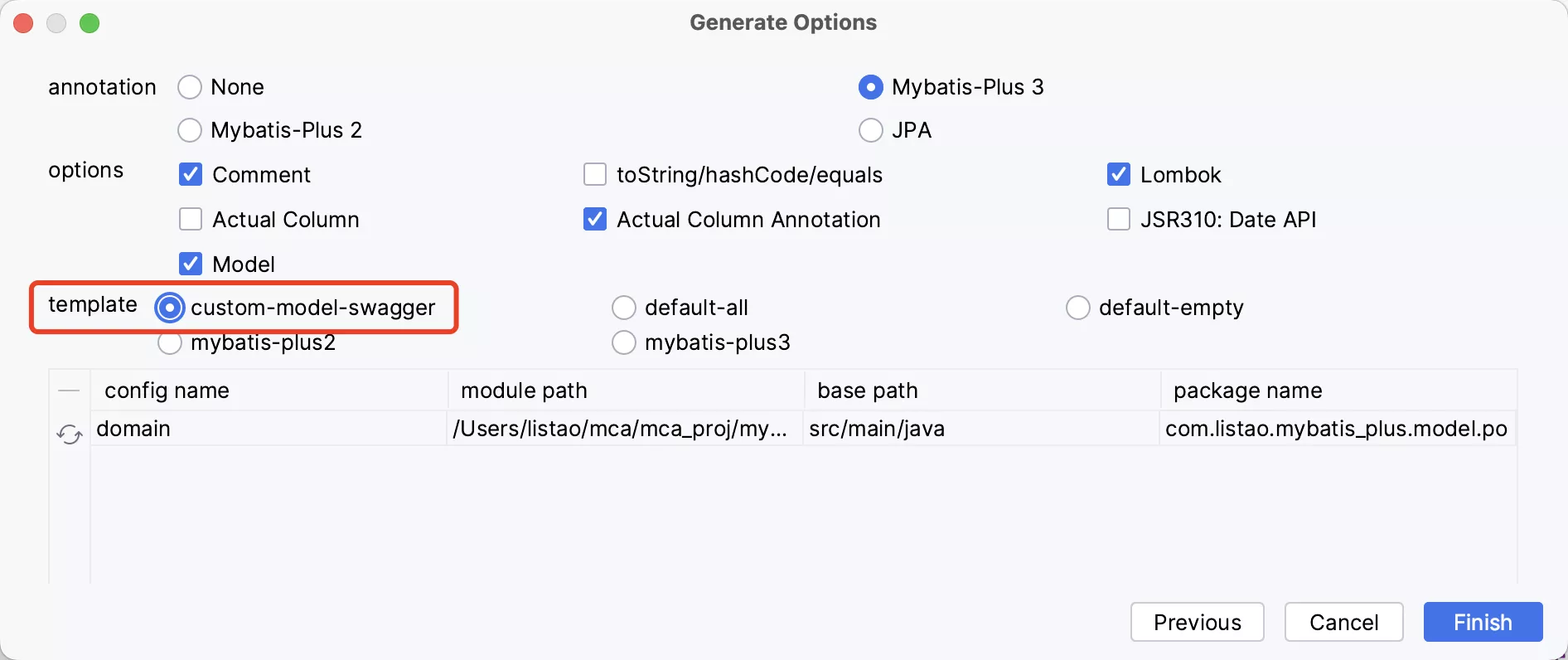
public class Ooxx implements Serializable {
@NotNull(message="[主键ID]不能为空")
@ApiModelProperty("主键ID")
private Long id;
@Size(max= 30,message="编码长度不能超过30")
@ApiModelProperty("姓名")
@Length(max= 30,message="编码长度不能超过30")
private String name;
@ApiModelProperty("年龄")
private Integer age;
@Size(max= 50,message="编码长度不能超过50")
@ApiModelProperty("邮箱")
@Length(max= 50,message="编码长度不能超过50")
private String email;
@ApiModelProperty("是否删除")
private Integer isDel;
}
- OpenAPI = Swagger3
- 官方样例代码导出,收集至《open_api官方逆向代码》
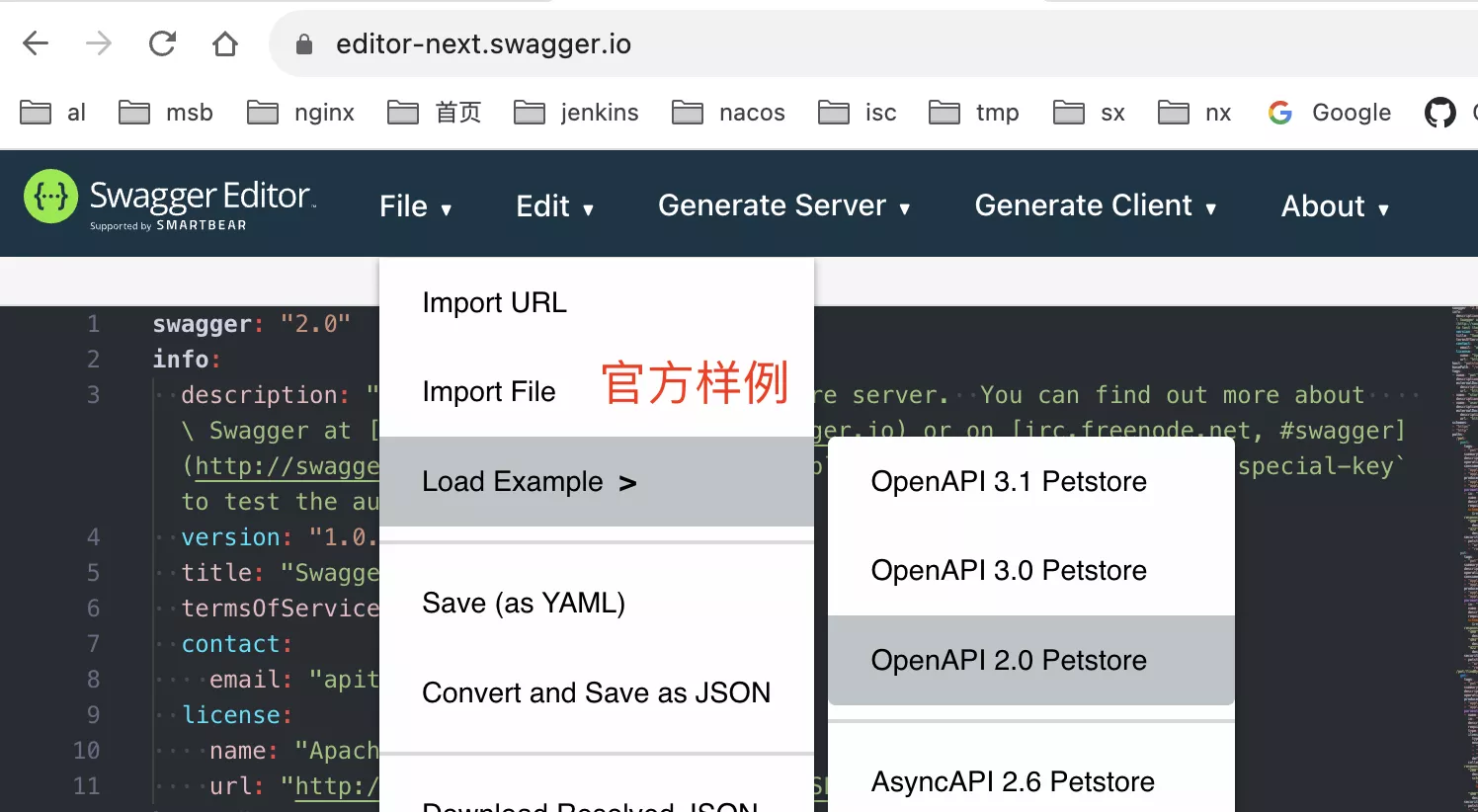
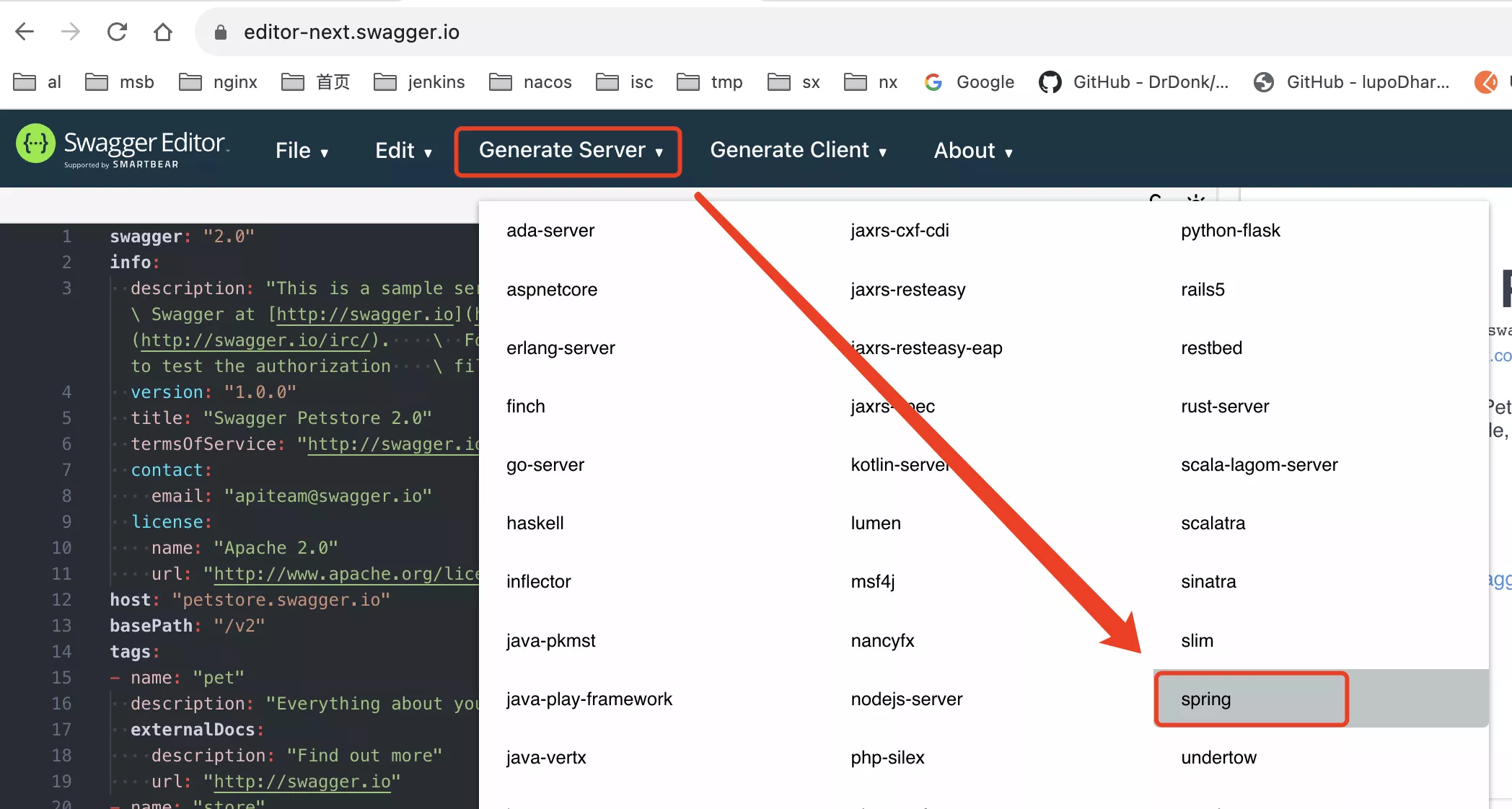